In Java, Scanner is a class that is used for getting the input of strings and different primitive types such as int, double, etc. The scanner class is found in the package java. It extends the class Object and implements the interfaces Closeable and Iterator. Inputs are broken into classes with the help of a whitespace delimiter. Declaration, syntax, working, and several other aspects of the Scanner class in Java will be discussed in the following sections.
Start Your Free Software Development Course
Web development, programming languages, Software testing & others
Java Scanner Class Declaration
Java Scanner class is declared as shown below.
public final class Scanner extends Object implements Iterator<String>
Copy after login
Syntax:
Scanner class can be used in the below syntax in Java programs.
Scanner sc = new Scanner(System.in);
Copy after login
Here, sc is the scanner object and System.in tells Java that the input will be this.
How Does Java Scanner Class Work?
Now, let us see how the Scanner class works.
- Import the class java.util.Scanner or the whole package java.util.
import java.util.*;
import java.util.Scanner;
Copy after login
- Create scanner object as shown in the syntax.
Scanner s = new Scanner(System.in);
Copy after login
- Declare a type int, char or string.
int n = sc.nextInt();
Copy after login
- Perform the operations on the input based on the requirement.
Constructors
The scanner class in Java has different constructors. They are:
-
Scanner(File src): A new scanner will be constructed that generates value from the mentioned file.
-
Scanner(File src, String charsetName): A new scanner will be constructed that generates value scanned from the mentioned file.
-
Scanner(InputStream src): A new scanner will be constructed that generates value from the mentioned input stream.
-
Scanner(InputStream src, String charsetName): A new scanner will be constructed that generates value from the mentioned input stream.
-
Scanner(Path src): A new scanner will be constructed that generates value from the mentioned file.
-
Scanner(Path src, String charsetName): A new scanner will be constructed that generates value from the mentioned file.
-
Scanner(Readable src): A new scanner will be constructed that generates value from the mentioned source.
-
Scanner(ReadableByteChannel src): A new scanner will be constructed that generates value from the mentioned channel.
-
Scanner(ReadableByteChannel src, String charsetName): A new scanner will be constructed that generates value from the mentioned channel.
-
Scanner(String src): A new scanner will be constructed that generates value from the mentioned string.
Methods of Java Scanner Class
Following are the methods that perform different functionalities.
-
close(): Scanner gets closed on calling this method.
-
findInLine(Pattern p): Next occurrence of the mentioned pattern p will be attempted to find out, ignoring the delimiters.
-
findInLine(String p): The next occurrence of the pattern that is made from the string will be attempted to find out, ignoring the delimiters.
-
delimiter(): Pattern that is currently used by the scanner to match delimiters will be returned.
-
findInLine(String p): The next occurrence of the pattern that is made from the string will be attempted to find out, ignoring the delimiters.
-
findWithinHorizon(Pattern p, int horizon): Tries to identify the mentioned pattern’s next occurrence.
-
hasNext(): If the scanner has another token in the input, true will be returned.
-
findWithinHorizon(String p, int horizon): Tries to identify the next occurrence of the mentioned pattern made from the string p, ignoring delimiters.
-
hasNext(Pattern p): If the next token matches the mentioned pattern p, true will be returned.
-
hasNext(String p): If the next token matches the mentioned pattern p made from the string p, true will be returned.
-
next(Pattern p): Next token will be returned, which matches the mentioned pattern p.
-
next(String p): Next token will be returned, which matches the mentioned pattern p made from the string p.
-
nextBigDecimal(): Input’s next token will be scanned as a BigDecimal.
-
nextBigInteger(): Input’s next token will be scanned as a BigInteger.
-
nextBigInteger(int rad): Input’s next token will be scanned as a BigInteger.
-
nextBoolean(): Input’s next token will be scanned as a Boolean, and it will be returned.
-
nextByte(): Input’s next token will be scanned as a byte.
-
nextByte(int rad): Input’s next token will be scanned as a byte.
-
nextDouble(): Input’s next token will be scanned as a double.
-
nextFloat(): Input’s next token will be scanned as a float.
-
nextInt(): Input’s next token will be scanned as an integer.
-
nextInt(int rad): Input’s next token will be scanned as an integer.
-
nextLong(int rad): Input’s next token will be scanned as a long.
-
nextLong(): Input’s next token will be scanned as a long.
-
nextShort(int rad): Input’s next token will be scanned as a short.
-
nextShort(): Input’s next token will be scanned as a short.
-
radix(): The default radix of the scanner will be returned.
-
useDelimiter(Pattern p): Delimiting pattern of the scanner will be set to the mentioned pattern.
-
useDelimiter(String p): Delimiting pattern of the scanner will be set to the mentioned pattern made from the string p.
-
useRadix(int rad): The scanner’s default radix will be set to the mentioned radix.
-
useLocale(Locale loc): The locale of the scanner will be set to the mentioned locale.
Examples of Java Scanner Class
Now, let us see some sample programs of the Scanner class in Java.
Example #1
Code:
import java.util.Scanner;
class ScannerExample {
public static void main(String[] args) {
//create object of scanner
Scanner sc = new Scanner(System.in);
System.out.println("Enter the username");
String obj = sc.nextLine();
System.out.println("The name you entered is: " + obj);
}
}
Copy after login
Output:
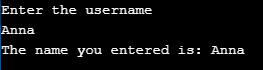
On executing the program, the user will be asked to enter a name. As the input is a string, the nextLine() method will be used. Once it is entered, a line will be printed displaying the name user given as input.
Example #2
Code:
import java.util.Scanner;
class ScannerExample {
public static void main(String[] args) {
//create object of scanner
Scanner sc = new Scanner(System.in);
System.out.println("Enter age");
int obj = sc.nextInt();
System.out.println("The age you entered is: " + obj);
}
}
Copy after login
Output:
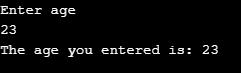
On executing the program, the user will be asked to enter the age. As the input is a number, the nextInt() method will be used. Once it is entered, a line will be printed displaying the age user given as input.
The above is the detailed content of Java Scanner Class. For more information, please follow other related articles on the PHP Chinese website!