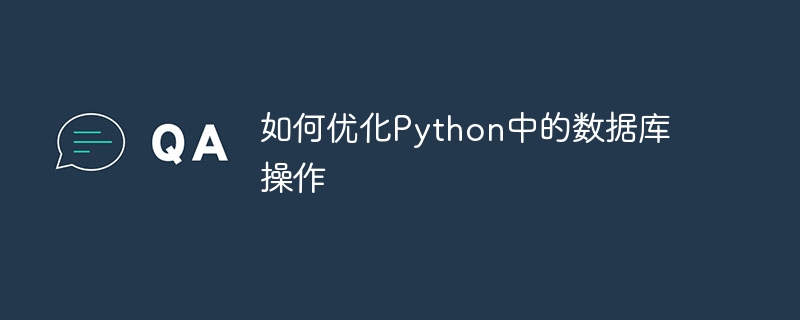
How to optimize database operations in Python
Overview:
As the amount of data continues to increase, database operations become more and more difficult in many projects The essential. This article will take you through how to optimize database operations in Python and improve the performance and efficiency of your code. We will focus on the following aspects: choosing an appropriate database system, optimizing query statements, using batch operations, caching query results, and properly handling database connections.
- Choose a suitable database system:
Before starting optimization, you must first choose a database system that suits the project needs. Different database systems have different performance characteristics and limitations. Commonly used database systems include MySQL, PostgreSQL, SQLite, etc. For large-scale data processing, MySQL and PostgreSQL are common choices, while for small-scale data operations, SQLite may be more suitable. Reasonable selection of database systems can help improve overall performance. - Optimize query statements:
Reasonable optimization of query statements can significantly increase the speed of database queries.
- Use index:
Database index is an important means to optimize query statements. By creating indexes on important fields, you can speed up queries. For example, using MySQL's CREATE INDEX
statement to create an index can greatly improve query efficiency. - Avoid using
SELECT *
:
Querying only the required fields can reduce unnecessary data transmission and processing. When a database table contains a large number of fields, using the SELECT *
statement may cause performance degradation. - Use JOIN statement:
When you need to query multiple tables, you can use the JOIN statement to merge multiple queries into one, reducing the load on the database. At the same time, reasonable selection of JOIN types (such as INNER JOIN, LEFT JOIN) will also help optimize query performance.
- Use batch operations:
Batch operations can reduce the cost of database connections and improve performance.
- Use
executemany
instead of execute
:
When you need to perform the same insert operation repeatedly, you can use executemany
The method inserts multiple records at one time instead of executing the execute
method multiple times. - Use
LOAD DATA
:
For batch insertion of large amounts of data, you can use the database's fast import function, such as MySQL's LOAD DATA
statement. This method is faster than inserting items one by one and can greatly improve insertion performance.
- Caching query results:
For situations where query results rarely change, you can consider caching the results to avoid frequent database queries.
- Use caching libraries:
There are many excellent caching libraries in Python, such as Redis, Memcached, etc. You can use these libraries to cache the query results, and get them directly from the cache the next time you need to query, to avoid requesting the database again. - Set an appropriate expiration time:
For cached data, you need to set a reasonable expiration time. If the data is updated, you can manually update the cache, or wait for the cache to expire before querying the database again.
- Properly handle database connections:
The establishment and disconnection of database connections require overhead, so the life cycle of the connection needs to be properly handled.
- Use connection pool:
Using connection pool can avoid frequent creation and destruction of connections and reduce connection overhead. Common connection pools include DBUtils
and SQLAlchemy
, etc. - Batch processing connection:
When multiple database operations need to be performed, use the same connection as much as possible. This can reduce the overhead of creating a new connection for each operation.
Sample code:
The following is a sample code that shows how to optimize query statements using a MySQL database:
import mysql.connector
# 连接数据库
conn = mysql.connector.connect(user='username', password='password', host='127.0.0.1', database='mydatabase')
# 创建游标对象
cursor = conn.cursor()
# 创建索引
cursor.execute("CREATE INDEX idx_name ON mytable (name)")
# 查询数据
cursor.execute("SELECT id, name FROM mytable WHERE age > 18")
# 获取结果
result = cursor.fetchall()
# 输出结果
for row in result:
print(f"ID: {row[0]}, Name: {row[1]}")
# 关闭游标和连接
cursor.close()
conn.close()
Copy after login
Summary:
By choosing an appropriate database system , optimizing query statements, using batch operations, caching query results, and properly handling database connections can significantly improve the efficiency of database operations in Python. According to the project needs and actual situation, the reasonable use of these optimization techniques can greatly improve the performance and efficiency of the code.
The above is the detailed content of How to optimize database operations in Python. For more information, please follow other related articles on the PHP Chinese website!