インタビュー キット: 配列 - スライディング ウィンドウ。
重要なのはパターンです!
パターンを学ぶと、すべてが少し簡単に感じ始めます。あなたも私と同じなら、おそらく技術系の面接は好きではないでしょう。私はあなたを責めません。面接は厳しいものになる可能性があります。
配列の問題は、面接で遭遇する最も一般的な問題の 1 つです。これらの問題には、多くの場合、自然配列の操作が関係します。
const arr = [1, 2, 3, 4, 5];
そして、本質的に文字の配列である文字列の問題:
"mylongstring".split(""); // ['m', 'y', 'l', 'o','n', 'g', 's','t','r','i', 'n', 'g']
配列の問題を解決するための最も一般的なパターンの 1 つは、スライディング ウィンドウです。
スライディング ウィンドウ パターン
スライディング ウィンドウ パターンには、配列内をスライドするウィンドウのように、同じ方向に移動する 2 つのポインターが含まれます。
いつ使用するか
最小値、最大値、最長値など、特定の条件を満たす サブ配列 または サブ文字列 を検索する必要がある場合は、スライディング ウィンドウ パターンを使用します。最短です。
ルール 1: サブ配列またはサブ文字列を検索する必要があり、データ構造が配列または文字列である場合は、スライディング ウィンドウ パターンの使用を検討してください。
簡単な例
スライディング ウィンドウにポインターの概念を導入する基本的な例を次に示します。
function SlidingWindow(arr) { let l = 0; // Left pointer let r = l + 1; // Right pointer while (r < arr.length) { console.log("left", arr[l]); console.log("right", arr[r]); l++; r++; } } SlidingWindow([1, 2, 3, 4, 5, 6, 7, 8]);
左 (L) と右 (R) ポインターは同時に移動する必要はありませんが、同じ方向に移動する必要があることに注意してください。
右ポインタが左ポインタよりも低くなることはありません。
実際の面接の問題でこの概念を探ってみましょう。
現実の問題: 繰り返し文字を含まない最長の部分文字列
問題: 文字列 s を指定して、文字を繰り返さない最長の部分文字列の長さを見つけます。
キーワード: サブ-文字列、最長 (最大)
function longestSubstr(str) { let longest = 0; let left = 0; let hash = {}; for (let right = 0; right < str.length; right++) { if (hash[str[right]] !== undefined && hash[str[right]] >= left) { left = hash[str[right]] + 1; } hash[str[right]] = right; longest = Math.max(longest, right - left + 1); } return longest; }
これが複雑に見えても心配しないでください。少しずつ説明していきます。
let str = "helloworld"; console.log(longestSubstr(str)); // Output: 5
この問題の核心は、繰り返し文字を含まない最長の部分文字列を見つけることです。
初期ウィンドウ: サイズ 0開始時には、左 (L) と右 (R) のポインターは両方とも同じ場所にあります。
let left = 0; for (let right = 0; right < str.length; right++) { // RIGHT POINTER
h e l l o w o r l d ^ ^ L R
let hash = {};
キーを保存しており、これがまさにこの問題を解決するために必要なものです。ハッシュを使用して、訪問したすべてのキャラクターを追跡し、現在のキャラクターを以前に見たことがあるかどうかを確認します (重複を検出するため)。
文字列を見ると、world が文字を繰り返さない最長の部分文字列であることが視覚的にわかります。
h e l l o w o r l d ^ ^ L R
段階的に見てみましょう:
初期状態
hash = {} h e l l o w o r l d ^ ^ L R
各反復で、R ポインタの下の文字をハッシュ マップに追加し、インクリメントします。
hash[str[right]] = right; longest = Math.max(longest, right - left + 1);
hash = {h: 0} h e l l o w o r l d ^ ^ L R
hash = {h: 0, e: 1} h e l l o w o r l d ^ ^ L R
反復 3:
hash = {h: 0, e: 1, l: 2} h e l l o w o r l d ^ ^ L R
if (hash[str[right]] !== undefined)
それでは、次に何をしましょうか?すでに左側の部分文字列を処理しているため、L ポインタを上に移動してウィンドウを左側から縮小します。しかし、L をどこまで移動すればよいでしょうか?
left = hash[str[right]] + 1;
hash = {h: 0, e: 1, l: 2} h e l l o w o r l d ^ ^ L R
hash[str[right]] = right; longest = Math.max(longest, right - left + 1);
hash = {h: 0, e: 1, l: 3} h e l l o w o r l d ^ ^ L R
hash = {h: 0, e: 1, l: 3, o: 4, w: 5} h e l l o w o r l d ^ ^ L R
hash = {h: 0, e: 1, l: 3, o: 4, w: 5} h e l l o w o r l d ^ ^ L R
が見つかるまで続行します。
hash = {h: 0, e: 1, l: 3, o: 4, w: 5, o: 6, r: 7} h e l l o w o r l d ^ ^ L R
ルール 3: 処理されたサブ x を無視する
現在のウィンドウの外側にあるものはすべて無関係です。すでに処理されています。これを管理するためのキーコードは次のとおりです:
if (hash[str[right]] !== undefined && hash[str[right]] >= left)
hash[str[right]] >= left
最終反復:
hash = {h: 0, e: 1, l: 8, o: 4, w: 5, o: 6, r: 7} h e l l o w o r l d ^ ^ L R
In Summary:
- Rule 1: Keywords in the problem (e.g., maximum, minimum) are clues. This problem is about finding the longest sub-string without repeating characters.
- Rule 2: If you need to find unique or non-repeating elements, think hash maps.
- Rule 3: Focus on the current window—anything outside of it is irrelevant.
Bonus Tips:
- Break down the problem and make it verbose using a small subset.
- When maximizing the current window, think about how to make it as long as possible. Conversely, when minimizing, think about how to make it as small as possible.
To wrap things up, here's a little challenge for you to try out! I’ll post my solution in the comments—it’s a great way to practice.
Problem 2: Sum Greater Than or Equal to Target
Given an array, find the smallest subarray with a sum equal to or greater than the target(my solution will be the first comment).
/** * * @param {Array<number>} arr * @param {number} target * @returns {number} - length of the smallest subarray */ function greaterThanOrEqualSum(arr, target){ let minimum = Infinity; let left = 0; let sum = 0; // Your sliding window logic here! }
Remember, like anything in programming, repetition is key! Sliding window problems pop up all the time, so don’t hesitate to Google more examples and keep practicing.
I’m keeping this one short, but stay tuned—the next article will dive into the two-pointer pattern and recursion (prepping for tree problems). It’s going to be a bit more challenging!
If you want more exclusive content, you can follow me on Twitter or Ko-fi I'll be posting some extra stuff there!
Resources:
Tech interview Handbook
leet code arrays 101
以上がインタビュー キット: 配列 - スライディング ウィンドウ。の詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。

ホットAIツール

Undresser.AI Undress
リアルなヌード写真を作成する AI 搭載アプリ

AI Clothes Remover
写真から衣服を削除するオンライン AI ツール。

Undress AI Tool
脱衣画像を無料で

Clothoff.io
AI衣類リムーバー

AI Hentai Generator
AIヘンタイを無料で生成します。

人気の記事

ホットツール

メモ帳++7.3.1
使いやすく無料のコードエディター

SublimeText3 中国語版
中国語版、とても使いやすい

ゼンドスタジオ 13.0.1
強力な PHP 統合開発環境

ドリームウィーバー CS6
ビジュアル Web 開発ツール

SublimeText3 Mac版
神レベルのコード編集ソフト(SublimeText3)

ホットトピック










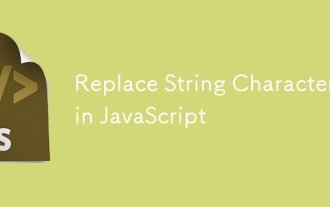
JavaScript文字列置換法とFAQの詳細な説明 この記事では、javaScriptの文字列文字を置き換える2つの方法について説明します:内部JavaScriptコードとWebページの内部HTML。 JavaScriptコード内の文字列を交換します 最も直接的な方法は、置換()メソッドを使用することです。 str = str.replace( "find"、 "置換"); この方法は、最初の一致のみを置き換えます。すべての一致を置き換えるには、正規表現を使用して、グローバルフラグGを追加します。 str = str.replace(/fi
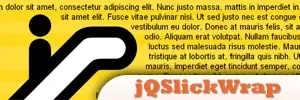
楽なWebページレイアウトのためにjQueryを活用する:8本質的なプラグイン jQueryは、Webページのレイアウトを大幅に簡素化します。 この記事では、プロセスを合理化する8つの強力なjQueryプラグイン、特に手動のウェブサイトの作成に役立ちます
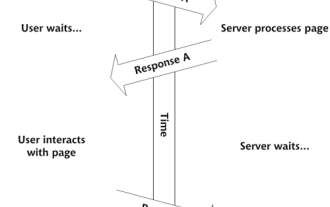
それで、あなたはここで、Ajaxと呼ばれるこのことについてすべてを学ぶ準備ができています。しかし、それは正確には何ですか? Ajaxという用語は、動的でインタラクティブなWebコンテンツを作成するために使用されるテクノロジーのゆるいグループ化を指します。 Ajaxという用語は、もともとJesse Jによって造られました
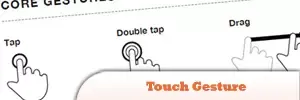
この投稿は、Android、BlackBerry、およびiPhoneアプリ開発用の有用なチートシート、リファレンスガイド、クイックレシピ、コードスニペットをコンパイルします。 開発者がいないべきではありません! タッチジェスチャーリファレンスガイド(PDF) Desigの貴重なリソース
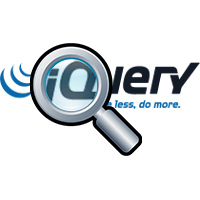
jQueryは素晴らしいJavaScriptフレームワークです。ただし、他のライブラリと同様に、何が起こっているのかを発見するためにフードの下に入る必要がある場合があります。おそらく、バグをトレースしているか、jQueryが特定のUIをどのように達成するかに興味があるからです
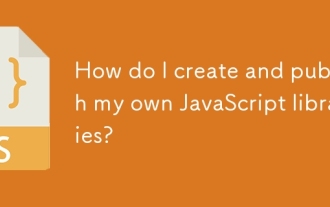
記事では、JavaScriptライブラリの作成、公開、および維持について説明し、計画、開発、テスト、ドキュメント、およびプロモーション戦略に焦点を当てています。
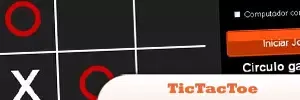
10の楽しいjQueryゲームプラグインして、あなたのウェブサイトをより魅力的にし、ユーザーの粘着性を高めます! Flashは依然としてカジュアルなWebゲームを開発するのに最適なソフトウェアですが、jQueryは驚くべき効果を生み出すこともできます。また、純粋なアクションフラッシュゲームに匹敵するものではありませんが、場合によってはブラウザで予期せぬ楽しみもできます。 jquery tic toeゲーム ゲームプログラミングの「Hello World」には、JQueryバージョンがあります。 ソースコード jQueryクレイジーワードコンポジションゲーム これは空白のゲームであり、単語の文脈を知らないために奇妙な結果を生み出すことができます。 ソースコード jquery鉱山の掃引ゲーム
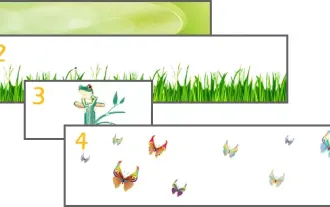
このチュートリアルでは、jQueryを使用して魅惑的な視差の背景効果を作成する方法を示しています。 見事な視覚的な深さを作成するレイヤー画像を備えたヘッダーバナーを構築します。 更新されたプラグインは、jQuery 1.6.4以降で動作します。 ダウンロードしてください
