


How do you use the FILTER_VALIDATE_* and FILTER_SANITIZE_* filters in PHP?
Mar 26, 2025 pm 12:24 PMHow do you use the FILTER_VALIDATE_ and FILTER_SANITIZE_ filters in PHP?
In PHP, the filter_var()
function is used to apply filters to variables, and it supports various filters categorized into two main groups: FILTER_VALIDATE_*
and FILTER_SANITIZE_*
. These filters help in ensuring data integrity and security.
Using FILTER_VALIDATE_* Filters:
-
Purpose: These filters are used to validate data. They check if the input matches certain criteria and return
true
if it does,false
otherwise. -
Usage: To validate an email address, for instance, you can use the
FILTER_VALIDATE_EMAIL
filter:$email = "example@example.com"; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { echo "Valid email address."; } else { echo "Invalid email address."; }
Copy after login
Using FILTER_SANITIZE_* Filters:
- Purpose: These filters are used to sanitize or clean up input data, often to prevent malicious code from being injected.
Usage: To sanitize a string to remove all tags, you could use the
FILTER_SANITIZE_STRING
filter:$input = "<p>Hello, World!</p>"; $sanitized = filter_var($input, FILTER_SANITIZE_STRING); echo $sanitized; // Outputs: "Hello, World!"
Copy after login
What are the specific differences between FILTER_VALIDATE_ and FILTER_SANITIZE_ filters in PHP?
The main differences between FILTER_VALIDATE_*
and FILTER_SANITIZE_*
filters in PHP are their purposes and the way they handle data:
Purpose:
FILTER_VALIDATE_*
filters are designed to validate data against specific criteria. They return a boolean value indicating whether the data is valid or not.FILTER_SANITIZE_*
filters are used to clean up data, removing unwanted characters or formatting the data to prevent security vulnerabilities.
Output:
FILTER_VALIDATE_*
filters typically returntrue
orfalse
, or the original value if it's valid (depending on the filter).FILTER_SANITIZE_*
filters return the sanitized version of the input data.
Usage Context:
FILTER_VALIDATE_*
is used when you need to check if the data meets certain standards before processing it further.FILTER_SANITIZE_*
is used to prepare data for safe use, such as storing in a database or displaying on a webpage.
How can you effectively implement FILTER_SANITIZE_* filters to enhance security in PHP applications?
Implementing FILTER_SANITIZE_*
filters effectively can significantly enhance the security of PHP applications. Here are some strategies:
Input Sanitization:
Always sanitize user input before processing or storing it. For example, useFILTER_SANITIZE_STRING
to remove HTML tags from user input:$userInput = $_POST['user_input']; $sanitizedInput = filter_var($userInput, FILTER_SANITIZE_STRING);
Copy after loginPreventing SQL Injection:
UseFILTER_SANITIZE_SPECIAL_CHARS
to escape special characters that could be used in SQL injection attacks:$username = $_POST['username']; $sanitizedUsername = filter_var($username, FILTER_SANITIZE_SPECIAL_CHARS);
Copy after loginPreventing XSS Attacks:
Sanitize data that will be displayed in HTML to prevent cross-site scripting (XSS) attacks. UseFILTER_SANITIZE_FULL_SPECIAL_CHARS
to convert special characters to their HTML entities:$comment = $_POST['comment']; $sanitizedComment = filter_var($comment, FILTER_SANITIZE_FULL_SPECIAL_CHARS); echo $sanitizedComment;
Copy after login- Consistent Application:
Apply sanitization consistently across your application, especially for data that comes from external sources.
Which FILTER_VALIDATE_* options are most commonly used for data validation in PHP?
Some of the most commonly used FILTER_VALIDATE_*
options in PHP for data validation include:
FILTER_VALIDATE_EMAIL:
Used to validate email addresses. It checks if the input string is a valid email format.$email = "example@example.com"; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { echo "Valid email address."; }
Copy after loginFILTER_VALIDATE_URL:
Used to validate URLs. It checks if the input string is a valid URL format.$url = "https://example.com"; if (filter_var($url, FILTER_VALIDATE_URL)) { echo "Valid URL."; }
Copy after loginFILTER_VALIDATE_IP:
Used to validate IP addresses. It checks if the input string is a valid IP address.$ip = "192.168.0.1"; if (filter_var($ip, FILTER_VALIDATE_IP)) { echo "Valid IP address."; }
Copy after loginFILTER_VALIDATE_INT:
Used to validate integers. It checks if the input string is a valid integer.$number = "42"; if (filter_var($number, FILTER_VALIDATE_INT)) { echo "Valid integer."; }
Copy after login
These filters are essential for ensuring that the data your application processes meets the expected format, thereby enhancing the reliability and security of your application.
The above is the detailed content of How do you use the FILTER_VALIDATE_* and FILTER_SANITIZE_* filters in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
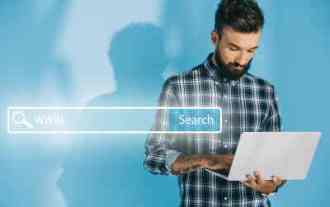
11 Best PHP URL Shortener Scripts (Free and Premium)
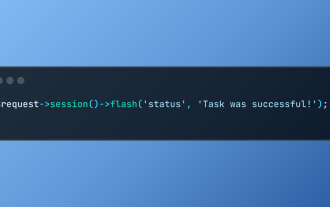
Working with Flash Session Data in Laravel
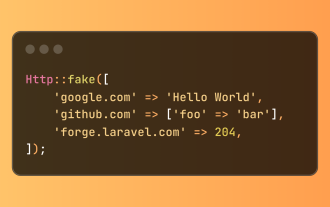
Simplified HTTP Response Mocking in Laravel Tests
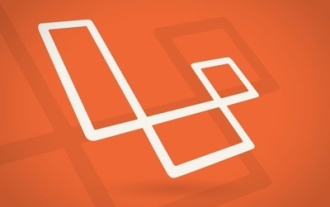
Build a React App With a Laravel Back End: Part 2, React
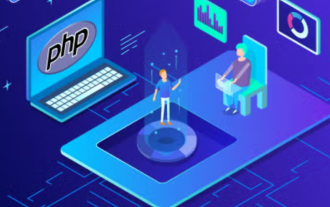
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
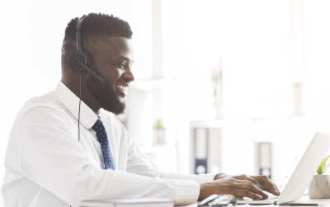
12 Best PHP Chat Scripts on CodeCanyon
