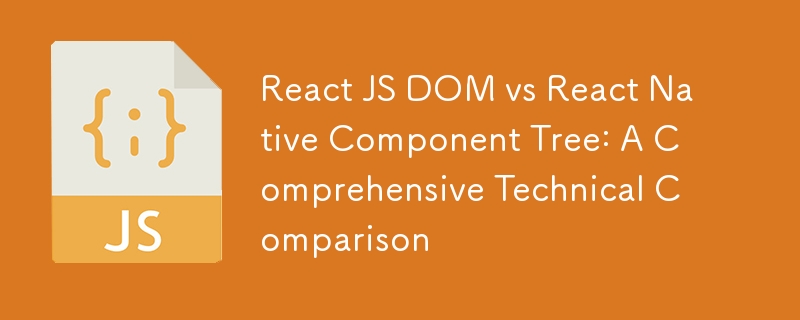
介绍
React JS 和 React Native 虽然共享核心原则,但在渲染和管理 UI 元素的方法上存在显着差异。本文对 React JS 中使用的文档对象模型 (DOM) 和 React Native 使用的组件树结构进行了深入的技术比较,包括 React Native 的新架构。
架构概览
React JS 和 DOM
React JS 在 Web 浏览器中运行,操作文档对象模型 (DOM) 来渲染和更新用户界面。
主要特征:
- 虚拟 DOM:React JS 使用虚拟 DOM 作为抽象层。
- 协调:在虚拟 DOM 和实际 DOM 之间协调更改。
- HTML 元素:UI 组件最终呈现为标准 HTML 元素。
React Native 和组件树
React Native 专为移动平台设计,不与 DOM 交互。相反,它管理特定于移动操作系统(iOS 或 Android)的本机组件树。
主要特征:
- 原生组件:UI 元素映射到特定于平台的原生组件。
- 桥接器:JavaScript 核心通过桥接器与本机模块进行通信。
- 影子树:用C语言维护组件的影子树,用于布局计算。
React Native 的新架构
React Native 正在过渡到新的架构,该架构显着改变了它处理渲染和本机交互的方式:
-
Fabric:一种新的渲染系统,可提高 UI 响应能力并允许更多并发操作。
-
TurboModules:增强的本机模块系统,提供类型安全接口和延迟加载功能。
渲染过程
反应JS
- JSX 被转换为 React.createElement() 调用。
- 虚拟 DOM 根据状态或 prop 更改进行更新。
- 协调算法将虚拟 DOM 与实际 DOM 进行比较。
- 必要的更新会批量并应用到真实的 DOM。
<p>// React JS Component<br>
function WebButton({ onPress, title }) {<br>
return (<br>
<button onClick={onPress} className="web-button"><br>
{title}<br>
</button><br>
);<br>
}</p>
登录后复制
反应本机
传统建筑:
- JSX 被转换为 React.createElement() 调用(类似于 React JS)。
- React Native 创建原生组件的实例,而不是 DOM 节点。
- 影子树已更新以进行布局计算。
- 原生 UI 组件通过特定于平台的 API 进行更新。
新架构(结构):
- JSX 仍然被转换为 React.createElement() 调用。
- 渲染现在用 C 完成,允许更多同步操作。
- 阴影树和布局计算与原生渲染更加紧密地结合。
- 可以更有效地应用更新,可能在单个框架中。
<p>// React Native Component (works with both architectures)<br>
import { TouchableOpacity, Text } from 'react-native';</p>
<p>function NativeButton({ onPress, title }) {<br>
return (<br>
<TouchableOpacity onPress={onPress}><br>
<Text>{title}</Text><br>
</TouchableOpacity><br>
);<br>
}</p>
登录后复制
性能影响
反应JS
- 优点:
- 虚拟 DOM 最大限度地减少实际 DOM 操作,从而提高性能。
- 批量更新减少了回流和重绘操作。
- 挑战:
- 大型 DOM 仍然会导致性能问题。
- 复杂的协调在计算上可能会很昂贵。
反应本机
传统建筑:
- 优点:
- 直接映射到本机组件可提供接近本机的性能。
- C 中的影子树可实现高效的布局计算。
- 挑战:
- 桥梁通信可能成为复杂交互的瓶颈。
- 大型列表或复杂的动画可能需要额外的优化。
新架构:
- Advantages:
- Fabric allows for more synchronous operations, reducing bridge-related bottlenecks.
- TurboModules provide lazy loading and more efficient native module interactions.
- Improved type safety and potential for better performance optimizations.
- Challenges:
- Migration from the old architecture may require significant effort for existing apps.
- Developers need to learn new concepts and potentially update their coding practices.
Developer Experience and Tooling
React JS
- Familiar web development paradigms and tools.
- Rich ecosystem of web-specific libraries and frameworks.
- Browser DevTools for debugging and performance profiling.
React Native
Traditional Architecture:
- Requires understanding of mobile development concepts.
- Platform-specific APIs and components need separate handling.
- Custom tooling like React Native Debugger and platform-specific profilers.
New Architecture:
- Introduces new concepts like Fabric and TurboModules that developers need to understand.
- Improved type safety with CodeGen for better developer experience.
- Enhanced debugging capabilities, especially for native module interactions.
Code Reusability and Cross-Platform Development
Shared Concepts
Both React JS and React Native share core concepts:
- Component-based architecture
- Unidirectional data flow
- Virtual representation of the UI
Divergences
-
UI Components:
- React JS uses HTML elements and CSS for styling.
- React Native uses platform-specific components and a subset of CSS properties.
-
Event Handling:
- React JS: DOM events (e.g., onClick, onChange)
- React Native: Touch events (e.g., onPress) and custom APIs
-
Layout:
- React JS: Flexbox, CSS Grid, and traditional CSS layouts
- React Native: Primarily Flexbox with some limitations
-
Native Functionality:
- React JS: Limited to web APIs and browser capabilities.
- React Native: Access to platform-specific APIs, enhanced with TurboModules in the new architecture.
Example of divergence in layout:
<p>// React JS<br>
<div style={{ display: 'flex', justifyContent: 'center' }}><br>
<span>Centered Content</span><br>
</div></p>
<p>// React Native (both architectures)<br>
import { View, Text } from 'react-native';</p>
<p><View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}><br>
<Text>Centered Content</Text><br>
</View></p>
登录后复制
Implications for Application Architecture
React JS
- Can leverage existing web APIs and browser capabilities.
- SEO considerations may influence component structure.
- Progressive enhancement and accessibility are key concerns.
React Native
Traditional Architecture:
- Must consider platform-specific capabilities and limitations.
- Performance optimization often involves native modules or platform-specific code.
- UI consistency across platforms requires careful component design.
New Architecture:
- Allows for more efficient bridge communication, potentially simplifying complex interactions.
- TurboModules enable more granular control over native module loading and execution.
- Fabric's synchronous layout capabilities may influence component design and animation strategies.
Conclusion
The architectural differences between React JS and React Native reflect their distinct target environments. React JS manipulates the DOM for web browsers, while React Native interacts with native components on mobile platforms. React Native's new architecture with Fabric and TurboModules represents a significant evolution, addressing performance bottlenecks and enhancing developer experience.
Understanding these differences is crucial for developers working across platforms or deciding between web and native mobile development. Each approach offers unique advantages and challenges, and the choice between them should be based on project requirements, performance needs, and target audience.
As both technologies continue to evolve, we can expect further optimizations and potentially more convergence in development patterns, making it easier to build truly cross-platform applications with React technologies.
以上是React JS DOM 与 React Native 组件树:全面的技术比较的详细内容。更多信息请关注PHP中文网其他相关文章!