How to Effectively Manage Configuration in Go Applications?
How to Handle Configuration in Go
When developing Go programs, it's common to need a mechanism to manage configuration parameters. Typically, in other contexts, one might turn to properties or INI files for this purpose. In Go, several approaches can be employed.
Recommended Approach: JSON
One preferred approach is to use the JSON format for configuration. Go's standard library provides methods for conveniently writing data structures as indented JSON, making them easy to read and edit. Additionally, JSON offers semantics for lists and mappings, which is not available in all INI-type config parsers.
Here's an example of how to use JSON for configuration:
conf.json:
{ "Users": ["UserA","UserB"], "Groups": ["GroupA"] }
Go program to read the configuration:
import ( "encoding/json" "os" "fmt" ) type Configuration struct { Users []string Groups []string } file, _ := os.Open("conf.json") defer file.Close() decoder := json.NewDecoder(file) configuration := Configuration{} err := decoder.Decode(&configuration) if err != nil { fmt.Println("error:", err) } fmt.Println(configuration.Users) // output: [UserA, UserB]
The above is the detailed content of How to Effectively Manage Configuration in Go Applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undress AI Tool
Undress images for free

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)
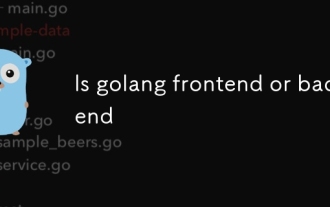
Golang is mainly used for back-end development, but it can also play an indirect role in the front-end field. Its design goals focus on high-performance, concurrent processing and system-level programming, and are suitable for building back-end applications such as API servers, microservices, distributed systems, database operations and CLI tools. Although Golang is not the mainstream language for web front-end, it can be compiled into JavaScript through GopherJS, run on WebAssembly through TinyGo, or generate HTML pages with a template engine to participate in front-end development. However, modern front-end development still needs to rely on JavaScript/TypeScript and its ecosystem. Therefore, Golang is more suitable for the technology stack selection with high-performance backend as the core.
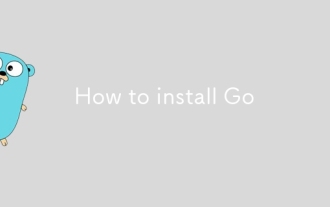
The key to installing Go is to select the correct version, configure environment variables, and verify the installation. 1. Go to the official website to download the installation package of the corresponding system. Windows uses .msi files, macOS uses .pkg files, Linux uses .tar.gz files and unzip them to /usr/local directory; 2. Configure environment variables, edit ~/.bashrc or ~/.zshrc in Linux/macOS to add PATH and GOPATH, and Windows set PATH to Go in the system properties; 3. Use the government command to verify the installation, and run the test program hello.go to confirm that the compilation and execution are normal. PATH settings and loops throughout the process
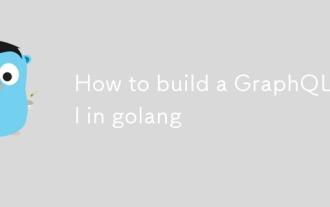
To build a GraphQLAPI in Go, it is recommended to use the gqlgen library to improve development efficiency. 1. First select the appropriate library, such as gqlgen, which supports automatic code generation based on schema; 2. Then define GraphQLschema, describe the API structure and query portal, such as defining Post types and query methods; 3. Then initialize the project and generate basic code to implement business logic in resolver; 4. Finally, connect GraphQLhandler to HTTPserver and test the API through the built-in Playground. Notes include field naming specifications, error handling, performance optimization and security settings to ensure project maintenance
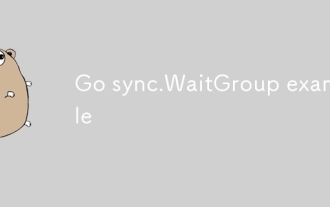
sync.WaitGroup is used to wait for a group of goroutines to complete the task. Its core is to work together through three methods: Add, Done, and Wait. 1.Add(n) Set the number of goroutines to wait; 2.Done() is called at the end of each goroutine, and the count is reduced by one; 3.Wait() blocks the main coroutine until all tasks are completed. When using it, please note: Add should be called outside the goroutine, avoid duplicate Wait, and be sure to ensure that Don is called. It is recommended to use it with defer. It is common in concurrent crawling of web pages, batch data processing and other scenarios, and can effectively control the concurrency process.
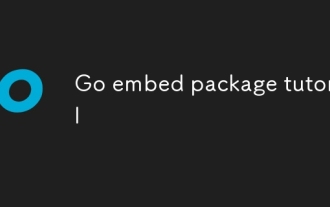
Using Go's embed package can easily embed static resources into binary, suitable for web services to package HTML, CSS, pictures and other files. 1. Declare the embedded resource to add //go:embed comment before the variable, such as embedding a single file hello.txt; 2. It can be embedded in the entire directory such as static/*, and realize multi-file packaging through embed.FS; 3. It is recommended to switch the disk loading mode through buildtag or environment variables to improve efficiency; 4. Pay attention to path accuracy, file size limitations and read-only characteristics of embedded resources. Rational use of embed can simplify deployment and optimize project structure.
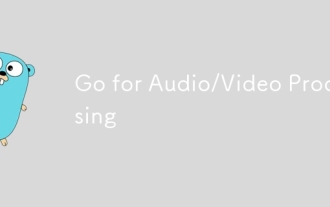
The core of audio and video processing lies in understanding the basic process and optimization methods. 1. The basic process includes acquisition, encoding, transmission, decoding and playback, and each link has technical difficulties; 2. Common problems such as audio and video aberration, lag delay, sound noise, blurred picture, etc. can be solved through synchronous adjustment, coding optimization, noise reduction module, parameter adjustment, etc.; 3. It is recommended to use FFmpeg, OpenCV, WebRTC, GStreamer and other tools to achieve functions; 4. In terms of performance management, we should pay attention to hardware acceleration, reasonable setting of resolution frame rates, control concurrency and memory leakage problems. Mastering these key points will help improve development efficiency and user experience.
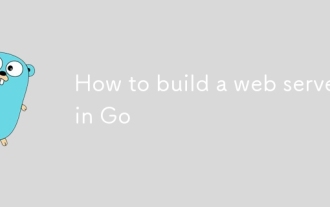
It is not difficult to build a web server written in Go. The core lies in using the net/http package to implement basic services. 1. Use net/http to start the simplest server: register processing functions and listen to ports through a few lines of code; 2. Routing management: Use ServeMux to organize multiple interface paths for easy structured management; 3. Common practices: group routing by functional modules, and use third-party libraries to support complex matching; 4. Static file service: provide HTML, CSS and JS files through http.FileServer; 5. Performance and security: enable HTTPS, limit the size of the request body, and set timeout to improve security and performance. After mastering these key points, it will be easier to expand functionality.
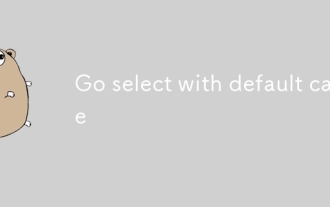
The purpose of select plus default is to allow select to perform default behavior when no other branches are ready to avoid program blocking. 1. When receiving data from the channel without blocking, if the channel is empty, it will directly enter the default branch; 2. In combination with time. After or ticker, try to send data regularly. If the channel is full, it will not block and skip; 3. Prevent deadlocks, avoid program stuck when uncertain whether the channel is closed; when using it, please note that the default branch will be executed immediately and cannot be abused, and default and case are mutually exclusive and will not be executed at the same time.
