


How Can I Count Substring Occurrences in JavaScript Using Regular Expressions?
Counting String Occurrences in Strings
Counting the frequency of a particular substring within a larger string can be a common task in programming. In JavaScript, there are several approaches to achieve this.
One method utilizes regular expressions. Regular expressions provide a powerful way to search and match patterns in strings. To count occurrences of a substring, we can use the g (global) flag in a regular expression to match all instances of the substring.
For instance, consider the following JavaScript code:
var temp = "This is a string."; var count = (temp.match(/is/g) || []).length; console.log(count);
In this example, we have a string temp containing the text "This is a string." We use the match method with the regular expression /is/g to search for all instances of the substring "is" within temp. The g flag ensures that all matches are captured.
The result of the match method is an array of matching substrings. However, if no matches are found, the match method returns null. To handle this case, we use the logical OR (||) operator to check if the match result is null and return an empty array [] instead.
Finally, we use the length property of the match result (or the empty array if there were no matches) to determine the count of occurrences of the substring in the string. In this case, console.log(count) will output '2', as there are two occurrences of the substring "is" in the given string.
The above is the detailed content of How Can I Count Substring Occurrences in JavaScript Using Regular Expressions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
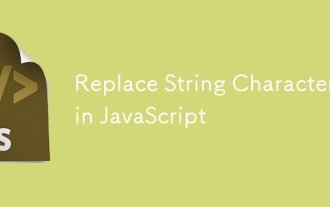
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
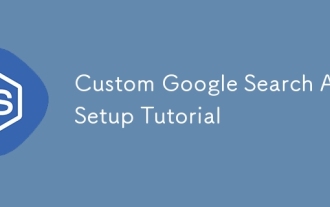
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
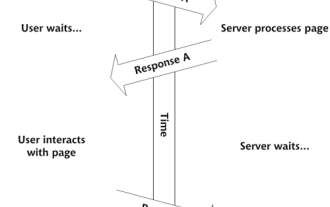
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
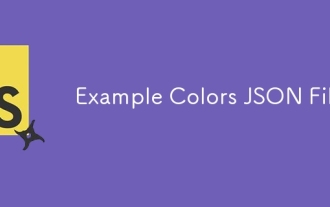
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
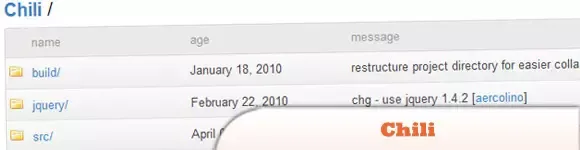
Enhance Your Code Presentation: 10 Syntax Highlighters for Developers Sharing code snippets on your website or blog is a common practice for developers. Choosing the right syntax highlighter can significantly improve readability and visual appeal. T
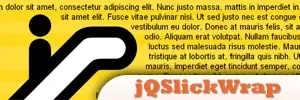
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
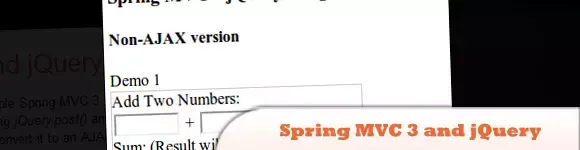
This article presents a curated selection of over 10 tutorials on JavaScript and jQuery Model-View-Controller (MVC) frameworks, perfect for boosting your web development skills in the new year. These tutorials cover a range of topics, from foundatio
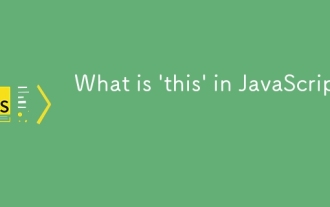
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was
