Using Inline Partials and Decorators with Handlebars 4.0
Handlebars, a popular JavaScript templating library for client-side and server-side rendering, extends the mustache specification for improved template management. For newcomers, exploring JavaScript templating with Handlebars via a Pluralsight course is recommended. Handlebars 4.0 (September 2015) introduced significant enhancements: Inline Partials and Decorators. This article explores both, detailing their syntax and optimal usage.
Key Concepts:
- Handlebars 4.0's key features: Inline Partials (reusable templates) and Decorators (modify rendering states).
- Inline Partials: Defined within templates, eliminating the need for JavaScript registration. They're block-scoped, limiting usage to the current and nested scopes. Ideal for small, reusable HTML snippets.
- Decorators: Modify the Handlebars program function, influencing the runtime before execution. Useful for altering context data or helpers.
- Decorators improve code modularity, extensibility, and testability, particularly beneficial for tasks like currency formatting.
- Both features streamline front-end development, resulting in cleaner, more organized, and potentially more efficient code.
Inline Partials
Partials, a common templating concept, promote code reuse by separating reusable templates into individual files. However, Handlebars' traditional partials have limitations: global scope, requiring JavaScript registration (though often handled by pre-compilers), and separation from their usage context. This often restricts their use to only the largest reusable code blocks.
Inline Partials address these issues. Defined within templates using Handlebars syntax, they require no JavaScript registration. Their block-scoped nature confines their use to the current and child scopes. Choose Inline Partials for small, reusable HTML segments that are either too small for separate files or used exclusively within a single template.
Using Inline Partials
Declare an Inline Partial by wrapping the reusable code with {{#* inline "partialName"}} ... {{/inline}}
. Then, use it within the template via {{> partialName}}
.
Example:
Instead of repeating <code><li>{{firstName}} {{lastName}}</li>
{{firstName}} {{lastName}}
{{#* inline "fullName"}} {{firstName}} {{lastName}} {{/inline}} {{#each clients}} <li>{{> fullName}}</li> {{/each}}
Comparing Partials and Inline Partials
{{firstName}} {{lastName}}
Consider a template with repeated
-
Traditional Partial: Requires a separate JavaScript file to register
Handlebars.registerPartial('fullName', '{{firstName}} {{lastName}}');
and then usage in the template as{{> fullName}}
. This separates the partial's definition, making understanding the full template more challenging. -
Inline Partial: A cleaner, self-contained approach:
{{#* inline "fullName"}} {{firstName}} {{lastName}} {{/inline}} {{#each clients}} <li>{{> fullName}}</li> {{/each}}
Decorators
Decorators modify the Handlebars program function, influencing the rendering process. They provide metadata to enhance template functionality, acting as a more fundamental companion to helper functions.
Handlebars compilation involves: 1. Getting the template; 2. Compiling it; 3. Rendering the output. Decorators intervene in the compilation step (Handlebars.compile
), affecting the block-scoped compiled functions. They control execution before rendering, allowing modifications to context data or helpers.
Using Decorators
Decorators are registered using Handlebars.registerDecorator()
. The function receives (program, props, container, context)
.
program
: The compiled Handlebars function. Modify arguments, return values, or context.props
: Properties set here affect the program function, even if replaced. Useful for metadata.container
: The Handlebars runtime container (partials, helpers, context). Modifiable.context
: The parent context, including Decorator arguments and data.
The Decorator must return a function (or falsy value) to render the modified template.
Example: Currency Formatting
Before Handlebars 4.0, currency formatting often relied on helpers. Decorators offer a more elegant solution.
A Decorator can dynamically register a currency formatting helper based on context:
{{#* inline "fullName"}}{{firstName}} {{lastName}}{{/inline}} <h1 id="Hello-gt-fullName">Hello {{> fullName}}</h1>
Then, in the template:
Handlebars.registerDecorator('activateFormatter', function(program, props, container, context) { // ... logic to select currency formatter based on context ... container.helpers = { formatMoneyHelper: selectedFormatter }; });
This approach is more modular, extensible, and testable than using helpers alone.
Conclusion
Inline Partials and Decorators significantly enhance Handlebars, improving code organization, reusability, and testability. They are valuable tools for building more maintainable and efficient front-end applications.
The above is the detailed content of Using Inline Partials and Decorators with Handlebars 4.0. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
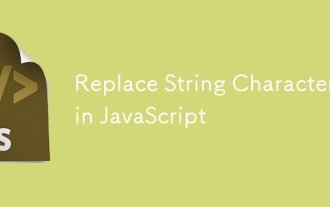
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
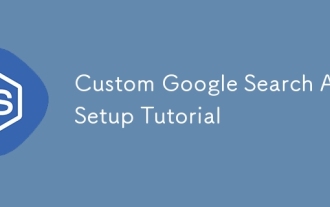
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
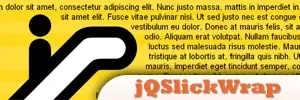
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
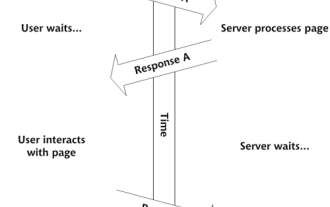
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
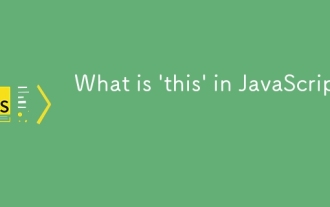
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
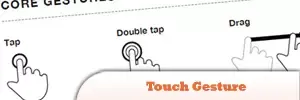
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
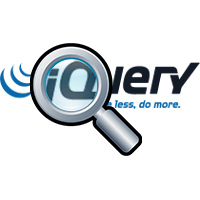
jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
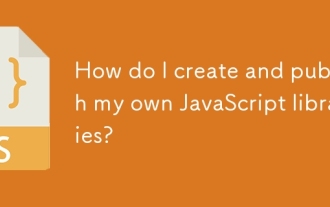
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
