empty object mode
In the Null Object Pattern, a null object replaces the check for NULL object instances. Instead of checking for null values, Null objects reflect a relationship that does nothing. Such Null objects can also provide default behavior when data is not available.
In the empty object pattern, we create an abstract class that specifies various operations to be performed and an entity class that extends the class. We also create an empty object class that does not implement any implementation of the class. Object classes will be used seamlessly wherever null values need to be checked.
Implementation
We will create an AbstractCustomer abstract class that defines an operation (in this case, the name of the customer), and extend the AbstractCustomer class entity class. Factory class CustomerFactory returns a RealCustomer or NullCustomer object based on the name passed by the customer.
NullPatternDemo, our demo class uses CustomerFactory to demonstrate the use of the null object pattern.
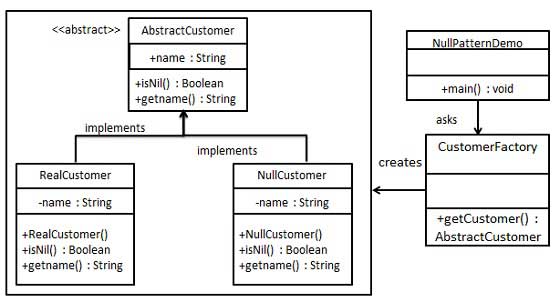
Step 1
Create an abstract class.
AbstractCustomer.java
public abstract class AbstractCustomer { protected String name; public abstract boolean isNil(); public abstract String getName(); }
Step 2
Create an entity class that extends the above class.
RealCustomer.java
public class RealCustomer extends AbstractCustomer { public RealCustomer(String name) { this.name = name; } @Override public String getName() { return name; } @Override public boolean isNil() { return false; } }
NullCustomer.java
public class NullCustomer extends AbstractCustomer { @Override public String getName() { return "Not Available in Customer Database"; } @Override public boolean isNil() { return true; } }
Step 3
Create CustomerFactory class.
CustomerFactory.java
public class CustomerFactory { public static final String[] names = {"Rob", "Joe", "Julie"}; public static AbstractCustomer getCustomer(String name){ for (int i = 0; i < names.length; i++) { if (names[i].equalsIgnoreCase(name)){ return new RealCustomer(name); } } return new NullCustomer(); } }
Step 4
Use CustomerFactory to get based on the name passed by the customer A RealCustomer or NullCustomer object.
NullPatternDemo.java
public class NullPatternDemo { public static void main(String[] args) { AbstractCustomer customer1 = CustomerFactory.getCustomer("Rob"); AbstractCustomer customer2 = CustomerFactory.getCustomer("Bob"); AbstractCustomer customer3 = CustomerFactory.getCustomer("Julie"); AbstractCustomer customer4 = CustomerFactory.getCustomer("Laura"); System.out.println("Customers"); System.out.println(customer1.getName()); System.out.println(customer2.getName()); System.out.println(customer3.getName()); System.out.println(customer4.getName()); } }
Step 5
Verify the output.
Customers Rob Not Available in Customer Database Julie Not Available in Customer Database