Using Java frameworks simplifies web application development but requires ensuring security. Common security considerations include SQL injection, XSS, SSRF, and RCE. Mitigation measures include: using prepared statements to prevent SQL injection; HTML escaping and CSP to prevent XSS; verifying sources, rate limiting, and whitelisting to prevent SSRF; promptly updating frameworks and using security functions to prevent RCE. Implementing these measures reduces the risk of vulnerabilities and protects application security.
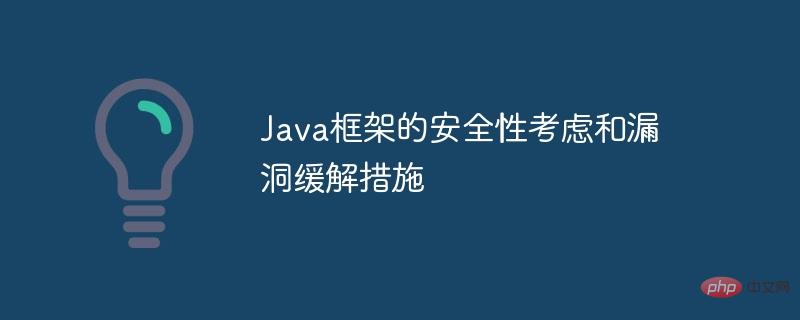
Security considerations and vulnerability mitigation measures for Java frameworks
Using Java frameworks can simplify the development of web applications, but only if they are secure. This article will explore common Java framework security considerations and provide mitigations to help protect your applications.
Common Security Considerations
- ##SQL injection: An attacker injects malicious SQL queries to perform unauthorized operations.
- Cross-site scripting (XSS): The attacker injects malicious code and executes it in the victim's browser, causing session hijacking or data theft.
- Server Side Request Forgery (SSRF): An attacker tricks an application into sending a request to an unauthorized server.
- Remote Code Execution (RCE): An attacker exploits a code vulnerability to execute arbitrary code on the application server.
- Buffer overflow: The attacker sends excessive data to the application, causing buffer overflow and damaging the integrity of the program.
Vulnerability Mitigation Measures
SQL Injection
Use prepared statements or parameterized queries to prevent unescaped User input is injected into the SQL query. - Validate and filter user input, using regular expressions or whitelists.
-
Cross-site scripting
Use HTML escaping to prevent malicious HTML code from being executed in the browser. - Enable Content Security Policy (CSP) to limit the scripts and styles that an application can load.
- Validate and filter user-generated HTML content.
-
Server-side request forgery
Verify the origin of the request, using a whitelist of IP addresses or a checksum. - Restrict the external URLs that the application can access.
- Implement rate limiting to prevent large numbers of unauthorized requests.
-
Remote Code Execution
Update frameworks and libraries in a timely manner and patch known vulnerabilities. - Use input validation and data type checking to prevent malicious input from executing code.
- Deploy a Web Application Firewall (WAF) to detect and block malicious HTTP requests.
-
Buffer overflow
Use secure coding practices to avoid buffer overflows. - Use safe functions provided by the library or framework, such as
- String.copy()
instead of using raw pointers directly.
Practical case
SQL injection mitigation:
// 使用预编译语句
PreparedStatement ps = connection.prepareStatement("SELECT * FROM users WHERE name = ?");
ps.setString(1, username);
Copy after login
XSS mitigation:
// HTML转义用户输入
String escapedInput = HtmlUtils.htmlEscape(userInput);
Copy after login
SSRF Mitigation:
// 验证请求的来源
if (request.getRemoteAddr().startsWith("192.168.1.")) {
// 允许内部网络的请求
} else {
// 拒绝外部网络的请求
}
Copy after login
By following these mitigations, you can significantly reduce the risk of vulnerabilities in Java frameworks and protect your web applications from attacks.
The above is the detailed content of Security considerations and vulnerability mitigation measures for Java frameworks. For more information, please follow other related articles on the PHP Chinese website!