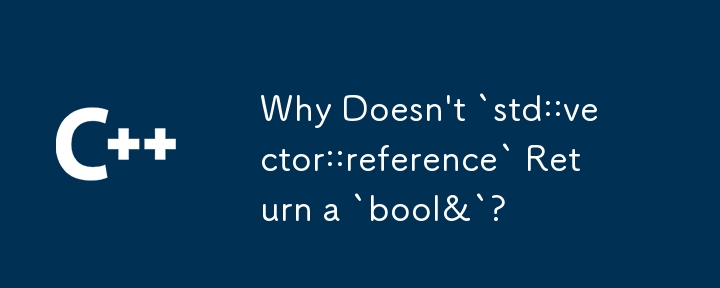
Why vector::reference Doesn't Return Reference to Bool?
In C , the std::vector container is specialized for boolean values. This specialization introduces differences in behavior compared to vectors of other data types.
The Issue
In the provided example, a function callIfToggled is used to toggle a bool value and call a method on a struct. When attempting to pass a reference to an element of a std::vector to callIfToggled, a compilation error occurs. This is because the reference type returned by vector::reference is not the expected bool&.
The Reason
Within the bool vector specialization, vector::reference returns a reference to a char, not a bool. This is done to optimize performance by using a bitwise representation for Boolean values.
Solutions
-
Use vector Instead: Replace the bool vector with a vector to work around the specialization issue.
-
Use Boost Containers Library: The Boost Containers library provides an unspecialized version of vector that offers a true reference to bool values.
-
Wrap Bool Values in a Structure: Create a wrapper struct around bool called MyBool. This allows passing a reference to the wrapper, which in turn provides indirect access to the underlying bool value.
Example (Vector of Char):
#include <vector>
struct A
{
void foo() {}
};
template<typename T>
void callIfToggled(char v1, char &v2, T &t)
{
if (v1 != v2)
{
v2 = v1;
t.foo();
}
}
int main()
{
std::vector<char> v = { false, true, false };
const char f = false;
A a;
callIfToggled(f, v[0], a);
callIfToggled(f, v[1], a);
callIfToggled(f, v[2], a);
}
Copy after login
The above is the detailed content of Why Doesn't `std::vector::reference` Return a `bool&`?. For more information, please follow other related articles on the PHP Chinese website!