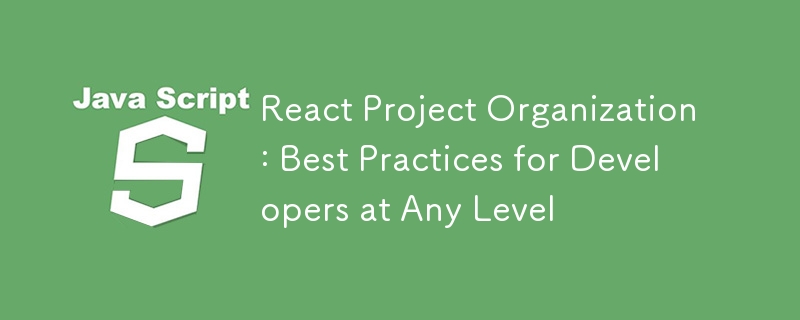
React プロジェクト構造をマスターする: すべてのレベル向けのフレンドリーなガイド
React の世界へようこそ!始めたばかりの人でも、いくつかのプロジェクトを抱えている人でも、ベテランのプロでも、プロジェクトを整理するのは迷路を進むように感じることがあります。でも心配しないでください、私がしっかりカバーします!この投稿では、React プロジェクトをきちんと構造化し、スケーラブルで管理しやすい状態に保つ方法を検討します。飛び込んでみましょう!
初心者向け: シンプルかつ魅力的なものを保つ
React を初めて使用する場合は、ファイルの置き場所についてあまり心配せずに、単に作業を進めたいだけかもしれません。全然大丈夫ですよ!この段階では、基本を学び、フレームワークに慣れることが重要です。
基本的なフォルダー構造:
-
コンポーネント: このフォルダーには、基本的なボタンからより複雑なフォームに至るまで、すべての React コンポーネントが含まれています。コンポーネントを 1 か所に整理すると、初心者でもコンポーネントを簡単に見つけて操作できるようになります。
-
フック: カスタム フックは別のフォルダーに保存されます。フックは、機能コンポーネントの状態と副作用を管理するために不可欠です。
-
テスト: すべてのテスト ファイルは、テスト対象のコードとは別に、単一のフォルダーに配置されます。この分離は、プロジェクトの明確さと組織性を維持するのに役立ちます。
例:
/src
/components
/hooks
/tests
index.js
App.js
ログイン後にコピー
このセットアップは簡単で、圧倒されることなくコンポーネントとフックを追跡するのに役立ちます。
中級開発者向け: 組織化とスケールアップ
React に慣れてきて、より複雑なアプリケーションの構築を開始したら、プロジェクトに構造を追加します。ここで物事がもう少し整理され、スケーラビリティについて考え始めます。
中間フォルダー構造:
-
アセット: ここに、すべての画像、グローバル CSS ファイル、およびその他の非 JavaScript アセットを保管します。これはアプリのツールボックスのようなものです。
-
Context: 状態管理に React の Context API を使用している場合、ここにコンテキスト関連のファイルがすべて保存されます。これは、アプリのさまざまな部分の状態を管理するのに役立ちます。
-
データ: JSON ファイルまたはその他のデータ定数をここに保存します。データをロジックや UI から分離すると、管理と更新が容易になります。
-
ページ: 複数のページがあるアプリの場合、ページごとに個別のフォルダーを作成することをお勧めします。このようにして、各ページに独自のコンポーネントとスタイルのセットを含めることができます。
-
Utils: ユーティリティ関数とヘルパーがここにあります。これらは、一般的なタスクを処理することで作業を容易にするコードの小さなスニペットです。
例:
/src
/assets
/components
/context
/data
/pages
/utils
index.js
App.js
ログイン後にコピー
この構造は、整理された状態を維持するのに役立つだけでなく、コードベースの移動と保守も容易になります。それは、単純なツールボックスから、よく組織されたワークショップにアップグレードするようなものです。
上級開発者向け: 堅牢でスケーラブルなアーキテクチャの作成
上級レベルでは、堅牢なアーキテクチャを必要とする大規模なアプリケーションやプロジェクトに取り組んでいることになるでしょう。ここでの目標は、高度にモジュール化され、保守しやすいコードベースを作成することです。この構造は、複雑さを適切に処理できるように設計されています。
Advanced Folder Structure:
-
Public: Contains static files like your index.html, which is the entry point of your app. It’s like the welcome mat of your project.
-
Src: The main hub of your application, neatly divided into several subdirectories.
-
Assets: Further divided into categories like audios, icons, images, and videos. It’s your multimedia corner.
-
Components: Organized by functionality or feature, with each component having its own folder. This includes the component file, its styles, and any related assets.
-
Contexts: For managing state and contexts across your app. It’s like the control room for your app’s state.
-
Services: If your app talks to APIs or performs other backend tasks, those logic pieces go here.
-
Store: For global state management using libraries like Redux or MobX. It’s your app’s memory bank.
-
Hooks: Custom hooks, organized by their purpose. Think of these as your app’s special abilities.
-
Pages: Each major page or view of your app gets its own folder. This keeps your app’s structure tidy and easy to follow.
-
Utils: Advanced utility functions and higher-order components (HOCs) live here. These are the tools that make your app smarter and more efficient.
Example:
/public
index.html
/src
/assets
/audios
/icons
/images
/videos
/components
/Button
index.jsx
button.module.css
/Modal
index.jsx
modal.module.css
/contexts
/services
/store
/hooks
/pages
/utils
index.js
App.js
ログイン後にコピー
This structure is like a well-oiled machine, designed to handle the complexity of large-scale apps with ease. It ensures that everything has its place, making the codebase easy to navigate and maintain.
Wrapping It All Up: Finding Your Perfect Fit
Choosing the right folder structure for your React project depends on your project's needs and your team's workflow. Start simple if you're a beginner, and gradually adopt more sophisticated structures as your project grows and your skills improve. Remember, there's no one-size-fits-all solution—adapt these structures to what works best for you and your team.
A well-organized project not only makes development smoother but also helps when collaborating with others or bringing new developers on board. As you continue to work with React, don't be afraid to refine and tweak your structure. The goal is always to make your codebase as clean, efficient, and maintainable as possible.
Happy coding!
以上がReact プロジェクトの組織化: あらゆるレベルの開発者のためのベスト プラクティスの詳細内容です。詳細については、PHP 中国語 Web サイトの他の関連記事を参照してください。