在Java中,Scanner是一個類,用於取得字串和不同原始類型(例如int、double等)的輸入。 scanner類別位於java.util.concurrent套件中。它擴展了 Object 類別並實作了 Closeable 和 Iterator 介面。在空格分隔符號的幫助下,輸入被分成類別。 Java 中 Scanner 類別的聲明、語法、工作和其他幾個方面將在以下部分中討論。
開始您的免費軟體開發課程
網頁開發、程式語言、軟體測試及其他
Java 掃描器類別聲明
Java Scanner 類別聲明如下。
public final class Scanner extends Object implements Iterator<String>
登入後複製
文法:
Scanner 類別可以在 Java 程式中按以下語法使用。
Scanner sc = new Scanner(System.in);
登入後複製
這裡,sc 是掃描器對象,System.in 告訴 Java 輸入將會是這個。
Java Scanner 類別如何運作?
現在,讓我們看看 Scanner 類別是如何運作的。
- 導入類別 java.util.Scanner 或整個套件 java.util。
import java.util.*;
import java.util.Scanner;
登入後複製
Scanner s = new Scanner(System.in);
登入後複製
int n = sc.nextInt();
登入後複製
建構子
Java中的掃描器類別有不同的建構子。他們是:
-
掃描器(文件來源): 將建構一個新的掃描儀,從上述文件產生值。
-
Scanner(File src, String charsetName): 將建構一個新的掃描器,產生從上述檔案掃描的值。
-
Scanner(InputStream src): 將建構一個新的掃描器,從上述輸入流產生值。
-
Scanner(InputStream src, String charsetName): 將建構一個新的掃描器,從上述輸入流產生值。
-
掃描器(路徑 src): 將建構一個新的掃描儀,從上述檔案產生值。
-
Scanner(Path src, String charsetName): 將建構一個新的掃描器,從上述檔案產生值。
-
掃描器(可讀源): 將建立一個新的掃描儀,從上述來源產生值。
-
Scanner(ReadableByteChannel src): 將建立一個新的掃描器,從上述通道產生值。
-
Scanner(ReadableByteChannel src, String charsetName): 將建構一個新的掃描器,從上述通道產生值。
-
Scanner(String src): 將建構一個新的掃描器,從上述字串產生值。
Java Scanner 類別的方法
以下是執行不同功能的方法。
-
close(): Scanner gets closed on calling this method.
-
findInLine(Pattern p): Next occurrence of the mentioned pattern p will be attempted to find out, ignoring the delimiters.
-
findInLine(String p): The next occurrence of the pattern that is made from the string will be attempted to find out, ignoring the delimiters.
-
delimiter(): Pattern that is currently used by the scanner to match delimiters will be returned.
-
findInLine(String p): The next occurrence of the pattern that is made from the string will be attempted to find out, ignoring the delimiters.
-
findWithinHorizon(Pattern p, int horizon): Tries to identify the mentioned pattern’s next occurrence.
-
hasNext(): If the scanner has another token in the input, true will be returned.
-
findWithinHorizon(String p, int horizon): Tries to identify the next occurrence of the mentioned pattern made from the string p, ignoring delimiters.
-
hasNext(Pattern p): If the next token matches the mentioned pattern p, true will be returned.
-
hasNext(String p): If the next token matches the mentioned pattern p made from the string p, true will be returned.
-
next(Pattern p): Next token will be returned, which matches the mentioned pattern p.
-
next(String p): Next token will be returned, which matches the mentioned pattern p made from the string p.
-
nextBigDecimal(): Input’s next token will be scanned as a BigDecimal.
-
nextBigInteger(): Input’s next token will be scanned as a BigInteger.
-
nextBigInteger(int rad): Input’s next token will be scanned as a BigInteger.
-
nextBoolean(): Input’s next token will be scanned as a Boolean, and it will be returned.
-
nextByte(): Input’s next token will be scanned as a byte.
-
nextByte(int rad): Input’s next token will be scanned as a byte.
-
nextDouble(): Input’s next token will be scanned as a double.
-
nextFloat(): Input’s next token will be scanned as a float.
-
nextInt(): Input’s next token will be scanned as an integer.
-
nextInt(int rad): Input’s next token will be scanned as an integer.
-
nextLong(int rad): Input’s next token will be scanned as a long.
-
nextLong(): Input’s next token will be scanned as a long.
-
nextShort(int rad): Input’s next token will be scanned as a short.
-
nextShort(): Input’s next token will be scanned as a short.
-
radix(): The default radix of the scanner will be returned.
-
useDelimiter(Pattern p): Delimiting pattern of the scanner will be set to the mentioned pattern.
-
useDelimiter(String p): Delimiting pattern of the scanner will be set to the mentioned pattern made from the string p.
-
useRadix(int rad): The scanner’s default radix will be set to the mentioned radix.
-
useLocale(Locale loc): The locale of the scanner will be set to the mentioned locale.
Examples of Java Scanner Class
Now, let us see some sample programs of the Scanner class in Java.
Example #1
Code:
import java.util.Scanner;
class ScannerExample {
public static void main(String[] args) {
//create object of scanner
Scanner sc = new Scanner(System.in);
System.out.println("Enter the username");
String obj = sc.nextLine();
System.out.println("The name you entered is: " + obj);
}
}
登入後複製
Output:
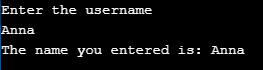
On executing the program, the user will be asked to enter a name. As the input is a string, the nextLine() method will be used. Once it is entered, a line will be printed displaying the name user given as input.
Example #2
Code:
import java.util.Scanner;
class ScannerExample {
public static void main(String[] args) {
//create object of scanner
Scanner sc = new Scanner(System.in);
System.out.println("Enter age");
int obj = sc.nextInt();
System.out.println("The age you entered is: " + obj);
}
}
登入後複製
Output:
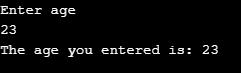
On executing the program, the user will be asked to enter the age. As the input is a number, the nextInt() method will be used. Once it is entered, a line will be printed displaying the age user given as input.
以上是Java 掃描器類的詳細內容。更多資訊請關注PHP中文網其他相關文章!