


Describe how Python's exception handling works. How can you create custom exceptions?
Mar 25, 2025 am 11:05 AMDescribe how Python's exception handling works. How can you create custom exceptions?
Python's exception handling mechanism is designed to handle errors or unexpected events gracefully during program execution. The primary construct for exception handling in Python is the try-except
block. Here’s how it works:
-
Try Block: The code that might raise an exception is enclosed in a
try
block. -
Except Block: If an exception is raised within the
try
block, the control is immediately transferred to anexcept
block that handles the exception. Python allows for multipleexcept
blocks to handle different types of exceptions. -
Else Block (optional): An
else
block can be used to execute code when no exceptions are raised in thetry
block. -
Finally Block (optional): A
finally
block is executed regardless of whether an exception occurred or not, often used for cleanup actions.
Example:
try: result = 10 / 0 except ZeroDivisionError: print("Cannot divide by zero!") else: print("Division successful") finally: print("Execution completed")
To create custom exceptions in Python, you can define a new class that inherits from the built-in Exception
class or any of its subclasses. Here's how to do it:
- Define the Custom Exception Class: Create a new class that inherits from
Exception
or a more specific exception class. - Implement Additional Functionality (optional): You can add attributes or methods to your custom exception to provide more detailed information about the error.
Example of creating a custom exception:
class CustomError(Exception): """A custom exception class""" def __init__(self, message, error_code): self.message = message self.error_code = error_code super().__init__(f"{self.message} (Error Code: {self.error_code})") try: raise CustomError("Something went wrong", 500) except CustomError as e: print(e)
What are the benefits of using custom exceptions in Python?
Using custom exceptions in Python offers several benefits:
- Improved Code Readability: Custom exceptions make it clear what type of error has occurred, making the code more readable and self-explanatory.
- Better Error Handling: They allow for more specific and targeted error handling, enabling you to catch and handle different types of errors differently.
- Enhanced Debugging: Custom exceptions can include additional information about the error (like error codes or messages), which can significantly aid in debugging and troubleshooting.
- Maintainability: By using custom exceptions, you can encapsulate error handling logic within the exception class itself, which can make your code easier to maintain and modify.
- Semantic Clarity: Custom exceptions can convey the specific context of an error, making it easier for developers to understand and fix issues.
How can exception handling improve the robustness of Python programs?
Exception handling can significantly improve the robustness of Python programs in several ways:
- Error Management: It allows the program to gracefully handle errors and unexpected conditions without crashing. This means the program can continue running or fail gracefully, informing the user of what went wrong.
- Resource Management: Using
finally
blocks ensures that resources like file handles or network connections are properly closed, even if an error occurs, which prevents resource leaks. - User Experience: Exception handling can improve user experience by providing clear and meaningful error messages, helping users understand what went wrong and what they can do about it.
- Code Modularity: By centralizing error handling logic, you can make your code more modular and easier to maintain. This is especially useful in large projects where errors need to be handled consistently across the application.
- Stability: Exception handling can help isolate errors to specific parts of the code, preventing a single error from bringing down the entire application.
What are some common built-in exceptions in Python and their uses?
Python includes a variety of built-in exceptions that are used to handle common error scenarios. Here are some of the most common ones:
SyntaxError: Raised when the parser encounters a syntax error. This exception helps in identifying syntax issues in the code.
try: eval("print 'Hello, World!'") # Syntax error in Python 3 except SyntaxError as e: print(e)
Copy after loginTypeError: Raised when an operation or function is applied to an object of an inappropriate type.
try: result = "a string" 123 except TypeError as e: print(e)
Copy after loginValueError: Raised when a function receives an argument of the correct type but an inappropriate value.
try: int("not a number") except ValueError as e: print(e)
Copy after loginIndexError: Raised when a sequence subscript is out of range.
try: my_list = [1, 2, 3] print(my_list[10]) except IndexError as e: print(e)
Copy after loginKeyError: Raised when a dictionary key is not found.
try: my_dict = {"a": 1, "b": 2} print(my_dict["c"]) except KeyError as e: print(e)
Copy after loginZeroDivisionError: Raised when the second argument of a division or modulo operation is zero.
try: result = 10 / 0 except ZeroDivisionError as e: print(e)
Copy after loginThese built-in exceptions help developers handle common errors efficiently and make their code more robust and error-resistant.
The above is the detailed content of Describe how Python's exception handling works. How can you create custom exceptions?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
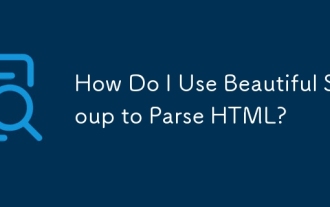
How Do I Use Beautiful Soup to Parse HTML?
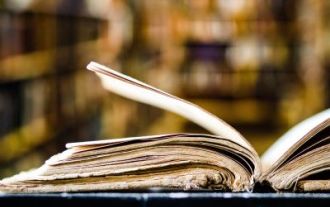
How to Use Python to Find the Zipf Distribution of a Text File
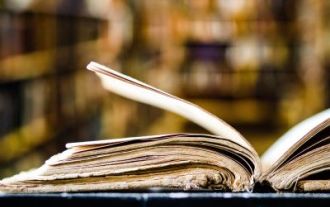
How to Work With PDF Documents Using Python
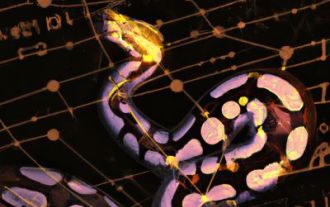
How to Cache Using Redis in Django Applications
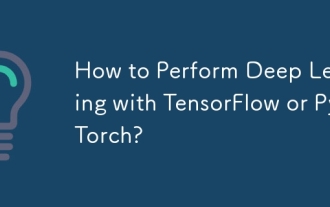
How to Perform Deep Learning with TensorFlow or PyTorch?
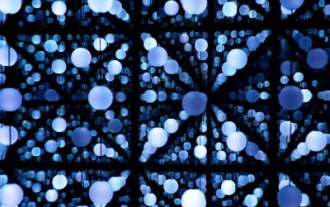
How to Implement Your Own Data Structure in Python
