Spring Boot チートシート
Spring Boot チートシート
注釈
Annotation | Description | Example |
---|---|---|
@SpringBootApplication | Marks a class as a Spring Boot application. Enables auto-configuration and component scanning. | @SpringBootApplication |
@RestController | Indicates that a class provides RESTful endpoints. It combines @Controller and @ResponseBody annotations. | @RestController |
@RequestMapping | Maps HTTP requests to handler methods of RESTful controllers. | @RequestMapping("/api") |
@Autowired | Injects dependencies into a Spring bean. It can be used for constructor, setter, or field injection. | @Autowired private MyService myService; |
@Component | Indicates that a class is a Spring-managed component. It is automatically detected during component scanning. | @Component |
@Repository | Marks a class as a Spring Data repository. It handles data access and persistence logic. | @Repository |
@Service | Marks a class as a service component in the business layer. It is used to separate business logic from presentation logic. | @Service |
@Configuration | Indicates that a class provides bean definitions. It is used along with @bean to define beans in Java-based configuration. | @Configuration |
@Value | Injects values from properties files or environment variables into Spring beans. | @Value("${my.property}") private String property; |
制御フロー
- 初期化: Spring Boot は、アプリケーションのプロパティをロードし、Bean を自動構成することによって開始されます。
- コンポーネント スキャン: Spring は、コントローラー、サービス、リポジトリなどのコンポーネントをスキャンします。
- Bean の作成: Spring は Bean を作成し、依存関係注入を使用して依存関係を解決します。
- リクエスト処理: 受信 HTTP リクエストは、リクエスト マッピングに基づいてコントローラー メソッドにマッピングされます。
- 実行: コントローラー メソッドはリクエストを処理し、サービスと対話し、応答を返します。
- レスポンスレンダリング: Spring はメソッドの戻り値を適切な HTTP レスポンス (JSON など) に変換します。
推奨されるフォルダー構成
ソース
§──メイン
│ §── ジャワ
│ │ └─ com
│ │ └─ 例
│ │ §── コントローラー
│ │ │ └── MyController.java
│ │ §── サービス
│ │ │ └── MyService.java
│ │ └─ Application.java
│ └── 資源
│ §── application.properties
│ └── テンプレート
│ └──index.html
└── テスト
━─ ジャワ
━──com
└── 例
└── コントローラー
━── MyControllerTest.java
Spring Boot チートシートでのエラー処理
エラー処理は、堅牢なアプリケーションを構築する上で重要な側面です。 Spring Boot は、エラーと例外を適切に処理し、スムーズなユーザー エクスペリエンスを保証するためのいくつかのメカニズムを提供します。
エラーの種類
- クライアント エラー: 400 Bad Request や 404 Not Found など、無効なクライアント リクエストによって引き起こされるエラー。
- サーバー エラー: 500 Internal Server Error など、サーバー側で発生するエラー。
- 検証エラー: 無効な入力またはデータ検証の失敗によるエラー。
エラー処理メカニズム
1. コントローラーのアドバイス
- @ControllerAdvice: Spring MVC でグローバル例外ハンドラーを定義するために使用されるアノテーション。
- @ExceptionHandler: コントローラー アドバイス内の特定の例外を処理するために使用されるアノテーション。
- 例:
@ControllerAdvice public class GlobalExceptionHandler { @ExceptionHandler(MyException.class) public ResponseEntity<ErrorResponse> handleMyException(MyException ex) { ErrorResponse response = new ErrorResponse("My error message"); return new ResponseEntity<>(response, HttpStatus.BAD_REQUEST); } @ExceptionHandler(Exception.class) public ResponseEntity<ErrorResponse> handleException(Exception ex) { ErrorResponse response = new ErrorResponse("Internal server error"); return new ResponseEntity<>(response, HttpStatus.INTERNAL_SERVER_ERROR); } }
応答エンティティ例外ハンドラ
- ResponseEntityExceptionHandler: 一般的な Spring MVC 例外を処理するための基本クラス。
- オーバーライド: handleMethodArgumentNotValid、handleHttpMessageNotReadable などのメソッドをオーバーライドします。
- 例:
@ControllerAdvice public class CustomExceptionHandler extends ResponseEntityExceptionHandler { @Override protected ResponseEntity<Object> handleMethodArgumentNotValid( MethodArgumentNotValidException ex, HttpHeaders headers, HttpStatus status, WebRequest request) { ErrorResponse response = new ErrorResponse("Validation failed"); return new ResponseEntity<>(response, HttpStatus.BAD_REQUEST); } // Other overrides for handling specific exceptions
3. エラーの属性
- ErrorAttributes: エラー応答の内容と形式をカスタマイズするために使用されるインターフェイス。
- DefaultErrorAttributes: Spring Boot によって提供されるデフォルトの実装。
@Component public class CustomErrorAttributes extends DefaultErrorAttributes { @Override public Map<String, Object> getErrorAttributes(WebRequest webRequest, boolean includeStackTrace) { Map<String, Object> errorAttributes = super.getErrorAttributes(webRequest, includeStackTrace); errorAttributes.put("customAttribute", "Custom value"); return errorAttributes; } }
プロパティファイルの使用
- application.properties: サーバー ポート、データベース URL などのアプリケーション全体のプロパティを保存します。
- カスタム プロパティ ファイル: さまざまな環境のカスタム プロパティ ファイルを定義します (例: application-dev.properties)。
- プロパティへのアクセス: @Value アノテーションまたは Spring の環境 API を使用してプロパティにアクセスします。
建築プロジェクト
- Maven: mvn clean install を実行してプロジェクトをビルドし、実行可能 JAR を生成します。
- Gradle: ./gradlew build を実行してプロジェクトをビルドし、実行可能 JAR を生成します。
追加のトピック
- Spring Boot スターター: スターターを使用して、プロジェクトに依存関係と自動構成を追加します。
- ロギング: Logback、Log4j、または Java Util Logging を使用してロギングを構成します。
- エラー処理: @ControllerAdvice を使用してグローバル例外処理を実装します。
- テスト: JUnit、Mockito、SpringBootTest を使用して単体テストと統合テストを作成します。
以上がSpring Boot チートシートの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undress AI Tool
脱衣画像を無料で

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Clothoff.io
AI衣類リムーバー

Video Face Swap
完全無料の AI 顔交換ツールを使用して、あらゆるビデオの顔を簡単に交換できます。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)
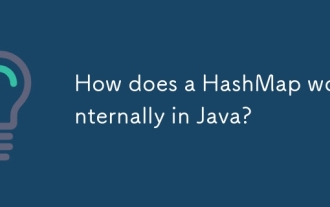
HashMapは、Javaのハッシュテーブルを介してキーと値のペアストレージを実装し、そのコアはデータの位置をすばやく配置することにあります。 1.最初にキーのHashCode()メソッドを使用して、ハッシュ値を生成し、ビット操作を介して配列インデックスに変換します。 2。異なるオブジェクトは、同じハッシュ値を生成し、競合をもたらす場合があります。この時点で、ノードはリンクされたリストの形式で取り付けられています。 JDK8の後、リンクされたリストが長すぎ(デフォルトの長さ8)、効率を改善するために赤と黒の木に変換されます。 3.カスタムクラスをキーとして使用する場合、equals()およびhashcode()メソッドを書き直す必要があります。 4。ハッシュマップは容量を動的に拡大します。要素の数が容量を超え、負荷係数(デフォルト0.75)を掛けた場合、拡張して再ハッシュします。 5。ハッシュマップはスレッドセーフではなく、マルチスレッドでconcuを使用する必要があります
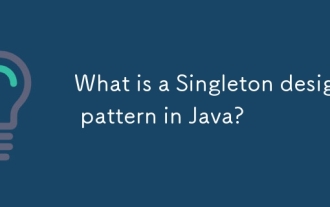
JavaのSingleton Design Patternは、クラスに1つのインスタンスしかないことを保証し、プライベートコンストラクターと静的方法を介したグローバルアクセスポイントを提供することを保証します。これは、共有リソースへのアクセスを制御するのに適しています。実装方法には以下が含まれます。1。レイジーロード、つまり、インスタンスは最初のリクエストが要求されたときにのみ作成されます。これは、リソースの消費が高く、必ずしも必要ではない状況に適しています。 2。スレッドセーフ処理。同期方法または再確認ロックを介して、マルチスレッド環境で1つのインスタンスのみが作成され、パフォーマンスへの影響が低下するようにします。 3.クラスの読み込み中にインスタンスを直接初期化するHungry Loadingは、事前に初期化できる軽量オブジェクトまたはシナリオに適しています。 4.列挙の実装は、Java列挙を使用してシリアル化、スレッドの安全性をサポートし、反射攻撃を防止することは、推奨される簡潔で信頼できる方法です。特定のニーズに応じて、さまざまな実装方法を選択できます
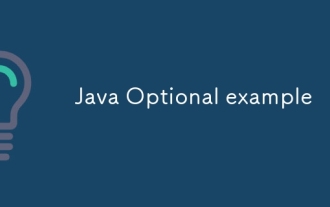
オプションは、意図を明確に表現し、ヌルの判断のコードノイズを減らすことができます。 1. optional.ofnullableは、nullオブジェクトに対処する一般的な方法です。たとえば、マップから値を取得する場合、Orelseを使用してデフォルト値を提供できるため、ロジックはより明確かつ簡潔になります。 2.チェーンコールマップを使用してネストされた値を達成してNPEを安全に回避し、リンクが無効である場合はデフォルト値を返す場合は自動的に終了します。 3.フィルターは条件付きフィルタリングに使用でき、その後の操作は条件が満たされた場合にのみ実行され続けます。そうしないと、軽量のビジネス判断に適したOrelseに直接ジャンプします。 4.基本的なタイプや単純なロジックなど、複雑さを高めるなど、オプションを過剰使用することはお勧めしません。一部のシナリオはNUに直接戻ります。
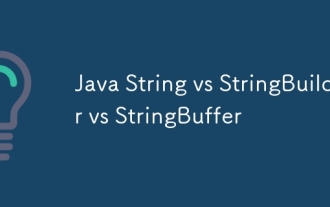
文字列は不変、StringBuilderは可変で非スレッドセーフ、StringBufferはMutableとThread-Safeです。 1.文字列のコンテンツが作成されると、少量のスプライシングに適しています。 2。StringBuilderは、単一のスレッドの頻繁なスプライシングに適しており、パフォーマンスが高くなっています。 3。StringBufferは、マルチスレッドの共有シナリオに適していますが、パフォーマンスがわずかに低くなっています。 4.初期容量を合理的に設定し、ループで文字列スプライシングを使用することはパフォーマンスを向上させることができます。
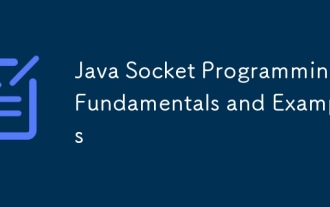
Javasocketプログラミングはネットワーク通信の基礎であり、クライアントとサーバー間のデータ交換はソケットを介して実現されます。 1。Javaのソケットは、クライアントが使用するソケットクラスとサーバーが使用するサーバーソケットクラスに分割されます。 2。ソケットプログラムを作成するときは、最初にサーバーリスニングポートを起動してから、クライアントによる接続を開始する必要があります。 3.コミュニケーションプロセスには、接続の確立、データの読み取りと書き込み、ストリームの閉鎖が含まれます。 4.注意事項には、ポート競合の避け、IPアドレスの正確な構成、合理的に閉じるリソース、複数のクライアントのサポートが含まれます。これらをマスターすると、基本的なネットワーク通信機能が実現できます。
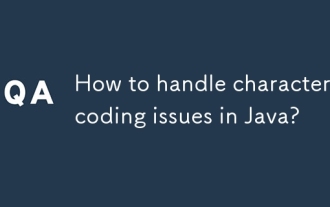
Javaのキャラクターエンコーディングの問題に対処するために、重要なのは、各ステップで使用されるエンコードを明確に指定することです。 1.テキストを読み書きするときは常にエンコードを指定し、inputstreamreaderとoutputStreamWriterを使用し、明示的な文字セットを渡して、システムのデフォルトエンコードに依存しないようにします。 2.ネットワーク境界で文字列を処理するときに両端が一貫していることを確認し、正しいコンテンツタイプのヘッダーを設定し、ライブラリでエンコードを明示的に指定します。 3. string.getBytes()およびNewString(byte [])を注意して使用し、プラットフォームの違いによって引き起こされるデータの破損を避けるために、常に手動でstardantcharsets.utf_8を指定します。要するに、
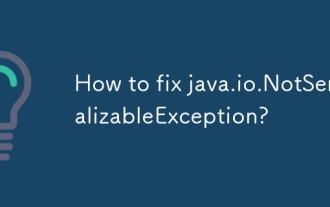
java.io.notserializableExceptionに遭遇するためのコアワークアウンドは、シリアル化する必要があるすべてのクラスがシリアル化可能なインターフェイスを実装し、ネストされたオブジェクトのシリアル化サポートを確認することです。 1.メインクラスに機器を追加する可能性のあるものを追加します。 2.クラス内の対応するカスタムフィールドのクラスも、シリアル化可能なものを実装していることを確認します。 3.一時的に使用して、シリアル化する必要のないフィールドをマークする。 4.コレクションまたはネストされたオブジェクトの非シリアル化されたタイプを確認します。 5.どのクラスがインターフェイスを実装していないかを確認します。 6.キーデータの保存やシリアル化可能な中間構造の使用など、変更できないクラスの交換設計を検討します。 7.変更を検討してください
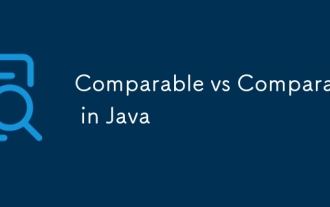
Javaでは、Defaultのデフォルトソートルールを内部的に定義するためにAcparableが使用され、コンパレータを使用して複数のソートロジックを外部から定義します。 1.Comparableは、クラス自体によって実装されるインターフェイスです。比較()メソッドを書き換えることにより、自然な順序を定義します。弦や整数など、固定および最も一般的に使用されるソートメソッドを備えたクラスに適しています。 2。Comparatorは、同じクラスに複数の並べ替え方法が必要な状況に適した、Compare()メソッドを介して実装された外部定義の機能インターフェイスであり、クラスソースコードを変更できない、またはソートロジックが変更されることが多い場合があります。 2つの違いは、比較可能がソートロジックを定義するだけで、クラス自体を変更する必要があることですが、比較して
