这篇文章主要介绍了关于如何使用MixPHP来开发API接口,有着一定的参考价值,现在分享给大家,有需要的朋友可以参考一下
MixPHP 是一款基于 Swoole 的常驻内存型 PHP 高性能框架,框架的高性能特点非常适合开发 API 接口,而且 MixPHP 非常接近传统 MVC 框架,所以开发接口时非常简单。
下面做一个开发 API 接口的简单实例:
从 articles
表,通过 id
获取一篇文章。
访问该接口的 URL:
数据库表结构如下:
1 2 3 4 5 6 7 | CREATE TABLE `articles` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`title` varchar(255) NOT NULL,
`content` varchar(255) NOT NULL,
`dateline` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
|
登录后复制
第一步
修改数据库配置文件,MixPHP 的应用配置文件中,关于数据库的信息都引用了 common/config/database.php 文件。
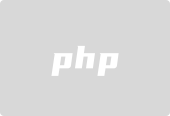
第二步
修改应用配置文件:
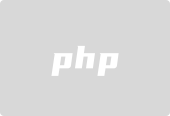
框架默认的 404/500 响应是网页,而 API 服务需要响应 JSON 数据,通常其他传统 MVC 框架需要修改很多地方才可完成这个需求,MixPHP 本身就提供该种配置,只需修改一下配置即可。
MixPHP 的默认 Web 应用中有两个配置文件,分别为:
开发 API 时我们推荐在 Apache/PHP-FPM 下开发,上线再部署至 mix-httpd 即可,反正是无缝切换的。
现在我们修改 response 键名下的 defaultFormat 键为 mixhttpError::FORMAT_JSON,如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | 'response' => [
' class ' => 'mix\http\compatible\Response',
'defaultFormat' => mix\http\Response::FORMAT_JSON,
'json' => [
' class ' => 'mix\http\Json',
],
'jsonp' => [
' class ' => 'mix\http\Jsonp',
'name' => 'callback',
],
'xml' => [
' class ' => 'mix\http\Xml',
],
],
|
登录后复制
然后修改 main_compatible.php 文件中 error 键名下的 format 键为 mixhttpError::FORMAT_JSON,如下:
1 2 3 4 5 6 7 | 'error' => [
' class ' => 'mix\http\Error',
'format' => mix\http\Error::FORMAT_JSON,
],
|
登录后复制
第三步
创建控制器:
1 | apps/index/controllers/ArticlesController.php
|
登录后复制
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | <?php
namespace apps\index\controllers;
use mix\facades\Request;
use mix\http\Controller;
use apps\index\messages\ErrorCode;
use apps\index\models\ArticlesForm;
class ArticlesController extends Controller
{
public function actionDetails()
{
$model = new ArticlesForm();
$model ->attributes = Request::get();
$model ->setScenario('actionDetails');
if (! $model ->validate()) {
return ['code' => ErrorCode::INVALID_PARAM];
}
$data = $model ->getDetails();
if (! $data ) {
return ['code' => ErrorCode::ERROR_ID_UNFOUND];
}
return ['code' => ErrorCode::SUCCESS, 'data' => $data ];
}
}
|
登录后复制
创建错误码类:
1 | apps/index/messages/ErrorCode.php
|
登录后复制
1 2 3 4 5 6 7 8 9 10 11 12 | <?php
namespace apps\index\messages;
class ErrorCode
{
const SUCCESS = 0;
const INVALID_PARAM = 100001;
const ERROR_ID_UNFOUND = 200001;
}
|
登录后复制
创建表单验证模型:
1 | apps/index/models/ArticlesForm.php
|
登录后复制
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | <?php
namespace apps\index\models;
use mix\validators\Validator;
use apps\common\models\ArticlesModel;
class ArticlesForm extends Validator
{
public $id ;
public function rules()
{
return [
'id' => ['integer', 'unsigned' => true, 'maxLength' => 10],
];
}
public function scenarios()
{
return [
'actionDetails' => ['required' => ['id']],
];
}
public function getDetails()
{
return ( new ArticlesModel())->getRowById( $this ->id);
}
}
|
登录后复制
创建数据表模型:
1 | apps/common/models/ArticlesModel.php
|
登录后复制
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | <?php
namespace apps\common\models;
use mix\facades\RDB;
class ArticlesModel
{
const TABLE = 'articles';
public function getRowById( $id )
{
$sql = "SELECT * FROM `" . self::TABLE . "` WHERE id = :id" ;
$row = RDB::createCommand( $sql )->bindParams([
'id' => $id ,
])->queryOne();
return $row ;
}
}
|
登录后复制
以上就是全部代码的编写。
第四步
使用 Postman 测试,如下:
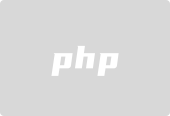
接口开发与测试完成,是不是很简单呀。
以上就是本文的全部内容,希望对大家的学习有所帮助,更多相关内容请关注PHP中文网!
相关推荐:
以上是如何使用MixPHP来开发API接口的详细内容。更多信息请关注PHP中文网其他相关文章!