
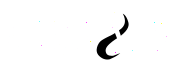
PHP 8.3 ist ein großes Update der PHP-Sprache.
Es enthält viele neue Funktionen, wie z. B. explizite Typisierung von Klassenkonstanten, Deep-Cloning von schreibgeschützten Eigenschaften und Ergänzungen zur Zufallsfunktionalität. Wie immer beinhaltet es auch Leistungsverbesserungen, Fehlerbehebungen und allgemeine Bereinigungen.
Jetzt auf PHP 8.3 upgraden!Typisierte Klassenkonstanten
interface
I
{
// We may naively assume that the PHP constant is always a string.
const
PHP
=
'PHP 8.2'
;
}
class
Foo
implements
I
{
// But implementing classes may define it as an array.
const
PHP
=
[];
}

interface
I
{
const
string PHP
=
'PHP 8.2'
;
}
class
Foo
implements
I
{
const
string PHP
=
[];
}
// Fatal error: Cannot use array as value for class constant
// Foo::PHP of type string
Dynamischer Klassenkonstantenabruf
class
Foo
{
const
PHP
=
'PHP 8.2'
;
}
$searchableConstant
=
'PHP'
;
var_dump
(
constant
(
Foo
::class .
"::
{
$searchableConstant
}
"
));

class
Foo
{
const
PHP
=
'PHP 8.3'
;
}
$searchableConstant
=
'PHP'
;
var_dump
(
Foo
::
{
$searchableConstant
}
);
New #[Override] attribute
use
PHPUnit\Framework\TestCase
;
final class
MyTest
extends
TestCase
{
protected
$logFile
;
protected function
setUp
():
void
{
$this
->
logFile
=
fopen
(
'/tmp/logfile'
,
'w'
);
}
protected function
taerDown
():
void
{
fclose
(
$this
->
logFile
);
unlink
(
'/tmp/logfile'
);
}
}
// The log file will never be removed, because the
// method name was mistyped (taerDown vs tearDown).

use
PHPUnit\Framework\TestCase
;
final class
MyTest
extends
TestCase
{
protected
$logFile
;
protected function
setUp
():
void
{
$this
->
logFile
=
fopen
(
'/tmp/logfile'
,
'w'
);
}
#[
\Override
]
protected function
taerDown
():
void
{
fclose
(
$this
->
logFile
);
unlink
(
'/tmp/logfile'
);
}
}
// The log file will never be removed, because the
// method name was mistyped (taerDown vs tearDown).
By adding the #[Override] attribute to a method, PHP will ensure that a method with the same name exists in a parent class or in an implemented interface. Adding the attribute makes it clear that overriding a parent method is intentional and simplifies refactoring, because the removal of an overridden parent method will be detected.
Deep-Cloning von schreibgeschützten Eigenschaften
class
PHP
{
public
string $version
=
'8.2'
;
}
readonly class
Foo
{
public function
__construct
(
public
PHP $php
) {}
public function
__clone
():
void
{
$this
->
php
= clone
$this
->
php
;
}
}
$instance
= new
Foo
(new
PHP
());
$cloned
= clone
$instance
;
// Fatal error: Cannot modify readonly property Foo::$php

class
PHP
{
public
string $version
=
'8.2'
;
}
readonly class
Foo
{
public function
__construct
(
public
PHP $php
) {}
public function
__clone
():
void
{
$this
->
php
= clone
$this
->
php
;
}
}
$instance
= new
Foo
(new
PHP
());
$cloned
= clone
$instance
;
$cloned
->
php
->
version
=
'8.3'
;
Nur-Lese-Eigenschaften können jetzt einmal innerhalb der magischen __clone-Methode geändert werden, um ein tiefes Klonen von Nur-Lese-Eigenschaften zu ermöglichen.
Neue json_validate()-Funktion
function
json_validate
(
string $string
):
bool
{
json_decode
(
$string
);
return
json_last_error
() ===
JSON_ERROR_NONE
;
}
var_dump
(
json_validate
(
'{ "test": { "foo": "bar" }
}'
));
// true

var_dump
(
json_validate
(
'{ "test": { "foo": "bar" }
}'
));
// true
json_validate() ermöglicht die Überprüfung, ob eine Zeichenfolge syntaktisch gültiges JSON ist, ist dabei aber effizienter als json_decode().
Neue Randomizer::getBytesFromString()-Methode
// This function needs to be manually implemented.
function
getBytesFromString
(
string $string
,
int
$length
) {
$stringLength
=
strlen
(
$string
);
$result
=
''
;
for (
$i
=
0
;
$i
<
$length
;
$i
++) {
// random_int is not seedable for testing, but secure.
$result
.=
$string
[
random_int
(
0
,
$stringLength
-
1
)];
}
return
$result
;
}
$randomDomain
=
sprintf
(
"%s.example.com"
,
getBytesFromString
(
'abcdefghijklmnopqrstuvwxyz0123456789'
,
16
,
),
);
echo
$randomDomain
;

// A \Random\Engine may be passed for seeding,
// the default is the secure engine.
$randomizer
= new
\Random\Randomizer
();
$randomDomain
=
sprintf
(
"%s.example.com"
,
$randomizer
->
getBytesFromString
(
'abcdefghijklmnopqrstuvwxyz0123456789'
,
16
,
),
);
echo
$randomDomain
;
Die in PHP 8.2 hinzugefügte Random Extension wurde um eine neue Methode erweitert, um zufällige Strings zu generieren, die nur aus bestimmten Bytes bestehen. Mit dieser Methode kann der Entwickler problemlos zufällige Bezeichner wie Domänennamen und numerische Zeichenfolgen beliebiger Länge generieren.
Neue Methoden Randomizer::getFloat() und Randomizer::nextFloat()
// Returns a random float between $min and $max, both including.
function
getFloat
(
float $min
,
float $max
) {
// This algorithm is biased for specific inputs and may
// return values outside the given range. This is impossible
// to work around in userland.
$offset
=
random_int
(
0
,
PHP_INT_MAX
) /
PHP_INT_MAX
;
return
$offset
* (
$max
-
$min
) +
$min
;
}
$temperature
=
getFloat
(-
89.2
,
56.7
);
$chanceForTrue
=
0.1
;
// getFloat(0, 1) might return the upper bound, i.e. 1,
// introducing a small bias.
$myBoolean
=
getFloat
(
0
,
1
) <
$chanceForTrue
;

$randomizer
= new
\Random\Randomizer
();
$temperature
=
$randomizer
->
getFloat
(
-89.2
,
56.7
,
\Random\IntervalBoundary
::
ClosedClosed
,
);
$chanceForTrue
=
0.1
;
// Randomizer::nextFloat() is equivalent to
// Randomizer::getFloat(0, 1, \Random\IntervalBoundary::ClosedOpen).
// The upper bound, i.e. 1, will not be returned.
$myBoolean
=
$randomizer
->
nextFloat
() <
$chanceForTrue
;
Aufgrund der begrenzten Präzision und der impliziten Rundung von Gleitkommazahlen ist die Generierung einer unverzerrten Gleitkommazahl, die innerhalb eines bestimmten Intervalls liegt, nicht trivial und die häufig verwendeten Userland-Lösungen können verzerrte Ergebnisse oder Zahlen außerhalb des angeforderten Bereichs erzeugen.
Der Randomizer wurde außerdem um zwei Methoden erweitert, um zufällige Floats auf unvoreingenommene Weise zu generieren. Die Randomizer::getFloat()-Methode verwendet den γ-Abschnittsalgorithmus, der in „Drawing Random Floating-Point Numbers from an Interval“ veröffentlicht wurde. Frédéric Goualard, ACM Trans. Modell. Berechnen. Simultan, 32:3, 2022.
Befehlszeilen-Linter unterstützt mehrere Dateien
php -l foo.php bar.php
No syntax errors detected in foo.php

php -l foo.php bar.php
No syntax errors detected in foo.php
No syntax errors detected in bar.php
Der Befehlszeilen-Linter akzeptiert jetzt verschiedene Eingaben für zu lintende Dateinamen
Neue Klassen, Schnittstellen und Funktionen
- Neues DOMElement::getAttributeNames(), DOMElement::insertAdjacentElement(), DOMElement::insertAdjacentText(), DOMElement::toggleAttribute(), DOMNode::contains(), DOMNode::getRootNode(), DOMNode::isEqualNode(), Methoden DOMNameSpaceNode::contains() und DOMParentNode::replaceChildren().
- Neue Methoden IntlCalendar::setDate(), IntlCalendar::setDateTime(), IntlGregorianCalendar::createFromDate() und IntlGregorianCalendar::createFromDateTime().
- Neue Funktionen ldap_connect_wallet() und ldap_exop_sync().
- Neue Funktion mb_str_pad().
- Neue Funktionen posix_sysconf(), posix_pathconf(), posix_fpathconf() und posix_eaccess().
- Neue ReflectionMethod::createFromMethodName()-Methode.
- Neue Funktion socket_atmark().
- Neue Funktionen str_increment(), str_decrement() und stream_context_set_options().
- Neue ZipArchive::getArchiveFlag()-Methode.
- Support for generation EC keys with custom EC parameters in OpenSSL extension.
- Neue INI-Einstellung zend.max_allowed_stack_size zum Festlegen der maximal zulässigen Stapelgröße.
- php.ini unterstützt jetzt die Fallback-/Standardwertsyntax.
- Anonyme Klassen können jetzt schreibgeschützt sein.
Abwertungen und Abwärtskompatibilitätsunterbrechungen
- Geeignetere Datums-/Uhrzeitausnahmen.
- Durch Zuweisen eines negativen Index n zu einem leeren Array wird nun sichergestellt, dass der nächste Index n 1 statt 0 ist.
- Änderungen an der Funktion range().
- Changes in re-declaration of static properties in traits.
- Die U_MULTIPLE_DECIMAL_SEPERATORS-Konstante ist zugunsten von U_MULTIPLE_DECIMAL_SEPARATORS veraltet.
- Die Variante MT_RAND_PHP Mt19937 ist veraltet.
- ReflectionClass::getStaticProperties() ist nicht mehr nullfähig.
- Die INI-Einstellungen „assert.active“, „assert.bail“, „assert.callback“, „assertion.Exception“ und „assert.warning“ sind veraltet.
- Der Aufruf von get_class() und get_parent_class() ohne Argumente ist veraltet.
- SQLite3: Standardfehlermodus auf Ausnahmen eingestellt.
Der Migrationsleitfaden ist im PHP-Handbuch verfügbar. Eine detaillierte Liste der neuen Funktionen und abwärtsinkompatiblen Änderungen finden Sie hier.
Wenn Sie frühere Versionen herunterladen müssen, können Sie diese anzeigen Mehr ChangeLog