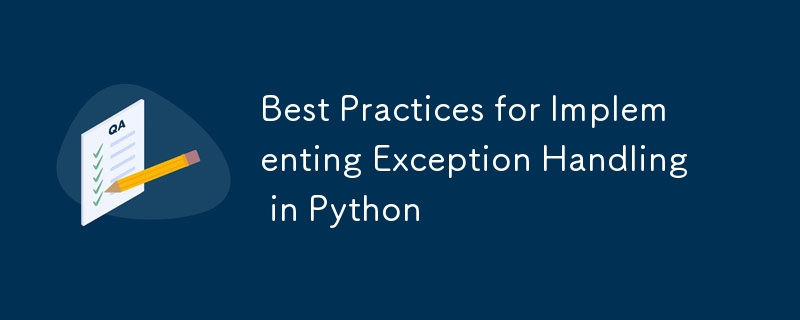
Writing effective exception handling code is essential for creating robust and maintainable applications.
Below are some best practices for writing exception handling code in Python:
1. Catch Only What You Can Handle
Be specific:
- Catch specific exceptions rather than using a broad except clause.
- This ensures that only the exceptions you expect and know how to handle are caught.
try: # Code that might raise an exception except ValueError as e: print(f"Value error occurred: {e}")
로그인 후 복사
2. Avoid Bare except: Clauses
Catch specific exceptions:
- Avoid using except: without specifying an exception type.
- This can catch unexpected errors and make debugging difficult.
try: # Code that might raise an exception except Exception as e: # Catch all exceptions if necessary print(f"An error occurred: {e}")
로그인 후 복사
3. Use try-except-else-finally Blocks
- try: Place the code that might raise an exception here.
- except: Handle exceptions in this block.
- else: Execute this block if no exception was raised in the try block.
- finally: Execute this block regardless of whether an exception was raised, often used for cleanup.
try: # Code that might raise an exception except ValueError as e: print(f"Value error: {e}") else: print("No exceptions occurred.") finally: print("This will always be executed.")
로그인 후 복사
4. Log Exceptions
- Use the logging module to log exceptions.
- Logging helps diagnose issues in production without revealing errors to end users.
import logging logging.basicConfig(level=logging.ERROR) try: # Code that might raise an exception except Exception as e: logging.error(f"An error occurred: {e}")
로그인 후 복사
5. Re-raise Exceptions When Necessary
- If you catch an exception but can't fully handle it, consider re-raising it so that it can be handled elsewhere.
try: # Code that might raise an exception except ValueError as e: logging.error(f"Value error: {e}") raise # Re-raise the exception
로그인 후 복사
6. Use Context Managers for Resource Management
- Use context managers (with statement) to manage resources like files, sockets, or database connections.
- This ensures that resources are properly released even if an exception is raised.
with open('file.txt', 'r') as file: content = file.read()
로그인 후 복사
7. Graceful Degradation
-Instead of allowing your application to crash, provide fallback mechanisms or user-friendly error messages.
- For example, if a configuration file is missing, you might use default settings instead.
try: with open('config.json', 'r') as file: config = json.load(file) except FileNotFoundError: print("Config file not found, using defaults.") config = {"default": "value"}
로그인 후 복사
8. Avoid Swallowing Exceptions
- Don't catch exceptions without taking any action.
- Ignoring exceptions can hide bugs and make the application behave unpredictably.
try: # Code that might raise an exception except Exception as e: pass # Bad practice - you're ignoring the error
로그인 후 복사
9. Document Exceptions
- Use docstrings to document the exceptions that your functions can raise.
- This helps other developers understand what exceptions to expect and how to handle them.
def divide(a, b): """ Divides two numbers. :param a: Numerator. :param b: Denominator. :return: The result of the division. :raises ZeroDivisionError: If the denominator is zero. """ if b == 0: raise ZeroDivisionError("Cannot divide by zero.") return a / b
로그인 후 복사
10. Use Custom Exceptions When Appropriate
- Create custom exceptions to represent specific error conditions in your application.
- This can make your code more readable and easier to maintain.
class InvalidInputError(Exception): """Exception raised for invalid inputs.""" pass def process_input(value): if not isinstance(value, int): raise InvalidInputError("Input must be an integer.") return value * 2
로그인 후 복사
11. Test Exception Handling
- Write tests to ensure that your exception handling works as expected.
- Use frameworks like unittest or pytest to test both normal and exceptional cases.
def test_divide(): assert divide(10, 2) == 5 with pytest.raises(ZeroDivisionError): divide(10, 0)
로그인 후 복사
12. Avoid Overusing Exceptions
Use exceptions for exceptional cases:
- Exceptions should be used for unexpected conditions, not as a regular control flow mechanism.
- For example, avoid using exceptions to handle predictable conditions like the end of a loop.
# Bad practice: using exceptions for control flow try: while True: value = next(iterator) except StopIteration: pass # End of iteration
로그인 후 복사
13. Chain Exceptions for Context
- Python allows you to chain exceptions to preserve the original context when raising a new exception.
- Use from to link related exceptions.
try: result = process_input(input_value) except InvalidInputError as e: raise ValueError("Failed to process input") from e
로그인 후 복사
By following these best practices, you can write more robust, maintainable, and readable exception handling code that gracefully manages errors and enhances your application's reliability.
Resoures
- Python's Official Documentation on Exception Handling
- Python's logging Module Documentation
- PEP 8 - Style Guide for Python Code (for general coding practices including exceptions)
- Real Python - Exception Handling
위 내용은 Python에서 예외 처리를 구현하는 모범 사례의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!