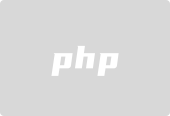
How to Write Wrappers for C Standard Library Functions
Using the standard namespace can clutter code; however, typing std:: before every instance of cout, cerr, cin, and endl can be tedious. This article explores a possible solution and considers other implications.
Proposed Wrapper
The following code provides an alternative approach using wrappers:
#include <iostream>
#include <string>
extern std::ostream& Cout;
extern std::ostream& Cerr;
extern std::istream& Cin;
extern std::string& Endl;
#include "STLWrapper.h"
std::ostream& Cout = std::cout;
std::ostream& Cerr = std::cerr;
std::istream& Cerr = std::cin;
std::string _EndlStr("\n");
std::string& Endl = _EndlStr;
Copy after login
This approach works but raises some questions:
Potential Issues
-
Namespace Overloading: Using wrappers introduces the possibility of name collisions with overloaded functions in the global namespace.
-
Unintentional Name Shadowing: Using short wrappers may unintentionally shadow variables or functions in the local scope.
-
Increased Complexity: The wrapper solution adds an extra layer of complexity to the codebase.
Alternative Perspectives
-
Avoid Namespace Declarations: While wrappers provide a workaround, it's generally recommended to avoid using namespace declarations (using namespace std) and instead use fully qualified names (std::cout). This enhances code clarity and reduces the risk of overloading issues.
-
Consider Readability: The length of identifiers is a trade-off between writing time and readability. While shorter identifiers may make code writing easier, longer identifiers may improve readability and understanding. Studies indicate that using fully qualified names can make code easier to read and interpret.
-
Exceptions: The std::swap function is an exception where using using can be beneficial, as it allows you to use swap(a, b) without specifying the namespace.
The above is the detailed content of Should You Use C Wrappers for Standard Library Functions?. For more information, please follow other related articles on the PHP Chinese website!