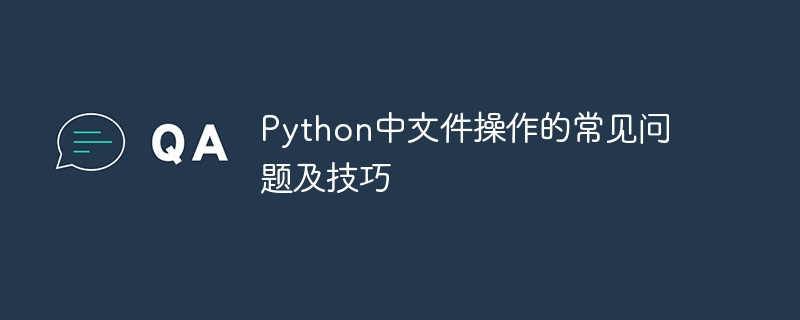
Common problems and techniques for file operations in Python
1. Common problems with file operations
- File path problems:
When we need to operate a file, we first need to make sure that our path to the file is correct. Common problems include:
- File path does not exist: When the file path we specify does not exist, Python will throw a FileNotFoundError exception. In order to avoid this problem, we can use the
os.path.exists()
function to check whether the file path exists.
- Relative path and absolute path: Relative path is relative to the current working directory, while absolute path is the path starting from the root directory. When writing code, try to use absolute paths to avoid unnecessary problems.
- Problems with opening and closing files:
When operating a file, we need to use the open()
function to open the file, and use # after the operation is completed. ##close()Function to close the file. However, sometimes we forget to close files, resulting in wasted resources or files that cannot be deleted immediately. To avoid this problem, we can use the
with statement to automatically close the file.
with open('file.txt', 'r') as f:
# 文件操作代码
Copy after login
Encoding issues: - When reading and writing files, encoding issues may cause garbled characters or failure to parse text content properly. To avoid this problem, we can specify the character encoding of the file. Common character encodings include UTF-8 and GBK.
with open('file.txt', 'r', encoding='utf-8') as f:
# 读取文件内容
with open('file.txt', 'w', encoding='utf-8') as f:
# 写入文件内容
Copy after login
2. Common skills of file operations
Reading and writing files: - We can use the
read() function To read the contents of the file, use the
write() function to write the contents of the file. At the same time, you can also use the
readlines() function to read the file content line by line.
# 读取文件内容
with open('file.txt', 'r') as f:
content = f.read()
# 写入文件内容
with open('file.txt', 'w') as f:
f.write('Hello, World!')
# 按行读取文件内容
with open('file.txt', 'r') as f:
lines = f.readlines()
Copy after login
Copying and moving files: - If we need to copy a file to another location, we can use the
copy( of the shutil
module )function. If we need to move a file to another location, we can use the
move() function of the
shutil module.
import shutil
# 复制文件
shutil.copy('file.txt', 'new_file.txt')
# 移动文件
shutil.move('file.txt', 'new_file.txt')
Copy after login
Deletion of files: - If we need to delete a file, we can use the
remove() function of the
os module.
import os
# 删除文件
os.remove('file.txt')
Copy after login
Renaming of files: - If we need to rename a file, we can use the
rename() of the
os module function.
import os
# 重命名文件
os.rename('file.txt', 'new_file.txt')
Copy after login
File attributes and information: - If we need to obtain the file size, creation time and other attributes, we can use the functions of the
os.path module.
import os.path
# 获取文件大小
size = os.path.getsize('file.txt')
# 获取文件创建时间
ctime = os.path.getctime('file.txt')
Copy after login
To sum up, when performing file operations in Python, we need to pay attention to common problems such as file path issues, closing files in a timely manner, and handling encoding issues. At the same time, mastering common skills such as reading and writing, copying and moving, deleting and renaming files can help us better operate files. In actual development, if you encounter other file operation problems, you can solve them by consulting official documents and learning related libraries.
The above is the detailed content of Frequently Asked Questions and Tips on File Operations in Python. For more information, please follow other related articles on the PHP Chinese website!