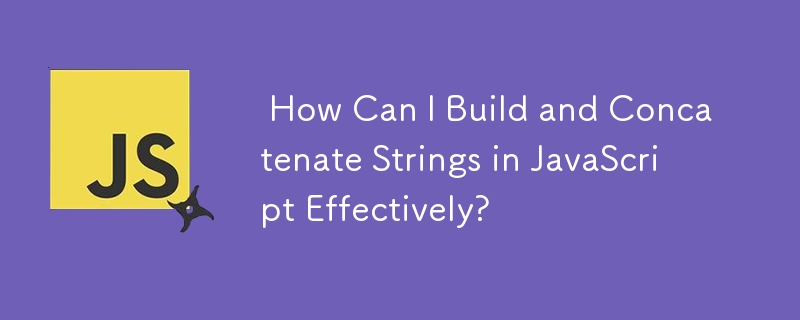
Building and Concatenating Strings in JavaScript: A Comprehensive Guide
Challenge:
As JavaScript projects grow in size and complexity, maintaining well-structured and readable strings becomes increasingly challenging. String concatenation can become tedious and difficult to manage.
Conventional String Concatenation Methods:
JavaScript offers several conventional methods for concatenating strings:
-
Concatenation Shorthand: Append strings using the operator (var x = 'hello' ' ' 'world';)
-
String.concat: Call the concat() method on a string object (var joined = 'hello '.concat('world');)
While these methods are reliable, they can result in verbose and unmanageable string construction.
Modern Approaches:
Template Strings (ES6 ):
- Utilize template strings (introduced in ES6) by enclosing strings in backticks ('hello ${username}')
- Embed variables and expressions within ${} to build complex strings
String.concat (ES5):
- Invoke the concat() method with multiple strings as arguments (var joined = 'hello '.concat(username);)
- Offers a slightly more verbose but more structured approach compared to the concatenation shorthand
Array Methods:
- Leverage array methods like join() to concatenate strings:
(var joined = ['hello', username].join(' '))
- Use reduce() combined with join or other methods for even more complex string manipulation:
(var b = a.reduce((pre, next) => pre ' ' next);)
Third-Party Libraries:
For highly specialized string manipulation requirements, third-party libraries like sprintf.js may offer additional functionality and customization options.
Best Practices:
- Choose an approach that balances readability, maintainability, and browser compatibility
- Prioritize concise and expressive strings
- Consider using placeholders or variables to make strings more dynamic
- Leverage modern features like template strings if possible
The above is the detailed content of How Can I Build and Concatenate Strings in JavaScript Effectively?. For more information, please follow other related articles on the PHP Chinese website!