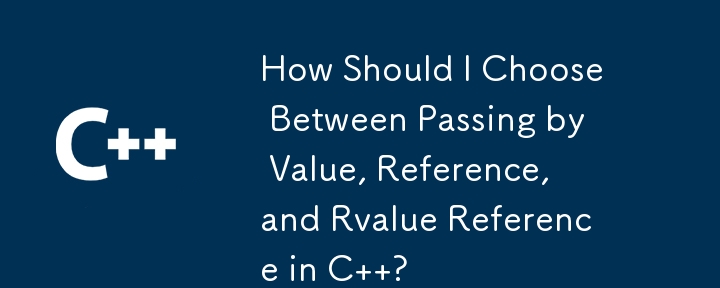
Understanding Parameter Passing in C
When passing parameters in C , selecting the appropriate method (by value, reference, or rvalue reference) ensures efficiency and clarity in code design. Here are best practices for each scenario:
1. Pass by Lvalue Reference:
- When the parameter needs to be modified and these modifications need to be visible to the caller.
- Example: void foo(my_class& obj) allows modifications within the function to reflect changes in the original object.
2. Pass by Lvalue Reference to Const:
- When the parameter's state only needs to be observed, without any required modifications.
- Example: void foo(my_class const& obj) permits access to the object's data without altering its state.
3. Pass by Value:
- For fundamental types (e.g., int, bool) or types with fast copying, this approach prioritizes efficiency.
- Example: void foo(my_class obj) ensures a copy or move occurs, depending on whether an lvalue or rvalue is passed.
4. Consider Overloading for Lvalues and Rvalues:
- For objects with expensive moves, provide separate overloads to handle lvalues and rvalues.
- Example: Overload foo() with my_class& and my_class&& to handle lvalue reference and rvalue reference scenarios, respectively.
5. Utilize Perfect Forwarding:
- Create a function template that accepts an rvalue reference to an unspecified type and forwards it with std::forward.
- Example: template void foo(C&& obj) automatically determines whether to copy or move based on the parameter's type.
Concerning the Provided Code:
Passing CreditCard as an Rvalue Reference:
- The claim of two moves and zero copies is incorrect.
- When passing an rvalue of CreditCard, only one move occurs during reference binding.
Overloading for Constructor:
- Consider creating separate constructor overloads for lvalue reference to CreditCard and rvalue reference to CreditCard.
- This approach guarantees a copy for lvalues and a move for rvalues, enhancing efficiency and meeting specific requirements.
The above is the detailed content of How Should I Choose Between Passing by Value, Reference, and Rvalue Reference in C ?. For more information, please follow other related articles on the PHP Chinese website!