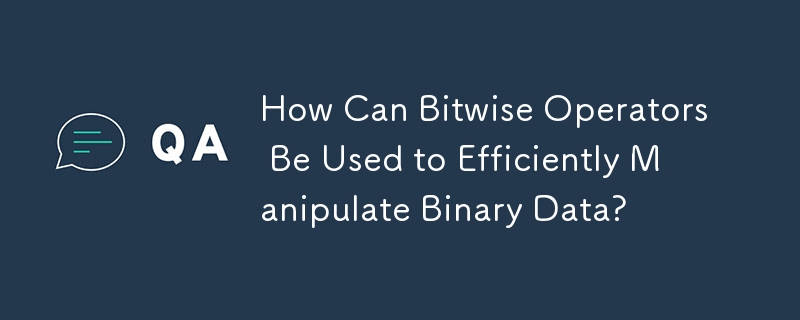
Bitwise Operators: Understanding Their Functionality and Practical Uses
Bitwise operators are a valuable tool for manipulating binary data, performing operations on multi-bit values at the individual bit level. Unlike arithmetic operators, they operate on each bit separately.
Bitwise Operators in Action
In Python, the commonly used bitwise operators are:
-
AND (&): Returns 1 only if both operands have a bit value of 1.
-
OR (|): Returns 1 if either or both operands have a bit value of 1.
-
XOR (^): Returns 1 only if exactly one of the operands has a bit value of 1.
-
NOT (~): Inverts the binary representation (0 -> 1, 1 -> 0).
-
Bit Shift (>>/<<): Shifts the bits to the right/left by a specified number of positions.
Example:
Consider the following Python code:
x = 1 # 0001
x << 2 # Shift left 2 bits: 0100
# Result: 4
x | 2 # Bitwise OR: 0011
# Result: 3
x & 1 # Bitwise AND: 0001
# Result: 1
Copy after login
- In the first line, the left shift operator (<<) multiplies the value (1) by 2 to the power of 2 (0100).
- In the second line, the OR operator (|) combines the value (1) with the decimal number 2 to give 3.
- In the third line, the AND operator (&) compares each bit and returns 1 only when both bits are 1.
Practical Applications of Bitwise Operators
Bitwise operators have diverse applications in programming:
-
Set or Clear Specific Bits: By using AND or OR with a mask value, you can set or clear individual bits.
-
Retrieve Individual Bits: By ANDing a value with a mask, you can isolate specific bits.
-
Bit Field Manipulation: In data structures, bit fields can be used to store multiple values in a limited space.
-
Data Packing and Unpacking: Combining bitwise operators allows efficient packing of data into a compact format for storage or transmission.
-
Error Detection and Correction: Bitwise operations can be used to detect and correct errors in data transmission.
-
Cryptography: Bitwise operators are fundamental in cryptographic algorithms like XOR encryption.
In conclusion, bitwise operators are a powerful tool for manipulating binary data at the bit level. Understanding their functionality and practical applications opens up possibilities for efficient and versatile programming.
The above is the detailed content of How Can Bitwise Operators Be Used to Efficiently Manipulate Binary Data?. For more information, please follow other related articles on the PHP Chinese website!