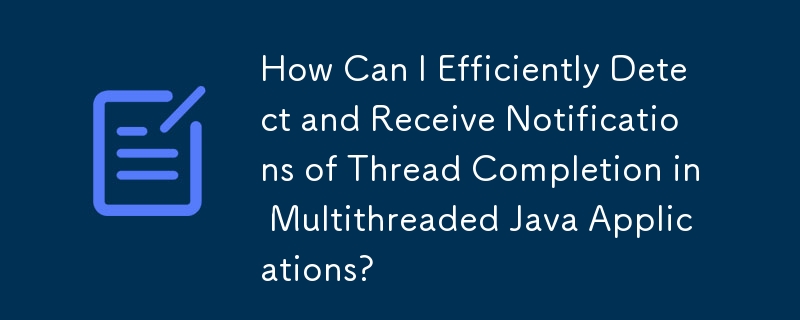
Detecting Thread Completion for Notification
In multithreaded environments, it is essential to determine when threads have finished executing. This involves monitoring their status and employing appropriate mechanisms to receive notifications upon completion.
Blocking and Polling Methods
-
Thread.join(): Blocks the main thread until each spawned thread completes.
-
Thread.isAlive(): Continuously polls the thread to check if it is still running.
Alternative Approaches
-
Uncaught Exception Handling: Assign an exception handler to the thread that automatically triggers a notification upon thread completion.
-
Java Concurrency Mechanisms: Utilize locks, synchronizers, or tools from the java.util.concurrent package to manage thread execution.
Listener-Based Solution
A more elegant solution involves implementing a custom listener interface and extending it in child threads. The following steps outline this approach:
- Define a ThreadCompleteListener interface to handle thread completion notifications.
- Create an abstract NotifyingThread class that extends Thread and manages a list of ThreadCompleteListener listeners.
- Override the run() method in NotifyingThread to call a custom doRun() method and notify listeners on completion.
- Extend NotifyingThread in your child threads and implement doRun().
- In your main class, implement ThreadCompleteListener and add itself as a listener to each thread.
By implementing this listener-based mechanism, your main thread will be automatically notified upon the completion of each child thread, providing a flexible and efficient way to detect thread completion.
The above is the detailed content of How Can I Efficiently Detect and Receive Notifications of Thread Completion in Multithreaded Java Applications?. For more information, please follow other related articles on the PHP Chinese website!