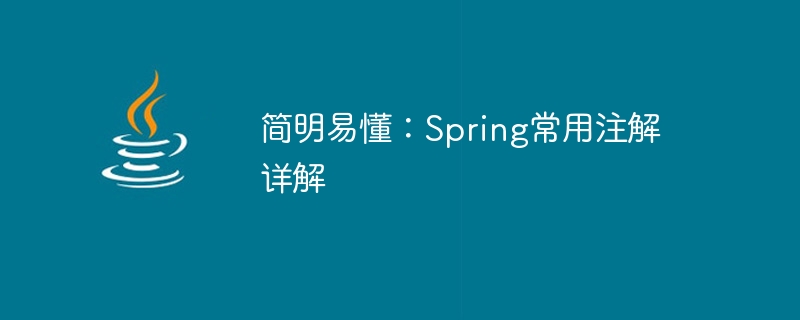
Spring is an open source framework that provides many annotations to simplify and enhance Java development. This article will explain commonly used Spring annotations in detail and provide specific code examples.
- @Autowired: Autowiring
@Autowired annotations can be used to automatically wire beans in the Spring container. When we use the @Autowired annotation where dependencies are required, Spring will find matching beans in the container and automatically inject them. The sample code is as follows:
@Autowired private UserService userService;
Copy after login
- @Component: Component
@Component annotation is used to identify a class as a Spring component, allowing Spring to automatically instantiate it as a Bean during scanning. . The sample code is as follows:
@Component public class UserService { // 业务逻辑代码 }
Copy after login
- @Controller: Controller
@Controller annotation is used to identify a class as a controller of the Spring MVC framework, handle requests and return responses. The sample code is as follows:
@Controller public class UserController { // 处理请求的代码 }
Copy after login
- @Service: The service
@Service annotation is used to identify a class as a Spring service layer component and is used to encapsulate business logic. The sample code is as follows:
@Service public class UserService { // 业务逻辑代码 }
Copy after login
- @Repository: Warehouse
@Repository annotation is used to identify components of the data access layer, usually used to interact with the database. The sample code is as follows:
@Repository public class UserRepository { // 数据访问方法 }
Copy after login
- @RequestMapping: Request mapping
@RequestMapping annotation is used to map the request URL to a processing method. The sample code is as follows:
@Controller @RequestMapping("/user") public class UserController { @RequestMapping("/login") public String login() { // 处理登录请求 } }
Copy after login
- @PathVariable: Request path parameter
@PathVariable annotation is used to bind the path parameters in the URL to the parameters of the method. The sample code is as follows:
@Controller @RequestMapping("/user") public class UserController { @RequestMapping("/profile/{id}") public String viewProfile(@PathVariable("id") int id) { // 根据id查询用户信息 } }
Copy after login
- @RequestParam: Request parameter
@RequestParam annotation is used to bind the request parameters to the parameters of the method. The sample code is as follows:
@Controller @RequestMapping("/user") public class UserController { @RequestMapping("/profile") public String viewProfile(@RequestParam("id") int id) { // 根据id查询用户信息 } }
Copy after login
- @ResponseBody: Return JSON data
@ResponseBody annotation is used to return the return value of the method directly as the data of the response body. The sample code is as follows:
@Controller @RequestMapping("/user") public class UserController { @RequestMapping("/profile/{id}") @ResponseBody public User viewProfile(@PathVariable("id") int id) { // 根据id查询用户信息 return userService.getUserById(id); } }
Copy after login
- @Transactional: Transaction Management
@Transactional annotation is used to mark the method as a transaction processing method. The sample code is as follows:
@Service public class UserService { @Transactional public void updateUser(User user) { // 更新用户信息的操作 } }
Copy after login
The above are some commonly used Spring annotations, which can greatly simplify and enhance Java development. By using these annotations, we can manage and organize our code more conveniently and improve development efficiency.
The above is the detailed content of Spring Annotation Revealed: Analysis of Common Annotations. For more information, please follow other related articles on the PHP Chinese website!