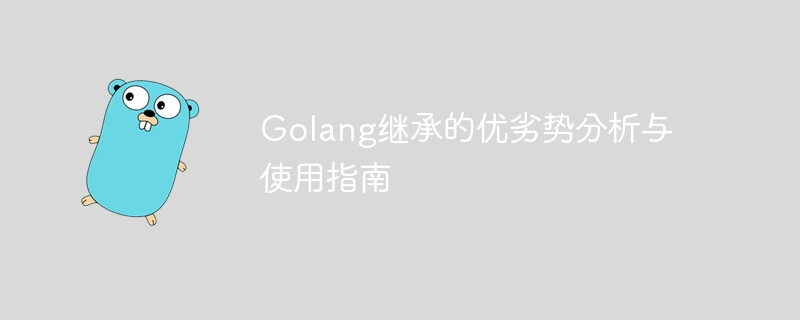
Golang inheritance advantages and disadvantages analysis and usage guide
Introduction:
Golang is an open source programming language with the characteristics of simplicity, efficiency and concurrency. As an object-oriented programming language, Golang provides code reuse through composition rather than inheritance.
Inheritance is a commonly used concept in object-oriented programming, which allows one class to inherit the properties and methods of another class. However, in Golang, inheritance is not a preferred programming method, but code reuse is achieved through the combination of interfaces. In this article, we will discuss the pros and cons of inheritance in Golang and provide some suggestions and usage guidelines.
Advantages:
- Simplify and reduce code complexity: Through inheritance, subclasses can inherit properties and methods from parent classes, thus avoiding writing the same code repeatedly. This greatly simplifies and reduces the complexity of your code.
- Improve the maintainability and scalability of code: Through inheritance, related properties and methods can be organized together, making the code clearer and easier to understand. In addition, if you need to modify a method of the parent class or add a new method, you only need to perform the corresponding operation in the subclass without affecting other classes.
- Code reusability: Through inheritance, code reuse can be achieved and repetitive work can be reduced. If multiple classes have the same properties and methods, they can be defined in a parent class and share code through inheritance.
Disadvantages:
- Increase the coupling of the code: inheritance will tightly couple the parent class and the subclass together. If the implementation of the parent class changes, it will affect all subclasses that inherit from that class. This increases the fragility of the code, making it difficult to maintain and extend.
- Complexity of inheritance hierarchy: When the inheritance hierarchy is too complex, it becomes difficult to understand and maintain the code. As the level of inheritance goes deeper, the complexity of the code increases accordingly.
- Destruction of the open-close principle: Inheritance will destroy the open-close principle, that is, it is open to expansion and closed to modification. If you need to modify the implementation of the parent class, it will affect all subclasses that inherit from that class, which violates this principle.
Usage Guide:
- Prioritize the use of interfaces: In Golang, it is recommended to use a combination of interfaces to achieve code reuse, rather than through inheritance. The combination of interfaces can organize code more flexibly, reduce code coupling, and follow the principles of interface-oriented programming.
- Use nested structures: In Golang, you can use nested structures to simulate the inheritance relationship of classes. Through nested structures, different properties and methods can be organized together to simplify the structure of the code.
- Pay attention to the rationality of the inheritance relationship: When designing the inheritance relationship, you need to pay attention to the rationality and applicability. You should not abuse inheritance for the sake of simplicity, but consider whether the inheritance relationship is actually needed to solve the problem.
Sample code:
// 定义一个人的结构体
type Person struct {
Name string
Age int
}
// 定义一个学生的结构体,继承自Person
type Student struct {
Person
Grade int
}
// 定义Person的方法
func (p *Person) SayHello() {
fmt.Println("Hello, my name is", p.Name)
}
// 定义Student的方法
func (s *Student) Study() {
fmt.Println("I am studying in grade", s.Grade)
}
func main() {
// 创建一个学生对象
student := Student{
Person: Person{
Name: "Alice",
Age: 18,
},
Grade: 12,
}
// 调用继承自Person的方法
student.SayHello() // 输出:Hello, my name is Alice
// 调用Student自己的方法
student.Study() // 输出:I am studying in grade 12
}
Copy after login
Conclusion:
Although inheritance still has its advantages in some scenarios, in Golang, it is recommended to use a combination of interfaces to implement the code Reuse. Through the combination of interfaces, the coupling of the code can be reduced and the maintainability and scalability of the code can be improved.
The above is the detailed content of Analysis of advantages and disadvantages of Golang inheritance and usage suggestions. For more information, please follow other related articles on the PHP Chinese website!