This article mainly introduces relevant information about the detailed example of converting Excel to shape file in java. I hope that through this article, everyone can realize such a function. Friends in need can refer to
Excel in java Detailed explanation of examples of converting shape files
Overview:
This article describes how to combine geotools and POI to convert Excel to shp, and then combine it with the previous shp The conversion to geojson data enables users to upload excel data and display it on the web.
Screenshot:
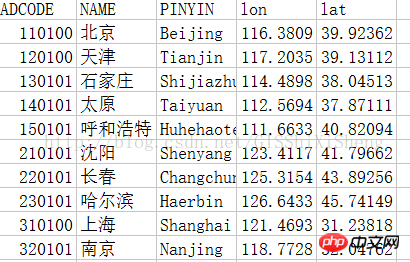
Original Excel file
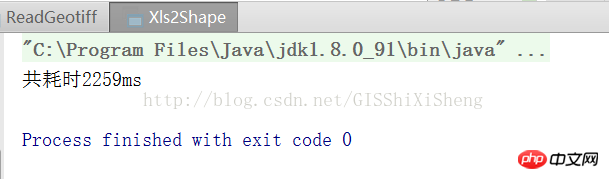
Time consuming to run
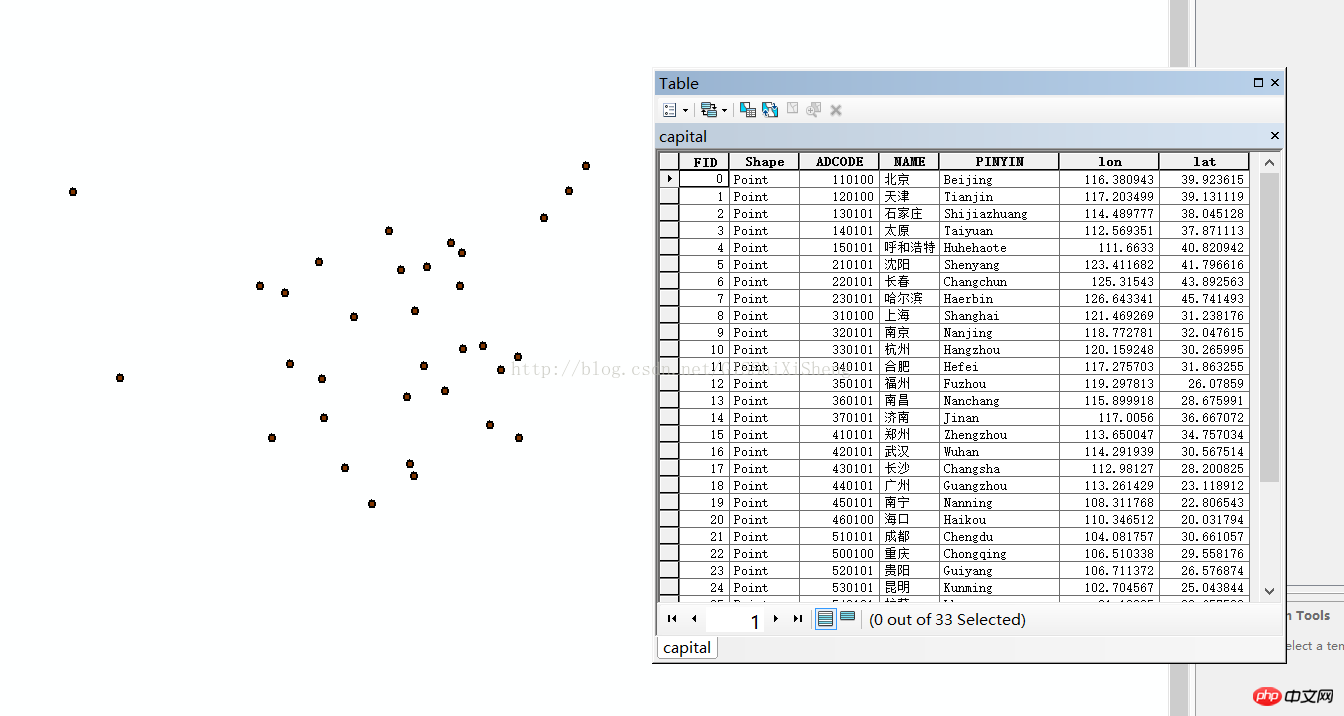
Running results
Code:
package com.lzugis.geotools;
import com.lzugis.CommonMethod;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.GeometryFactory;
import com.vividsolutions.jts.geom.Point;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.poifs.filesystem.POIFSFileSystem;
import org.geotools.data.FeatureWriter;
import org.geotools.data.Transaction;
import org.geotools.data.shapefile.ShapefileDataStore;
import org.geotools.data.shapefile.ShapefileDataStoreFactory;
import org.geotools.feature.simple.SimpleFeatureTypeBuilder;
import org.geotools.referencing.crs.DefaultGeographicCRS;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import java.io.File;
import java.io.FileInputStream;
import java.io.InputStream;
import java.io.Serializable;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Created by admin on 2017/9/6.
*/
public class Xls2Shape {
static Xls2Shape xls2Shp = new Xls2Shape();
private static String rootPath = System.getProperty("user.dir");
private CommonMethod cm = new CommonMethod();
private HSSFSheet sheet;
private Class getCellType(HSSFCell cell) {
if (cell.getCellType() == HSSFCell.CELL_TYPE_STRING) {
return String.class;
} else if (cell.getCellType() == HSSFCell.CELL_TYPE_NUMERIC) {
return Double.class;
} else {
return String.class;
}
}
private Object getCellValue(HSSFCell cell) {
if (cell.getCellType() == HSSFCell.CELL_TYPE_STRING) {
return cell.getRichStringCellValue().getString();
} else if (cell.getCellType() == HSSFCell.CELL_TYPE_NUMERIC) {
return cell.getNumericCellValue();
} else {
return "";
}
}
private List
Description:
1. The conversion is limited to the conversion of point objects;
2. Keep all excel-related attributes, lon and lat fields are required;
3. For Chinese fields, the first one is taken Letter processing;
The above is the detailed content of Code example of how to convert Excel to shape file in Java (picture). For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn