Popular Headlines
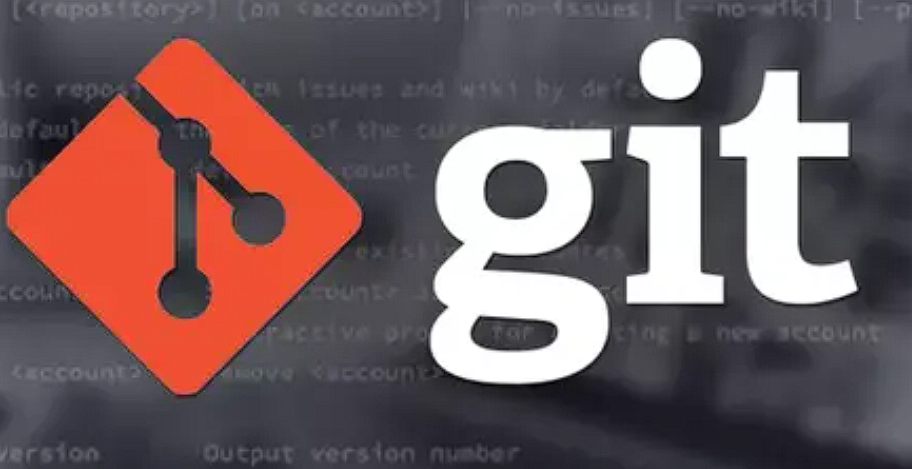
- Vite.js Tutorial – How to Install and Use Vite in Your Web Projects Vite.js is a rapid development tool for modern web projects. It focuses on speed and performance by improving the development experience.Vite uses native browser ES imports to enable support for modern browsers without a build process.
- How to Customize Your tsconfig.json File for Your TypeScript Project The tsconfig.json file is a powerful tool that allows you to customize the behavior of the TypeScript compiler to suit your project’s specific needs. By tweaking the compiler options in this configuration file, you can tailor TypeScript to your proje
- Mastering the Art of Data Engineering to Support Billion-Dollar Tech Ecosystems Data reigns supreme as the currency of innovation, and it is a valuable one at that. In the multifaceted world of technology, mastering the art of data engineering has become crucial for supporting billion-dollar tech ecosystems. This sophisticated craft involves creating and maintaining data infrastructures capable of handling vast amounts of information with high reliability and efficiency.
- Open Source: A Pathway To Personal and Professional Growth Open source can go beyond philanthropy: it’s a gateway to exponential learning, expanding your professional network, and propelling your software engineering career to the next level. In this article, I’ll explain why contributing to open-source projects is an excellent investment and share how to begin making your mark in the community.
- The Future Speaks: Real-Time AI Voice Agents With Ultra-Low Latency Voice mode has quickly become a flagship feature of conversational AI, putting users at ease and allowing them to interact in the most natural way — through speech. OpenAI has continually blazed trails with the introduction of real-time AI voice agen
Popular Topics
- Douyin level price list 1-75 Douyin level price comparison table: 1, level 1 = 0.5 yuan; level 2, 2 = 1 yuan; level 3, 3 = 2 yuan; level 4, 4 = 3 yuan; level 5, 5 = 5 yuan; 6, 6 Level = 7 yuan; Level 7 and 7 = 9 yuan; Level 8 and 8 = 13 yuan; Level 9 and 9 = 18 yuan; Level 10 and 10 = 24 yuan and so on. This topic provides you with relevant articles, downloads, and course content for you to download and experience for free.
- wifi shows no ip assigned Solution to wifi showing no IP allocation: 1. Restart the device and router, turn off the Wi-Fi connection on the device, turn off the device, turn off the router, wait a few minutes, then reopen the router to connect to wifi; 2. Check the router settings and restart DHCP, make sure the DHCP function is enabled; 3. Reset network settings, which will delete all saved WiFi networks and passwords. Please make sure they are backed up before performing this operation; 4. Update the router firmware, log in to the router management interface, and find the firmware Update options and follow the prompts. This topic provides you with various wifi-related articles, downloads and courses. I hope
- Virtual mobile phone number to receive verification code To receive the verification code with a virtual mobile phone number: first enter the Yima verification code receiving platform; then register as a website member; then open the SMS verification code service and select an operator; finally obtain the virtual mobile phone number, go to the platform where the verification code is to be sent, and put the phone Fill in the number and select [Send Verification Code].
- How to turn off windows security center Windows Security Center is a comprehensive security control panel for Windows systems, including firewall status prompts, anti-virus software status prompts, automatic update prompts and other basic system security information. So how to turn off Windows Security Center? I believe many friends are still not clear about it. PHP Chinese website has brought you relevant tutorials and tutorials. You are welcome to come and learn and read.
- myfreemp3 Myfreemp3 is a free online music search engine that allows users to search and play music directly on the web. Myfreemp3 has the advantages of fast search engine and rich resources. PHP Chinese website has compiled related articles and APP downloads about myfreemp3 for everyone. etc.
IntelligentAIQ&A
Ask
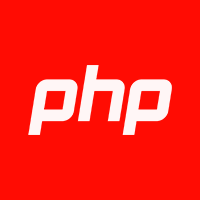
【Learning PHP】
PHP is a widely used open source multi-purpose scripting language. Compared with other technologies, PHP itself is open source and free; the program can be embedded in HTML for execution, and the execution efficiency is much higher than CGI that completely generates HTML tags. It runs on the server side, consumes very few system resources, has strong cross-platform and high efficiency features, and PHP supports almost all popular databases and operating systems. The most important thing is that PHP can also use C and C for program expansion. , PHP language has a very wide range of applications in WEB development. In addition, object-oriented has been greatly improved in PHP4 and PHP5, and can also be used to develop large-scale commercial programs.
- php array_diff_assoc() function
- php array_merge_recursive() function
- TCP/IP mail
- TCP/IP protocol
- TCP/IP addressing
- Web Forms - Database Connection
- Web Forms - ArrayList object
- Web Forms - Button control
- Web Forms - TextBox control
- Web Forms - HTML forms
- Web Forms - Events
- ionic Toggle
- ionic forms and input boxes
- ionic list
- ionic button
- ionic bottom
- ionic head
- MVC - Model
- MVC-SQL Database
- MVC - View
-
- IntermediateThe latest ThinkPHP 5.1 world premiere video tutorial (60 days to become a PHP expert online training course)
-
1404160 times of learning
- Free
-
- ElementaryPHP introductory tutorial one: Learn PHP in one week
-
4233211 times of learning
- Free
-
- ElementaryPHP complete self-study manual
-
2284295 times of learning
- Free
-
- IntermediateDugu Jiujian (4)_PHP video tutorial
-
1217209 times of learning
- Free
- Hot
- New
- Follow
- web3.0
- Backend Development
- Web Front-end
- Database
- Operation and Maintenance
- Development Tools
- PHP Framework
- Daily Programming
- WeChat Applet
- Common Problem
- Other
- Tech
- CMS Tutorial
- Java
- System Tutorial
- Computer Tutorials
- Hardware Tutorial
- Mobile Tutorial
- Software Tutorial
- Mobile Game Tutorial
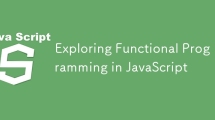
Exploring Functional Programming in JavaScript
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. It avoids changing state and mutable data. The fundamental idea is to build programs using pur
2024-09-30 22:33:02
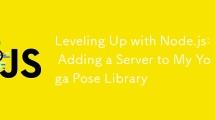
Leveling Up with Node.js: Adding a Server to My Yoga Pose Library
Leveling Up with Node.js: Adding a Server to My Yoga Pose Library
After getting comfortable with React through building a simple Yoga Pose Library, I wanted to take my project to the next level by adding some backend functionality. That’s where
2024-09-30 14:28:21
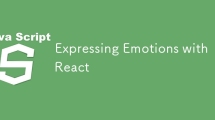
Expressing Emotions with React
In React, handling emotions or UI components related to emotions (like facial expressions, emotional states, or user feedback) can be achieved through a combination of state management, conditional rendering, and animations.
Here’s a basic outline o
2024-09-30 14:27:03
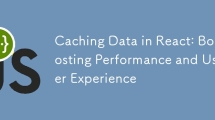
Caching Data in React: Boosting Performance and User Experience
Caching data in React can significantly improve performance and user experience by reducing the need to fetch the same data multiple times. Here are several approaches to implement data caching in React:
1. Using State Management Libraries
2024-09-30 14:23:03
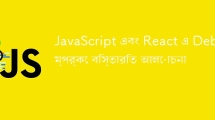
Detailed discussion on using Debounce in JavaScript and React
In JavaScript and React, debounce is a technique that quickly stops the iteration of a function and executes the function after a certain amount of time. It is mainly used to improve performance, especially when the user performs tasks such as typing or scrolling.
Debounce
2024-09-30 12:38:02
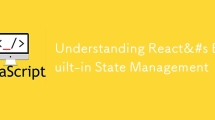
Understanding Reacts Built-in State Management
React's built-in state management relies on the useState and useReducer hooks to manage state within components. Here's a breakdown:
useState:
This hook is used for managing local component state. It returns an array with two elements: the curr
2024-09-30 12:36:02
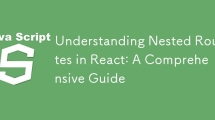
Understanding Nested Routes in React: A Comprehensive Guide
In React, nested routes allow you to structure your routes hierarchically, where one route is nested inside another. This is useful when building complex UIs where certain components or pages are shared across different routes.
To create nested rout
2024-09-30 12:35:02
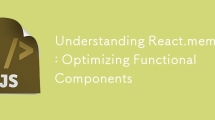
Understanding React.memo: Optimizing Functional Components
React.memo is a higher-order component used in React to optimize performance by preventing unnecessary re-renders of functional components. It works by memoizing the result of a component and only re-rendering it if its props change. This is useful f
2024-09-30 12:33:02
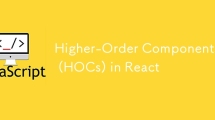
Higher-Order Components (HOCs) in React
Higher-Order Components (HOCs) in React are functions that take a component and return a new component with enhanced functionality. They allow you to reuse logic across multiple components without duplicating code.
Here's a basic example of a HOC:
2024-09-30 12:32:02
More
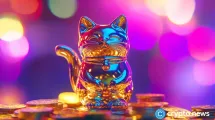
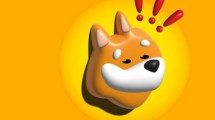
Bonk coin trading platform recommendation
Recommendations for the best Bonk currency trading platform: 1.Raydium: high liquidity, low fees; 2.Orca: rich trading pairs, friendly interface; 3.FTX: high liquidity and security; 4.Gate.io: currency selection Wide range, low fees; 5. HuobiGlobal: supports many currencies and provides leveraged transactions.
2024-09-30 22:37:31
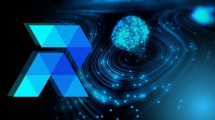
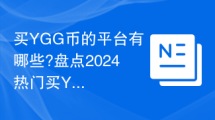
What are the platforms to buy YGG coins?
YGG coins can be purchased through the following platforms: Centralized exchanges: Binance, Huobi, OKX, Gate.io, Kraken Decentralized exchanges: Uniswap, SushiSwap, PancakeSwap, 1inchExchange Other platforms: YGG coin official website, ImmutableX
2024-09-30 22:34:01
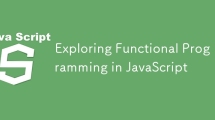
Exploring Functional Programming in JavaScript
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. It avoids changing state and mutable data. The fundamental idea is to build programs using pur
2024-09-30 22:33:02
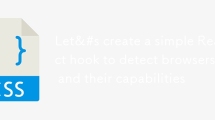
Lets create a simple React hook to detect browsers and their capabilities
User agent sniffing is the most popular approach for browser detection. Unfortunately, it's not very accessible for a front end development because of multiple reasons. Browser vendors constantly trying to make sniffing not possible. Thus, each brows
2024-09-30 22:18:02
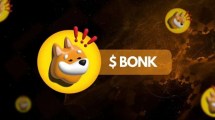
How to stake Bonk coins?
Bonk coins can be staked through the following steps: 1. Obtain and connect to a staking platform, such as the official Bonk website or Solana wallet. 2. Choose a staking pool. 3. Pledge Bonk coins. 4. Receive staking rewards and consider reinvesting regularly to maximize returns.
2024-09-30 22:13:02
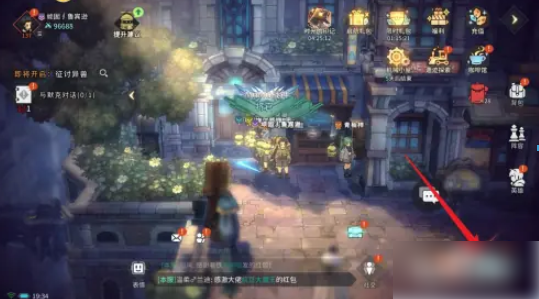
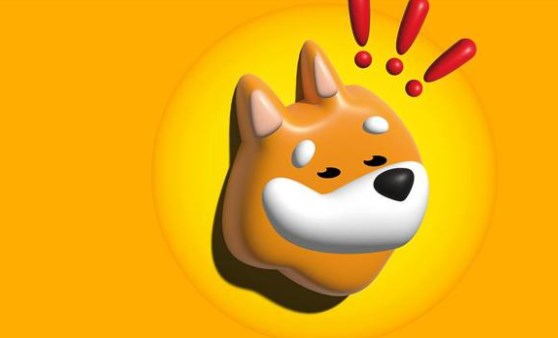
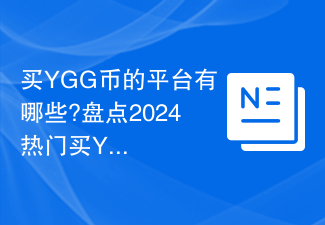
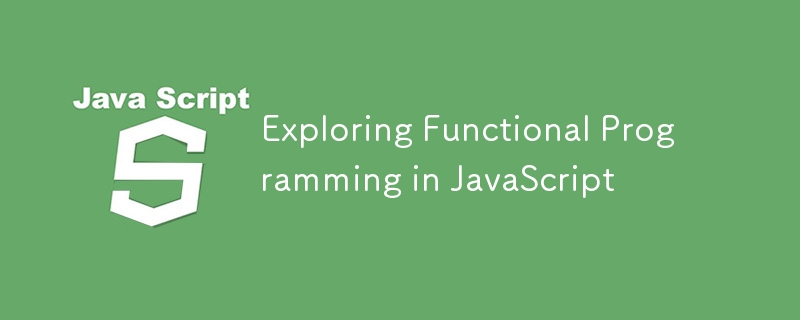
MoreArticle
![[Web front-end] Node.js quick start](https://img.php.cn/upload/course/000/000/067/662b5d34ba7c0227.png?x-oss-process=image/resize,m_fill,h_134,w_220)
- Elementary[Web front-end] Node.js quick start
-
4814
- Free
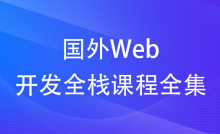
- ElementaryComplete collection of foreign web development full-stack courses
-
3726
- Free
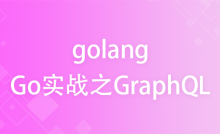
- ElementaryGo language practical GraphQL
-
3205
- Free
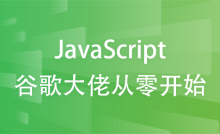
- Elementary550W fan master learns JavaScript from scratch step by step
-
566
- Free
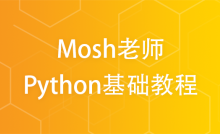
- ElementaryPython master Mosh, a beginner with zero basic knowledge can get started in 6 hours
-
16412
- Free
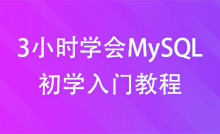
- ElementaryGetting Started with MySQL (Teacher mosh)
-
1162
- Free
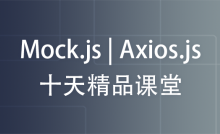
- ElementaryMock.js | Axios.js | Json | Ajax--Ten days of quality class
-
2215
- Free
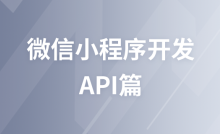
- ElementaryWeChat Mini Program Development API Chapter
-
5687
- Free
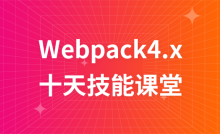
- ElementaryWebpack4.x---Ten-day skills class
-
6875
- Free
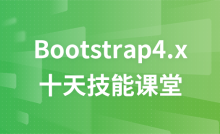
- ElementaryBootstrap4.x---Ten days of quality class
-
7886
- Free
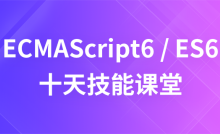
- ElementaryECMAScript6/ES6---Ten-day skills class
-
8420
- Free
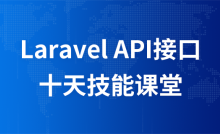
- ElementaryLaravel---API interface
-
3000
- Free
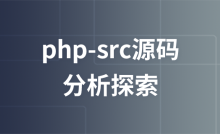
- Elementaryphp-src source code analysis and exploration
-
1930
- Free
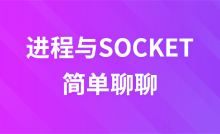
- ElementaryProcesses and SOCKETs
-
1592
- Free
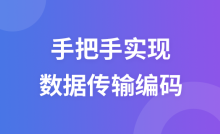
- ElementaryStep by step implementation of data transmission encoding
-
478
- Free
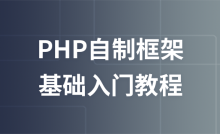
- ElementaryPHP self-made framework
-
3685
- Free
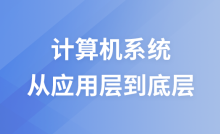
- ElementaryComputer system from application layer to bottom layer
-
2132
- Free
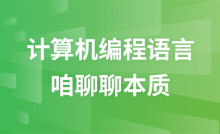
- ElementaryBriefly talk about the nature of computer programming languages
-
368
- Free
-
0 Like0 answer1438 Views
-
0 Like0 answer1597 Views
-
0 Like0 answer1369 Views
-
0 Like0 answer1289 Views
-
0 Like0 answer1342 Views
-
0 Like0 answer4692 Views
-
1 Like1 answer1430 ViewsI have a URL parameter that contains the category ID and I want to treat it as an array like this: http://example.com?cat[]=3,9,13 In PHP I use Getting the array: $catIDs=$_GET['cat']; When I do echogettype($catIDs); it shows that it is actually treated as an array, but when I do print_r($catIDs); I get the following Result: Array([0]=>3,9,13)
P粉785905797 2024-04-06 22:09 Ask
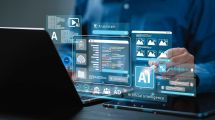
Check Out These 6 Lesser-Known AI Apps That Provide Unique Experiences
At this point, most folks have heard of ChatGPT and Copilot, two pioneering generative AI apps that have led the AI boom.But did you know that heaps of lesser-known AI tools can deliver wonderful, unique experiences? Here are six of the best. 1 D
2024-09-25 21:56:42
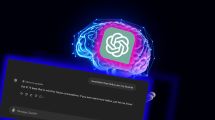
This ChatGPT Feature Helps Me Get Personalized Results for Every Query
When I first encountered ChatGPT's Memories feature, I wasn’t sure what to expect. But after using it for a while, I’ve realized the world of difference it makes in the quality of responses I get. It’s like having a digital assistant that not only li
2024-09-25 21:13:22
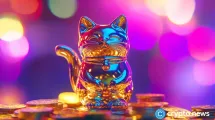
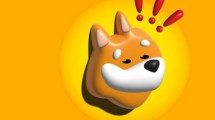
Bonk coin trading platform recommendation
Recommendations for the best Bonk currency trading platform: 1.Raydium: high liquidity, low fees; 2.Orca: rich trading pairs, friendly interface; 3.FTX: high liquidity and security; 4.Gate.io: currency selection Wide range, low fees; 5. HuobiGlobal: supports many currencies and provides leveraged transactions.
2024-09-30 22:37:31
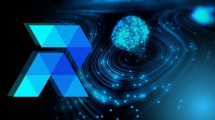
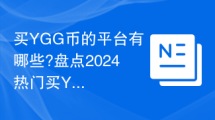
What are the platforms to buy YGG coins?
YGG coins can be purchased through the following platforms: Centralized exchanges: Binance, Huobi, OKX, Gate.io, Kraken Decentralized exchanges: Uniswap, SushiSwap, PancakeSwap, 1inchExchange Other platforms: YGG coin official website, ImmutableX
2024-09-30 22:34:01
-
-
WIKINDX Reference management, document management, citations and more. Designed by scholars for scholars, continuously developed since 2003 and used by individuals and major research institutions worldwide, WIKINDX is a virtual research environment (enhanced online literature manager) that stores searchable references, notes, documents, Quotes, thoughts, etc. The integrated WYSIWYG word processor exports formatted articles to RTF and HTML. Plug-ins include a citation style editor and import/export of references (BibTeX, Endnote, RIS, etc.). WIKINDX supports every reference text
-
-
phpList phpList provides open source email marketing services, including analysis, list segmentation, content personalization and bounce processing. The rich technical functions and secure and stable code base are the result of 17 years of continuous development. Used in 95 countries, available in more than 20 languages, and used in 25 billion email campaigns sent last year. You can deploy it with your own SMTP server or get a free hosting account at http://phplist.com.
-
SecLists SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
-
-