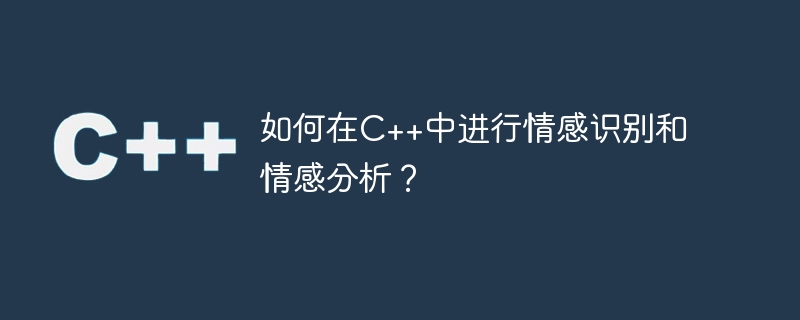
How to perform emotion recognition and sentiment analysis in C?
Overview:
Emotion recognition and sentiment analysis are one of the important applications in the field of natural language processing. It can help us understand the emotional color in text, and plays an important role in public opinion monitoring, sentiment analysis and other scenarios. This article will introduce how to implement the basic methods of emotion recognition and emotion analysis in C, and provide corresponding code examples.
- Data preparation
To perform emotion recognition and sentiment analysis, you first need to prepare a data set suitable for the task. Datasets typically contain a large number of annotated text samples, each with an emotional category label (such as positive, negative, or neutral). Public data sets can be used, such as IMDb movie evaluation data, Twitter sentiment analysis data, etc. You can also collect data yourself and label it manually.
- Text preprocessing
Before performing sentiment analysis, the original text needs to be preprocessed. The main goal of preprocessing is to remove noise and irrelevant information, making the text more suitable for subsequent feature extraction and classification. Common preprocessing steps include: punctuation removal, stop word filtering, word stemming, etc. In C, you can use existing text processing libraries, such as Boost library and NLTK library, to complete these tasks.
- Feature extraction
Feature extraction is the core step of emotion recognition and emotion analysis. By converting text into feature vectors, machine learning algorithms can be helped to better understand and classify the sentiment of text. Common feature extraction methods include: bag-of-words model, TF-IDF, word vector, etc. In C, third-party libraries, such as LIBSVM library and GloVe library, can be used to implement feature extraction.
The following is a simple sample code that demonstrates how to use the bag-of-words model for feature extraction:
#include #include #include
Copy after login
- Model training and classification
After completing feature extraction , the model can be trained using machine learning algorithms and used to classify new texts emotionally. Commonly used machine learning algorithms include naive Bayes, support vector machines, deep learning, etc. Existing machine learning libraries, such as MLlib library and TensorFlow library, can be used in C to complete model training and classification.
The following is a simple sample code that demonstrates how to use the Naive Bayes algorithm for sentiment classification:
#include #include
Copy after login
Summary:
This article describes how to implement it in C Basic methods of emotion recognition and sentiment analysis. Through steps such as preprocessing, feature extraction, model training, and classification, we can accurately judge and classify the sentiment of text. At the same time, we also provide corresponding code examples to help readers better understand and practice emotion recognition and emotion analysis technology. Hope this article is helpful to everyone.
The above is the detailed content of How to perform emotion recognition and sentiment analysis in C++?. For more information, please follow other related articles on the PHP Chinese website!