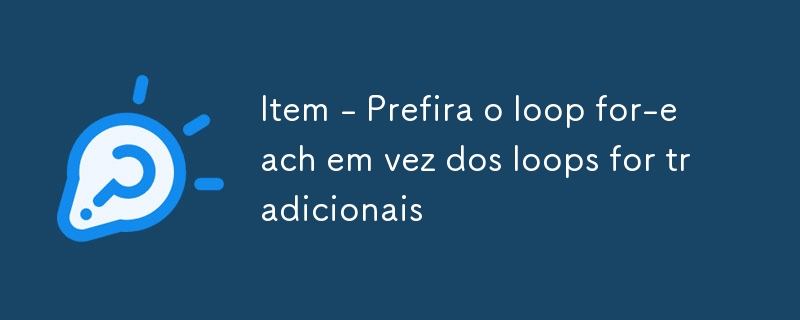
Problems with the traditional for loop:
- Traditional for loops have index variables or iterators, which can generate unnecessary "debris" and increase the chances of error.
- Errors such as using the wrong index or iterator may not be detected by the compiler.
- The traditional loop is different for arrays and collections, making maintenance and switching between types difficult.
Example of traditional for loop in collection:
for (Iterator<String> i = collection.iterator(); i.hasNext();) {
String element = i.next();
// Processa elemento
}
Copy after login
Example of traditional for loop in array:
for (int i = 0; i < array.length; i++) {
String element = array[i];
// Processa elemento
}
Copy after login
Advantages of the for-each loop:
- Simplification: Eliminates unnecessary iterators and indexes, focusing only on the elements.
- Fewer Errors: Reduces the possibility of errors associated with control variables.
- Flexibility: Works the same way for arrays and collections, making it easy to switch between them.
- Performance: There is no loss of performance compared to the traditional for loop.
For-each loop example:
for (String element : collection) {
// Processa elemento
}
for (String element : array) {
// Processa elemento
}
Copy after login
Problems with traditional for loops in nested iterations:
- Using iterators explicitly in nested loops can cause hard-to-detect errors.
- Example: Calling next() on the wrong iterator may throw an exception or generate unexpected results.
Common error in nested loops with iterators:
for (Iterator<Suit> i = suits.iterator(); i.hasNext();) {
for (Iterator<Rank> j = ranks.iterator(); j.hasNext();) {
System.out.println(i.next() + " " + j.next());
}
}
Copy after login
Fix with nested for-each loops:
- Using for-each loops automatically eliminates these errors as there is no explicit control of iterators.
Correct example with for-each:
for (Suit suit : suits) {
for (Rank rank : ranks) {
System.out.println(suit + " " + rank);
}
}
Copy after login
Limitations of the for-each loop:
- Destructive filtering: Does not allow removing elements during iteration, making it necessary to use an explicit iterator or methods such as removeIf (Java 8).
- Transformation: If you need to modify the elements of a list or array, the for-each loop is not enough, as you need to access the index.
- Parallel Iteration: When it is necessary to iterate over multiple collections in parallel, the for-each loop does not work properly, as explicit control over the indexes is required.
Example of transformation with traditional for loop:
for (int i = 0; i < list.size(); i++) {
list.set(i, modify(list.get(i)));
}
Copy after login
Iterable Interface:
- The for-each loop works with any object that implements the Iterable interface, making it easier to iterate over new types of collections.
Iterable Interface:
public interface Iterable<T> {
Iterator<T> iterator();
}
Copy after login
Conclusion:
- Always prefer for-each loops for clarity, security and flexibility. Use traditional for loops only when really necessary (transformation, destructive filtering, or parallel iteration).
The above is the detailed content of Item - Prefer for-each loop over traditional for loops. For more information, please follow other related articles on the PHP Chinese website!