Is Go's `range` Keyword Safe for Concurrent Map Access?
Concurrent Access to Maps with 'range' in Go
In the "Go maps in action" blog entry, the documentation states that maps are not safe for concurrent use without synchronization mechanisms like sync.RWMutex. However, it's not clear whether access via range iteration constitutes a "read" or if the range keyword has specific locking requirements.
Concurrency of range
The range expression is evaluated once before the loop begins. For maps, this means that the map variable (e.g., testMap) is only evaluated once, even though new key-value pairs may be added or removed during the iteration. This separation between the initial evaluation and the iteration itself implies that the map is not accessed by the for statement while executing an iteration.
Safe Iteration
Therefore, the following iteration is safe for concurrent access:
func IterateMapKeys(iteratorChannel chan int) error { testMapLock.RLock() defer testMapLock.RUnlock() mySeq := testMapSequence for k, _ := range testMap { .... } return nil }
This design ensures that the map is locked only when necessary, while allowing for concurrent access to other goroutines.
Concurrent Modification
However, this type of locking only prevents concurrent access, not concurrent modification. It's possible for another goroutine to acquire the write lock and modify the map even while the iteration is ongoing. To prevent this, the map should remain locked throughout the iteration.
Example
This example demonstrates the difference between unlocking and locking within the for block:
func IterateMapKeys(iteratorChannel chan int) error { testMapLock.RLock() defer testMapLock.RUnlock() mySeq := testMapSequence for k, _ := range testMap { testMapLock.RUnlock() .... testMapLock.RLock() .... } return nil }
In this example, releasing the read lock within the for block allows for concurrent modification and potential errors.
Conclusion
The range keyword itself does not provide synchronization for concurrent access to maps. Using proper synchronization mechanisms (e.g., sync.RWMutex) is crucial to ensure the safety of concurrent iterations and modifications.
The above is the detailed content of Is Go's `range` Keyword Safe for Concurrent Map Access?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undress AI Tool
Undress images for free

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)
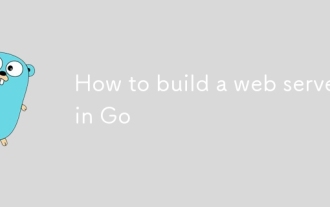
It is not difficult to build a web server written in Go. The core lies in using the net/http package to implement basic services. 1. Use net/http to start the simplest server: register processing functions and listen to ports through a few lines of code; 2. Routing management: Use ServeMux to organize multiple interface paths for easy structured management; 3. Common practices: group routing by functional modules, and use third-party libraries to support complex matching; 4. Static file service: provide HTML, CSS and JS files through http.FileServer; 5. Performance and security: enable HTTPS, limit the size of the request body, and set timeout to improve security and performance. After mastering these key points, it will be easier to expand functionality.
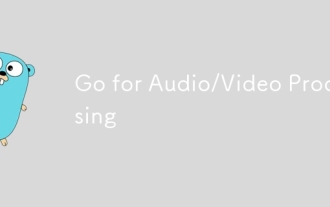
The core of audio and video processing lies in understanding the basic process and optimization methods. 1. The basic process includes acquisition, encoding, transmission, decoding and playback, and each link has technical difficulties; 2. Common problems such as audio and video aberration, lag delay, sound noise, blurred picture, etc. can be solved through synchronous adjustment, coding optimization, noise reduction module, parameter adjustment, etc.; 3. It is recommended to use FFmpeg, OpenCV, WebRTC, GStreamer and other tools to achieve functions; 4. In terms of performance management, we should pay attention to hardware acceleration, reasonable setting of resolution frame rates, control concurrency and memory leakage problems. Mastering these key points will help improve development efficiency and user experience.
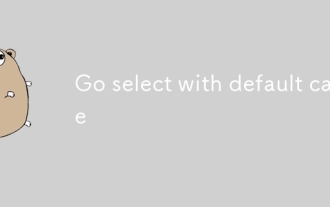
The purpose of select plus default is to allow select to perform default behavior when no other branches are ready to avoid program blocking. 1. When receiving data from the channel without blocking, if the channel is empty, it will directly enter the default branch; 2. In combination with time. After or ticker, try to send data regularly. If the channel is full, it will not block and skip; 3. Prevent deadlocks, avoid program stuck when uncertain whether the channel is closed; when using it, please note that the default branch will be executed immediately and cannot be abused, and default and case are mutually exclusive and will not be executed at the same time.
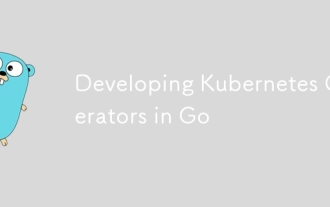
The most efficient way to write a KubernetesOperator is to use Go to combine Kubebuilder and controller-runtime. 1. Understand the Operator pattern: define custom resources through CRD, write a controller to listen for resource changes and perform reconciliation loops to maintain the expected state. 2. Use Kubebuilder to initialize the project and create APIs to automatically generate CRDs, controllers and configuration files. 3. Define the Spec and Status structure of CRD in api/v1/myapp_types.go, and run makemanifests to generate CRDYAML. 4. Reconcil in the controller
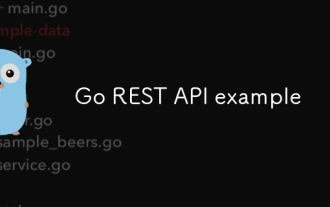
How to quickly implement a RESTAPI example written in Go? The answer is to use the net/http standard library, which can be completed in accordance with the following three steps: 1. Set up the project structure and initialize the module; 2. Define the data structure and processing functions, including obtaining all data, obtaining single data based on the ID, and creating new data; 3. Register the route in the main function and start the server. The entire process does not require a third-party library. The basic RESTAPI function can be realized through the standard library and can be tested through the browser or Postman.
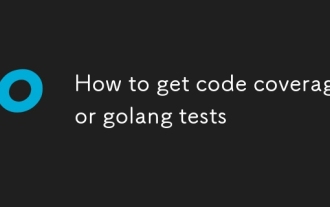
Use the getest built-in command to generate coverage data: run getest-cover./... to display the coverage percentage of each package, or use getest-coverprofile=coverage.out./... to generate a detailed report and view the specific uncovered lines of code through gotoolcover-html=coverage.out-ocoverage.html. Integrate coverage report in CI: create a coverage.out file, and upload analysis through third-party tools such as codecov or coveralls, for example, using curl--data-binary@coverage.o
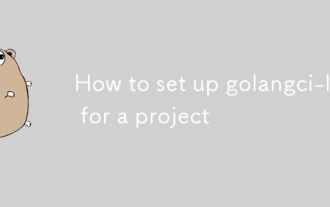
The installation steps of golangci-lint are: 1. Install using binary installation or Goinstall command; 2. Verify whether the installation is successful; the configuration methods include: 3. Create a .golangci.yml file to enable/disable linters, set an exclusion path, etc.; the integration methods are: 4. Add lint steps in CI/CD (such as GitHubActions) to ensure that lint checks are automatically run for each submission and PR.
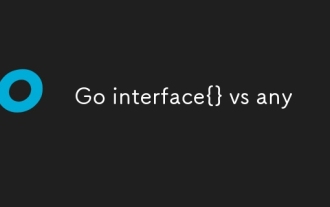
In Go language, interface{} and any are exactly the same type. Since Go1.18, any has been introduced as an alias for interface{}. The main purpose is to improve the readability and semantic clarity of the code; 1. Any is more suitable for scenarios that express "arbitrary types", such as function parameters, map/slice element types, general logic implementations, etc.; 2. Interface{} is more suitable for defining interface behavior, emphasizing interface types, or compatible with old code; 3. The performance of the two is exactly the same as the underlying mechanism, and the compiler will replace any with interface{}, which will not cause additional overhead; 4. Pay attention to type safety issues when using it, and may need to cooperate with type assertions or
