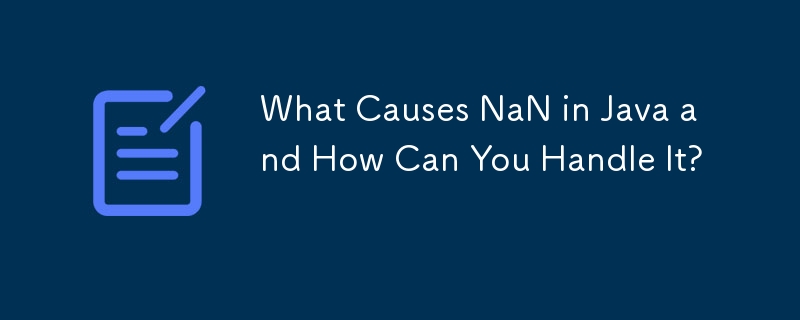
NaN in Java
When encountering unexpected outputs in programming, it's crucial to understand the root cause. In Java, NaN (Not-a-Number) is a special value that indicates an undefined or invalid result. It often arises when performing arithmetic operations with inputs that are mathematically undefined.
Understanding the Origin of NaN
As mentioned, NaN occurs when floating-point operations encounter certain conditions:
-
Division by Zero: Dividing zero by itself (0.0 / 0.0) or any other number is undefined, resulting in NaN.
-
Square Root of Negative Numbers: Java does not support imaginary numbers, so attempting to take the square root of a negative number yields NaN.
-
Other Undefined Cases: Certain trigonometric functions, such as sine (sin) and cosine (cos), may produce NaN for specific input angles.
Implications of NaN
NaN is a problematic value because it does not behave like regular numbers during arithmetic calculations:
-
Inequality Checks Fail: NaN is never equal (==) or not equal (!=) to any other value, including itself.
-
Arithmetic Operations Yield NaN: Performing arithmetic operations on NaN always results in NaN, regardless of the other operand.
-
Conditional Statements Can Be Problematic: Using NaN in conditional statements can lead to unexpected behavior since NaN cannot be compared to any other value.
Handling NaN
To mitigate issues related to NaN, consider the following best practices:
-
Check for NaN Before Calculations: Use Java's Float.isNaN or Double.isNaN methods to determine if a value is NaN before performing calculations.
-
Default Values for NaN: Assign default values to NaN to handle it gracefully and avoid errors.
-
Avoid Invalid Inputs: Improve program robustness by preventing invalid inputs that could result in NaN.
-
Error Handling: Use exception handling to catch and handle situations where NaN arises.
The above is the detailed content of What Causes NaN in Java and How Can You Handle It?. For more information, please follow other related articles on the PHP Chinese website!