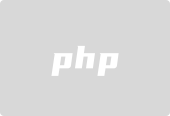
How to Customize Error Messages from Struct Tag Validation
In Gin 1.17, using struct tag validation for data validation before database operations is straightforward. While the default error messages are verbose, they can be customized to provide clearer user feedback.
Overview of the Problem
Gin employs the github.com/go-playground/validator/v10 package for validation. When validation fails, it returns a validator.ValidationErrors object. This verbose error message contains information about each field that failed validation.
Customizing Error Messages
To obtain customized error messages, you can:
- Unwrap the standard error type using the errors package.
- Access the individual validator.FieldError objects from the unwrapped validator.ValidationErrors.
- Construct a custom error model and populate it with the field name and a custom error message determined by the field's validation tag.
Implementation Example
Here's an implementation that translates validation errors into a JSON response with a dynamic field-based error structure:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | type ApiError struct {
Field string
Msg string
}
func HandleValidationErrors(c *gin.Context, err error) {
var ve validator.ValidationErrors
if errors.As(err, &ve) {
out := make([]ApiError, len(ve))
for i, fe := range ve {
out[i] = ApiError{fe.Field(), msgForTag(fe.Tag())}
}
c.JSON(http.StatusBadRequest, gin.H{ "errors" : out})
}
}
func msgForTag(tag string) string {
switch tag {
case "required" :
return "This field is required"
case "email" :
return "Invalid email"
}
return ""
}
|
Copy after login
Usage Example
In a handler function, use HandleValidationErrors to handle validation errors:
1 2 3 4 5 6 7 8 9 | func UserHandler(c *gin.Context) {
var u User
err := c.BindQuery(&u);
if err != nil {
HandleValidationErrors(c, err)
return
}
}
|
Copy after login
The above is the detailed content of How to Customize Validation Error Messages in Gin 1.17 Using Struct Tags?. For more information, please follow other related articles on the PHP Chinese website!