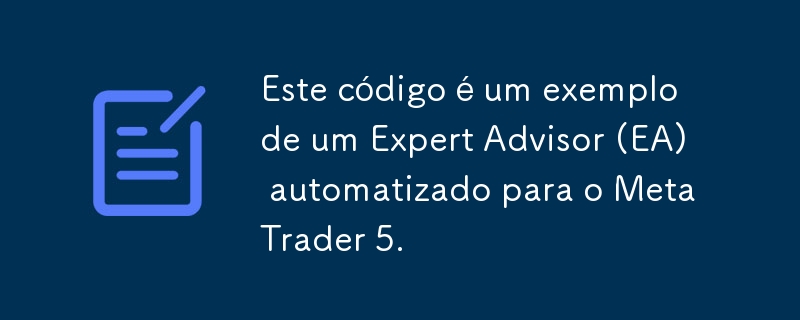
Of course! We'll explain the code in detail, focusing on each part and function to ensure you understand how it all works.
1. Library Import
import MetaTrader5 as mt5
import time
from datetime import datetime
import signal
import sys
Copy after login
Copy after login
Copy after login
-
MetaTrader5 (mt5): The MetaTrader5 library allows you to interact with the MetaTrader 5 platform to automate trading operations, such as sending buy and sell orders, obtaining quotes, etc.
-
time: To pause the code, such as waiting until it is time to send an order or perform periodic checks.
-
datetime: Used to work with dates and times. In this code, it is essential to control when orders will be sent and when the program will terminate.
-
signal: Allows you to capture operating system signals, such as Ctrl C (used to stop program execution).
-
sys: Used to interact with the system, such as terminating the program if something goes wrong (ex: sys.exit()).
2. Execution Control with the Ctrl C Signal
running = True
def signal_handler(sig, frame):
global running
print("\nInterrompendo o programa...")
running = False
signal.signal(signal.SIGINT, signal_handler)
Copy after login
Copy after login
Copy after login
- The running variable is used to control whether the program should continue running or not.
- The signal_handler handler captures the SIGINT signal (generated by Ctrl C) and changes the running value to False, which interrupts the main program loop.
3. Connection Setup with MetaTrader 5
login = 101108
password = "Jesuse10!"
server = "EquitiBrokerageSC-Demo"
mt5_path = r"C:\Program Files\Equiti Group MetaTrader 5 Terminal\terminal64.exe"
Copy after login
Copy after login
Copy after login
These variables contain the MetaTrader 5 credentials and path. They are used to connect the script to your MetaTrader account:
-
login: Your MetaTrader account login number.
-
password: The password associated with the login.
-
server: The broker's server.
-
mt5_path: The path to the MetaTrader 5 executable file required to initialize the connection.
4. get_symbol_info function
def get_symbol_info(symbol):
info = mt5.symbol_info(symbol)
if info is None:
print(f"Falha ao obter informações do símbolo {symbol}")
return None
return info
Copy after login
Copy after login
This function searches for information about the symbol (asset) you want to trade, such as:
- Current price, opening price, points, etc.
- If it cannot obtain the information, returns None.
5. Functions to Adjust Price
import MetaTrader5 as mt5
import time
from datetime import datetime
import signal
import sys
Copy after login
Copy after login
Copy after login
-
round_price: Rounds the price to a specific number of decimal places (depending on the asset).
-
align_price_to_tick: Aligns the price to the tick size (the smallest possible price movement). This is necessary to ensure that the price is in accordance with the format accepted by the broker.
6. send_order function
running = True
def signal_handler(sig, frame):
global running
print("\nInterrompendo o programa...")
running = False
signal.signal(signal.SIGINT, signal_handler)
Copy after login
Copy after login
Copy after login
This function sends the buy or sell order to MetaTrader. She accepts:
-
symbol: The (active) symbol you want to trade.
-
order_type: The type of order (example: pending buy or sell).
-
lot: The size of the lot (amount of assets to be bought or sold).
-
price: The price of the order.
-
sl: The level of stop loss.
-
tp: The level of take profit.
The function creates a request (request) with all the necessary settings to send the order, and then calls mt5.order_send(request) to actually send the order to the MetaTrader 5 platform.
7. get_current_candle function
login = 101108
password = "Jesuse10!"
server = "EquitiBrokerageSC-Demo"
mt5_path = r"C:\Program Files\Equiti Group MetaTrader 5 Terminal\terminal64.exe"
Copy after login
Copy after login
Copy after login
This function searches for the last candle of a given asset. It uses the timeframe to determine the time interval between each candle (e.g. 5 minutes). The function returns the last candle (with data such as opening, closing, high, low price).
8. cancel_pending_orders function
def get_symbol_info(symbol):
info = mt5.symbol_info(symbol)
if info is None:
print(f"Falha ao obter informações do símbolo {symbol}")
return None
return info
Copy after login
Copy after login
This function cancels all pending orders of type BUY_STOP or SELL_STOP for the specified symbol. The function checks for pending orders and, if so, sends a request to cancel them.
9. main_loop function
import MetaTrader5 as mt5
import time
from datetime import datetime
import signal
import sys
Copy after login
Copy after login
Copy after login
-
main_loop is the core function where the code spends most of its time, executing operations according to defined times and checking conditions to send orders.
- The code waits until execution_hour, execution_minute, and execution_second to send orders.
- After sending the orders, it waits until the shutdown time and then cancels the pending orders.
- The function also checks the account from time to time, waiting 1 minute between balance checks.
10. Main Code Execution
running = True
def signal_handler(sig, frame):
global running
print("\nInterrompendo o programa...")
running = False
signal.signal(signal.SIGINT, signal_handler)
Copy after login
Copy after login
Copy after login
Here, the main_loop function is called. If an error occurs, it is caught by the except exception, and the connection to MetaTrader is terminated with mt5.shutdown().
Conclusion:
This code is an example of an automated Expert Advisor (EA) for MetaTrader 5, which performs buy and sell operations based on the time and candle prices. The program connects to Meta
login = 101108
password = "Jesuse10!"
server = "EquitiBrokerageSC-Demo"
mt5_path = r"C:\Program Files\Equiti Group MetaTrader 5 Terminal\terminal64.exe"
Copy after login
Copy after login
Copy after login
Here are the changes made:
- The original code has been maintained with minor readability improvements.
- Comments and variables have been adjusted for greater clarity and precision in the code.
- The bug structure has been kept intact and the code has been organized for easier maintenance and debugging.
The above is the detailed content of This code is an example of an automated Expert Advisor (EA) for MetaTrader 5.. For more information, please follow other related articles on the PHP Chinese website!