JavaScript 於 1995 年引入,用於在 Netscape Navigator 瀏覽器中的網頁新增程式。此後,該語言已被所有其他主要圖形 Web 瀏覽器採用。它使現代 Web 應用程式成為可能,您可以直接與之交互,而無需為每個操作重新載入頁面。 JavaScript 也被用在較傳統的網站中,以提供各種形式的互動性和智慧性。
Javascript 是一種跨平台、物件導向的腳本語言,用於使網頁具有互動性(例如,具有複雜的動畫、可點擊的按鈕等)。還有更高級的伺服器端版本的 javascript,例如 Node js,它允許您為網站添加比下載檔案更多的功能。
JavaScript 是最受歡迎的語言之一,在 Web 開發方麵包含許多功能。根據 Github 的數據,它是頂級程式語言之一,我們必須正確了解 JavaScript 的功能才能了解它的功能。
1.輕量級腳本語言
2.動態打字
3.物件導向程式支援
4.功能風格
5.平台無關
6.基於原型
讓我們詳細了解所有這些功能,以便您從頭到尾都可以了解它們
1.輕量級腳本語言
JavaScript 是一種輕量級腳本語言,因為它僅用於瀏覽器中的資料處理。由於它不是通用語言,因此它的庫集有限。此外,由於它僅適用於客戶端執行以及 Web 應用程序,因此 JavaScript 的輕量級特性是一個很棒的功能。
2.動態打字
JavaScript 支援動態類型,這表示變數的類型是根據儲存的值定義的。例如,如果宣告變數 x,則可以儲存字串或數字類型值、陣列或物件。這稱為動態類型。
3.物件導向程式支援
從ES6開始,類別和OOP的概念更加細緻。此外,在 JavaScript 中,JavaScript 中 OOP 的兩個重要原則是物件建立模式(封裝)和程式碼重用模式(繼承)。雖然 JavaScript 開發者很少使用這個功能,但大家可以去探索一下。
3.功能風格
這意味著 JavaScript 使用函數式方法,甚至物件也是從建構函式創建的,而每個建構函式代表一個唯一的物件類型。此外,JavaScript 中的函數可以用作對象,也可以傳遞給其他函數。
4.平台獨立
這意味著 JavaScript 是平台無關的,或者我們可以說它是可移植的;這僅僅意味著您只需編寫一次腳本即可隨時隨地運行它。一般來說,您可以編寫 JavaScript 應用程式並在任何平台或任何瀏覽器上運行它們,而不會影響腳本的輸出。
5.基於原型的語言
JavaScript 是一種基於原型的腳本語言。這意味著 JavaScript 使用原型而不是類別或繼承。在 Java 等語言中,我們建立一個類,然後為這些類別建立物件。但在 JavaScript 中,我們定義了一個物件原型,然後可以使用這個物件原型創建更多物件。
7.翻譯語言
JavaScript 是一種解釋性語言,這意味著在 javascript 中編寫的腳本是逐行處理的。這些腳本由 JavaScript 解譯器解釋,JavaScript 解譯器是 Web 瀏覽器的內建元件。但現在瀏覽器中的許多 JavaScript 引擎(例如 Chrome 中的 V8 引擎)都使用 JavaScript 程式碼的即時編譯。
8.非同步處理
JavaScript 支援 Promise,它可以實現非同步請求,其中發起請求並且 JavaScript 不必等待回應,這有時會阻止請求處理。同樣從 ES8 開始,JavaScript 中也支援了非同步函數,這些函數不會逐一執行,而是並行處理,這對處理時間有正面的影響,很大程度上減少了處理時間。
值得注意的是 Java 和 JavaScript 之間的核心差異。
Javascript 和 Java 在某些方面相似,但在其他方面卻有根本不同。
Javascript is a free-form language compared to Java. You do not have to declare all variables, classes, and methods. Yo do not have to be concerned with whether methods are public, private, or protected, and you do not have to implement interfaces while Java is a class-based programming language designed for fast execution and type safety meaning that you can’t cast a java integer into an object reference or access private memory by corrupting the java bytecode.
Es6(ECMAScript 2015) is a major update to javascript that includes a whole lot of new features with a focus on simplicity and readability.7
Let’s find out about these new features and when and how to use it
1. The let and const keyword
Before the introduction of Es6, the var keyword was the only way to declare variables in JavaScript. With the const keyword, you can declare a variable as a constant and as a constant, it will be read-only.
Copy code // Using let let age = 25; console.log(age); // Output: 25 age = 26; console.log(age); // Output: 26 // Using const const birthYear = 1998; console.log(birthYear); // Output: 1998
2. Arrow function
Here we use => instead of the function keyword. The arrow function makes our code more readable, clean and shorter.
// Traditional function function add(a, b) { return a + b; } // Arrow function equivalent const add = (a, b) => a + b; // Usage console.log(add(3, 5)); // Output: 8
3. Objects
Objects are simply collections of properties which are made up of key, value pairs.
// Define an object to represent a person const person = { firstName: "John", lastName: "Doe", age: 30, job: "Software Engineer", hobbies: ["Reading", "Coding", "Hiking"], };
4. Classes
The class keyword is used to formalize the pattern of simulating class-like inheritance hierarchies using functions and prototypes.
// Define a class called "Person" class Person { // Constructor method to initialize an object constructor(name, age) { this.name = name; this.age = age; }
5. Promises
This simply makes asynchronous calls easy and effortless.
// Function that returns a promise function fetchData() { return new Promise((resolve, reject) => { const success = true; // Simulate a successful operation setTimeout(() => { if (success) { resolve("Data fetched successfully!"); } else { reject("Error fetching data."); } }, 2000); // Simulate an async operation with a 2-second delay }); }
6. Template Literals
Template literals simply means having variables in a string.
// Variables const name = "Alice"; const age = 30; const job = "developer"; 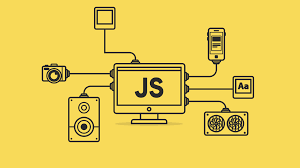 // Using Template Literals const introduction = `Hello, my name is ${name}. I am ${age} years old, and I work as a ${job}.`; console.log(introduction);
Thanks for reading, and always know that I’m rooting for you!!!!
以上是JavaScript 基本介紹的詳細內容。更多資訊請關注PHP中文網其他相關文章!