This article explores the various types of touch events supported by mobile devices, including tap, double tap, long press, swipe, pinch, rotate, and pan. It provides guidance on distinguishing between different touch events using event object proper
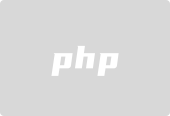
What are the various types of touch events supported by mobile devices?
Mobile devices support a wide range of touch events that allow users to interact with the device's screen. The most common touch events include:
-
Tap: A single tap on the screen, often used to select or activate an element.
-
Double tap: A quick double tap on the screen, often used to zoom in or out of content.
-
Long press: A prolonged press on the screen, often used to open a context menu or trigger a specific action.
-
Swipe: A finger movement across the screen, often used to navigate through content or dismiss notifications.
-
Pinch: A two-finger movement that brings the fingers together or spreads them apart, often used to zoom in or out of content.
-
Rotate: A two-finger movement that rotates the fingers around each other, often used to rotate an object or image.
-
Pan: A two-finger movement that drags the screen in a specific direction, often used to scroll through content or move an object.
How can I distinguish between different touch events (e.g., tapping, swiping)?
Distinguishing between different touch events requires analyzing the properties of the event object. The event object contains information about the touch point, such as the position, pressure, and type of touch (e.g., finger, stylus). By examining these properties, you can determine the type of touch event that occurred.
Here is an example of how to distinguish between a tap and a swipe event using JavaScript:
element.addEventListener('touchstart', (e) => {
// Start position of the touch
let startPosition = { x: e.touches[0].clientX, y: e.touches[0].clientY };
});
element.addEventListener('touchend', (e) => {
// End position of the touch
let endPosition = { x: e.changedTouches[0].clientX, y: e.changedTouches[0].clientY };
// Calculate the distance and direction of the swipe
let distance = calculateDistance(startPosition, endPosition);
let direction = calculateDirection(startPosition, endPosition);
// If the distance is less than a threshold, it's a tap
if (distance < TAP_THRESHOLD) {
handleTap();
}
// Otherwise, it's a swipe
else {
handleSwipe(direction);
}
});
Copy after login
What best practices should I follow when handling touch events in mobile applications?
When handling touch events in mobile applications, it's essential to follow certain best practices to ensure a smooth and responsive user experience. Here are some recommendations:
-
Use the right event listeners: Choose the appropriate event listeners based on the desired touch behavior. For example, use 'touchstart' to capture the start of a touch event and 'touchend' to capture the end of a touch event.
-
Handle touch events consistently: Ensure that touch events are handled in a consistent manner throughout the application. Define a set of standards for handling touch events and adhere to it throughout the codebase.
-
Optimize touch event handling: Avoid unnecessary touch event handling that can consume resources and slow down the application. Only handle touch events that are essential for the application's functionality.
-
Provide visual feedback: Provide visual feedback to users when they interact with touch events. For example, display a visual cue when a button is pressed or an item is dragged.
-
Test the application thoroughly: Test the application thoroughly on different mobile devices to ensure that touch events are handled correctly in various scenarios.
The above is the detailed content of What are the touch events on mobile terminals?. For more information, please follow other related articles on the PHP Chinese website!