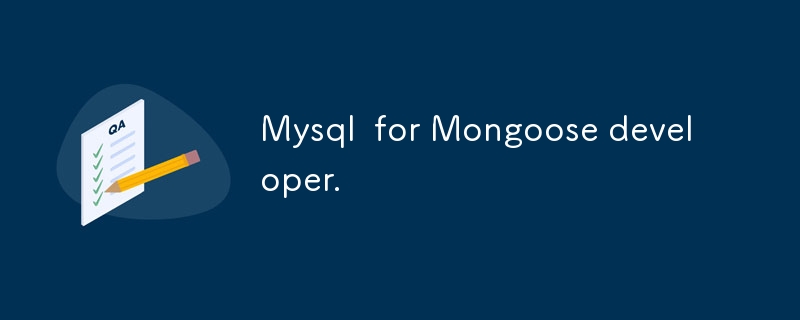
介绍
- 我不在乎。
- MySQL 是一个关系数据库管理系统(RDBMS)。它是一个开源、多用户、多线程数据库系统,允许在表中存储和管理结构化数据。它使用结构化查询语言(SQL)来管理和操作数据。
MySQL 的主要特点:
- 开源
- 跨平台
-
关系数据库:MySQL 基于关系数据库模型,它将数据存储在表(也称为关系)中。
-
高性能:针对速度进行了优化,可以高效处理大量数据。
-
ACID Compliant:MySQL 支持 ACID(原子性、一致性、隔离性、持久性)属性,确保数据库事务得到可靠处理。
- 原子性确保事务被视为单个不可分割的单元。事务中的所有操作要么成功完成,要么不应用任何操作。换句话说,交易是原子的:它是“全有或全无”。
- 一致性确保事务将数据库从一种有效状态转变为另一种有效状态。事务完成后,所有数据必须处于一致状态,遵守所有定义的规则、约束和关系。
- 隔离性确保事务彼此隔离地执行,即使它们同时发生。每笔交易都应该像唯一正在处理的交易一样执行,防止其他交易的干扰。
- 持久性确保事务一旦提交,它就是永久性的,即使在出现断电或崩溃等系统故障的情况下也是如此。事务所做的更改将保存到磁盘,并且不会出现任何后续故障。
-
多用户访问:MySQL允许多个用户同时访问数据库,而不影响性能。
SQL关键字
创造
-
创建数据库
- CREATE DATABASE 命令用于创建新数据库。在 Mongoose 中,您不需要显式创建数据库;当您连接到数据库时它会自动创建。
// DB is created if it doesn't exist
mongoose.connect('mongodb://localhost/my_database');
登录后复制
登录后复制
登录后复制
登录后复制
CREATE DATABASE my_database;
登录后复制
登录后复制
登录后复制
-
使用数据库
- USE DB_NAME 用于选择要使用的数据库。在 Mongoose 中,这是由连接字符串处理的。
mongoose.connect('mongodb://localhost/my_database');
登录后复制
登录后复制
登录后复制
-
创建表
- CREATE TABLE 命令用于在数据库中创建新表。在 Mongoose 中,这类似于创建新集合。
// DB is created if it doesn't exist
mongoose.connect('mongodb://localhost/my_database');
登录后复制
登录后复制
登录后复制
登录后复制
CREATE DATABASE my_database;
登录后复制
登录后复制
登录后复制
-
创建索引
- CREATE INDEX 命令用于在表上创建索引以提高查询性能。在 MongoDB 中,也是一样的。
mongoose.connect('mongodb://localhost/my_database');
登录后复制
登录后复制
登录后复制
描述
- 在 SQL 中用于查看表的结构(其列、数据类型、约束等)。 Mongoose 示例:在 MongoDB 中,没有与 DESCRIBE 直接等效的东西。但是,您可以通过编程方式检查架构。
mongoose.model('User', UserSchema);
登录后复制
登录后复制
CREATE TABLE Users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) NOT NULL UNIQUE
);
登录后复制
登录后复制
插入
- INSERT INTO 命令用于在表中插入新行。在猫鼬中,您可以将新文档插入集合/(模型)中。
UserSchema.index({ email: 1 }); // Unnamed Index
UserSchema.index({ email: 1 }, { name: 'idx_email' }); // Named Index
登录后复制
登录后复制
-- Syntax: CREATE INDEX index_name ON table_name (column_name);
CREATE INDEX idx_email ON Users (email); -- Named Index
CREATE INDEX ON Users (email); -- Unnamed Index
登录后复制
登录后复制
选择
- SQL 中的 SELECT 语句用于从数据库中检索数据。在 Mongoose 中,这相当于使用 .find() 方法来查询集合。
console.log(UserSchema.paths);
// Outputs details about the schema fields and types
登录后复制
登录后复制
更新
- UPDATE语句用于修改表中现有的记录。在 mongoose 中,您使用 find 和 update 或 .update()
// In mongoose its equivalent to .save() or .create();
const newUser = new User({ name: 'John Doe', email: 'john@example.com' });
newUser.save()
登录后复制
登录后复制
INSERT INTO Users (name, email)
VALUES ('John Doe', 'john@example.com');
登录后复制
登录后复制
删除
- DELETE 语句用于删除表中现有的记录。在 mongoose 中,我们会使用deleteOne、deleteMany 或查找并删除。
const users = await User.find(); // Fetches all users
const { name, email } = await User.findById(1); // Fetches user with id = 1
登录后复制
登录后复制
SELECT * FROM Users; -- all users
SELECT name, email FROM Users WHERE id = 1; -- user of id 1
登录后复制
登录后复制
改变
- SQL中的ALTER TABLE语句用于修改现有表的结构(添加列、删除列和修改列)。
在 Mongoose 中,等效操作是修改架构以包含新字段,然后在必要时处理现有文档的更新。
// update all user of name kb
const query = { name: "kb" };
User.update(query, { name: "thekbbohara" })
登录后复制
登录后复制
-- update all user of name kb
UPDATE Users
SET name = "thekbbohara", email = "thekbbohara@gmail.com"
WHERE name = "kb";
登录后复制
登录后复制
加入
- JOIN 子句用于根据两个或多个表之间的相关列组合来自两个或多个表的行。在 MongoDB 中,连接 不像关系数据库那样原生支持。相反,您通常使用聚合管道(如$lookup)来实现类似的功能。
User.deleteOne({ _id: 1 })
// All users whose name is notKb will be deleted.
User.deleteMany({ name: "notKb" })
登录后复制
内连接
- INNER JOIN 关键字选择在两个表中具有匹配值的记录。
DELETE FROM Users WHERE id = 1;
DELETE FROM Users WHERE name = "notKb"
-- All users whose name is notKb will be deleted.
登录后复制
左连接
- LEFT JOIN 关键字返回左表(table1)中的所有记录,以及右表(table2)中的匹配记录(如果有)。
// Update the UserSchema to add the 'age' field
const UserSchema = new mongoose.Schema({
name: String,
email: String,
age: Number, // New field
});
登录后复制
右连接
- RIGHT JOIN 关键字返回右表 (table2) 中的所有记录,以及左表 (table1) 中的匹配记录(如果有)。
-- Adds an 'age' column to the Users table
ALTER TABLE Users ADD age INT;
-- Delete 'Email' column from Users table
ALTER TABLE Users DROP COLUMN email;
-- Makes 'id' column unsigned and auto-incrementing
ALTER TABLE Users MODIFY COLUMN id INT UNSIGNED AUTO_INCREMENT;
登录后复制
交叉连接
- CROSS JOIN 关键字返回两个表(table1 和 table2)中的所有记录。
// DB is created if it doesn't exist
mongoose.connect('mongodb://localhost/my_database');
登录后复制
登录后复制
登录后复制
登录后复制
数据类型
MySQL 中有三种主要数据类型:字符串、数字、日期和时间。但在MongoDB中,有多种数据类型,但它们与MySQL中的不同。 MongoDB使用BSON(Binary JSON)来存储数据,它支持丰富的数据类型。以下是 MySQL 和 MongoDB 中常见数据类型的比较:
字符串数据类型
MySQL |
MongoDB (BSON) |
Notes |
CHAR, VARCHAR
|
String |
Both store textual data. MongoDB's String is analogous to VARCHAR. |
TEXT, TINYTEXT, etc. |
String |
No separate TEXT type in MongoDB; all textual data is stored as String. |
数字数据类型
MySQL |
MongoDB (BSON) |
Notes |
INT, SMALLINT, etc. |
NumberInt |
Represents 32-bit integers. |
BIGINT |
NumberLong |
Represents 64-bit integers. |
FLOAT, DOUBLE
|
NumberDouble |
Represents floating-point numbers. |
DECIMAL, NUMERIC
|
String or custom |
MongoDB doesn't have an exact equivalent; use String for precision. |
日期和时间数据类型
MySQL |
MongoDB (BSON) |
Notes |
DATE |
Date |
Both store date-only values. |
DATETIME, TIMESTAMP
|
Date |
MongoDB stores both date and time as a Date object. |
TIME |
String or custom |
MongoDB does not have a direct TIME type; store as String if needed. |
YEAR |
String or Int
|
Represented using String or NumberInt. |
布尔数据类型
MySQL |
MongoDB (BSON) |
Notes |
BOOLEAN, TINYINT(1)
|
Boolean |
Both store true/false values. |
二进制数据类型
MySQL |
MongoDB (BSON) |
Notes |
BLOB, TINYBLOB, etc. |
BinData |
MongoDB's BinData is used for storing binary data like files. |
JSON/数组数据类型
MySQL |
MongoDB (BSON) |
Notes |
JSON |
Object |
MongoDB natively stores JSON-like documents as Object. |
N/A |
Array |
MongoDB has a native Array type for storing lists of values. |
其他数据类型
MySQL |
MongoDB (BSON) |
Notes |
ENUM |
String or custom |
Use a String field with validation for enumerated values. |
SET |
Array |
Use an Array to represent sets of values. |
N/A |
ObjectId |
Unique identifier type in MongoDB, typically used as a primary key. |
N/A |
Decimal128 |
Used for high-precision decimal numbers in MongoDB. |
主键
// DB is created if it doesn't exist
mongoose.connect('mongodb://localhost/my_database');
登录后复制
登录后复制
登录后复制
登录后复制
CREATE DATABASE my_database;
登录后复制
登录后复制
登录后复制
外键
mongoose.connect('mongodb://localhost/my_database');
登录后复制
登录后复制
登录后复制
数据完整性和约束
-
非空:
确保列不能有 NULL 值。
mongoose.model('User', UserSchema);
登录后复制
登录后复制
CREATE TABLE Users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) NOT NULL UNIQUE
);
登录后复制
登录后复制
-
独特:
确保列中的所有值都是唯一的。
UserSchema.index({ email: 1 }); // Unnamed Index
UserSchema.index({ email: 1 }, { name: 'idx_email' }); // Named Index
登录后复制
登录后复制
-- Syntax: CREATE INDEX index_name ON table_name (column_name);
CREATE INDEX idx_email ON Users (email); -- Named Index
CREATE INDEX ON Users (email); -- Unnamed Index
登录后复制
登录后复制
-
默认:
如果未提供值,则为列分配默认值。
console.log(UserSchema.paths);
// Outputs details about the schema fields and types
登录后复制
登录后复制
-
检查(MySQL 8.0):
确保列中的值满足给定条件。
// In mongoose its equivalent to .save() or .create();
const newUser = new User({ name: 'John Doe', email: 'john@example.com' });
newUser.save()
登录后复制
登录后复制
INSERT INTO Users (name, email)
VALUES ('John Doe', 'john@example.com');
登录后复制
登录后复制
-
自动增量:
自动为列生成唯一值,通常用作主键。
const users = await User.find(); // Fetches all users
const { name, email } = await User.findById(1); // Fetches user with id = 1
登录后复制
登录后复制
SELECT * FROM Users; -- all users
SELECT name, email FROM Users WHERE id = 1; -- user of id 1
登录后复制
登录后复制
仅此而已。一切顺利,请随时留下您的反馈,您可以在这里与我联系:thekbbohara
哦,顺便问一下我们如何设置Mysql。
我推荐使用 docker:
// update all user of name kb
const query = { name: "kb" };
User.update(query, { name: "thekbbohara" })
登录后复制
登录后复制
-- update all user of name kb
UPDATE Users
SET name = "thekbbohara", email = "thekbbohara@gmail.com"
WHERE name = "kb";
登录后复制
登录后复制
以上是供 Mongoose 开发人员使用的 Mysql。的详细内容。更多信息请关注PHP中文网其他相关文章!